Closed DailyStruggle closed 3 years ago
I like what ya did here, and I love the explanation of the change. thank you for your contribution! I will do some tests before the next update, but this will be very much appreciated for those looking for a more flat randomness across the "donut" random generation.
also, I built a basic logging/learning algorithm on top of this design for performance improvements and for optional unique placements and automatic region expansion.
I'm not sure how to work it in within this repo, but it's an interesting bonus feature of the design.
The circle calculation trended towards the outer edge where min>0. A new donut calculation flattens the spatial distribution.
The new calculation follows a 2D spiral that turns once per integer step, with a length equal to the area within the donut.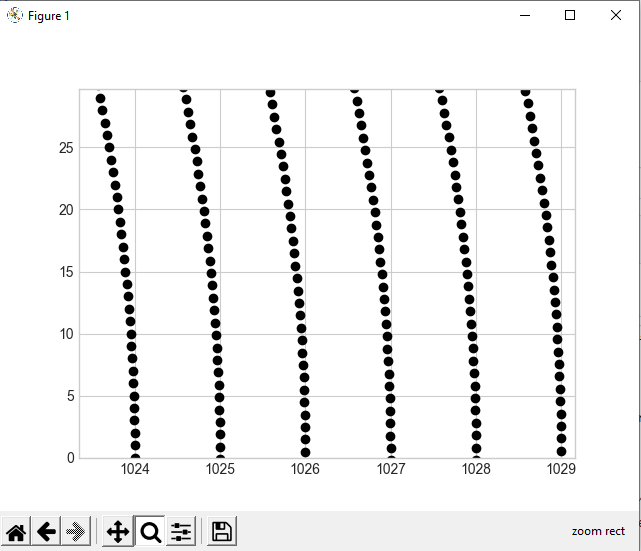
using maxrad = 4096 min = 0
it matches the old distribution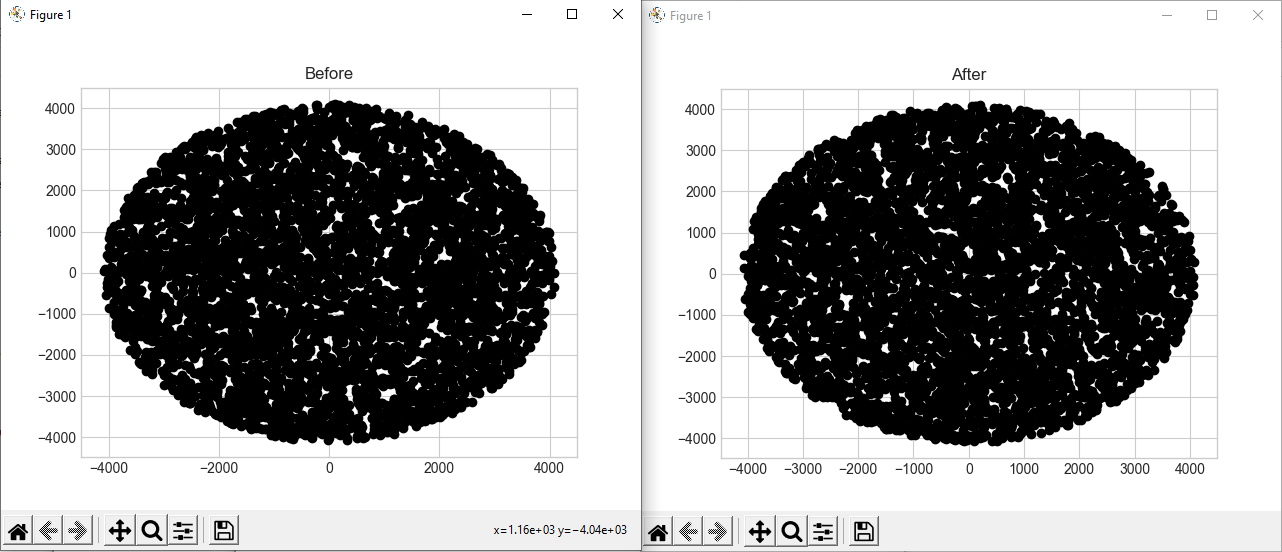
using maxRad = 4096 min = 1024
there's an improvement around the donut hole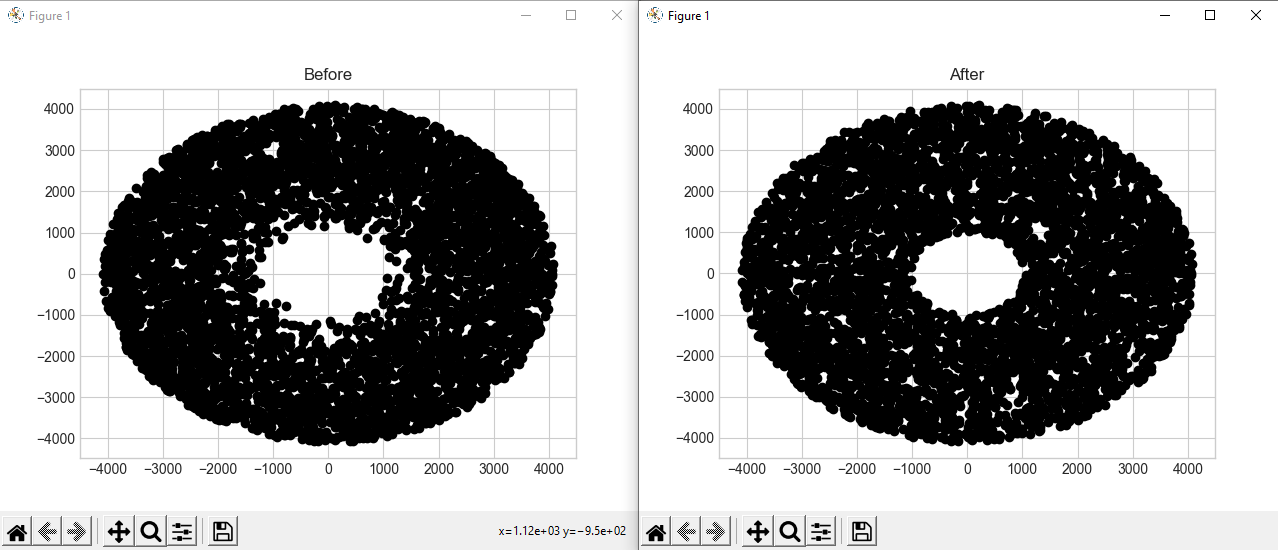
python scripts used: before
after