Closed ctaggart closed 6 years ago
I can give you our map bindings if you like and you can compare.
Scratch that. Our map is for react proper. But I assume we need to look at the other plugins that I wrote to see how these manual bindings differ. One thing I that I didn't saw before is the ofType instantiation. I don't think we do it like that
ofType looks to wrap createElement
. Yes, I'd be curious how you do it. This is based on this example https://github.com/mapbox/react-native-mapbox-gl/blob/master/example/src/components/ShowMap.js
https://github.com/fable-compiler/fable-react-native/blob/master/src/extra/react-native-signature-view/Fable.Helpers.ReactNativeSignatureView.fs is the simplest thing I know. There are more samples in that folder.
Also you always have to do the manual linking steps that are explained on the package readme
Thanks, that gives me some ideas. Will try them in the morning.
It is working for me now. 😅
The main changes were:
default
let [<Import("default","@mapbox/react-native-mapbox-gl")>] mapbox: Mapbox.IExports = jsNative
MapView
inherits from ComponentClass
type MapView =
inherit ComponentClass<MapViewPropTypes>
abstract setDirection: direction: float * ?animated: bool * ?callback: (unit -> unit) -> Promise<unit>
//abstract MapView: MapViewStatic
abstract MapView: MapView
The MapView.style is set to flex
:
Looks kind of ugly, but it works for now:
type StyleProps =
abstract flex: float option with get, set
let style = Fable.Import.ReactNative.Globals.StyleSheet.create(jsOptions<StyleProps>(fun o -> o.flex <- Some 1.)) :> obj :?> ViewStyle
Cool. So this means you are doing elmish react native? Would be very cool.
Cameron Taggart notifications@github.com schrieb am Di., 27. März 2018, 23:45:
It is working for me now. 😅
The main changes were:
- import default
let [Import("default","@mapbox/react-native-mapbox-gl")] mapbox: Mapbox.IExports = jsNative
MapView inherits from ComponentClass
type MapView = inherit ComponentClass
abstract setDirection: direction: float * ?animated: bool * ?callback: (unit -> unit) -> Promise<unit>
reference MapView not the static wrapper
//abstract MapView: MapViewStatic abstract MapView: MapView
The MapView.style is set to flex: Looks kind of ugly, but it works for now:
type StyleProps = abstract flex: float option with get, set
let style = Fable.Import.ReactNative.Globals.StyleSheet.create(jsOptions<StyleProps>(fun o -> o.flex <- Some 1.)) :> obj :?> ViewStyle
— You are receiving this because you were mentioned. Reply to this email directly, view it on GitHub https://github.com/SAFE-Stack/SAFE-Nightwatch/issues/57#issuecomment-376686285, or mute the thread https://github.com/notifications/unsubscribe-auth/AADgNCwJ4kTM74YMbP7PPPawpW7cUMLWks5tirMCgaJpZM4S8RVy .
Cool. So this means you are doing elmish react native? Would be very cool.
I am. I have a short contract to make LaneSpotter work on Android.
I've tried extending this to use Mapbox on a new page as part of my tech evaluation. If I can get it to work (very soon), I'll use Fable, if not I have to use TypeScript.
https://www.mapbox.com/help/first-steps-react-native-sdk/
The problem I'm stuck on leads to this error: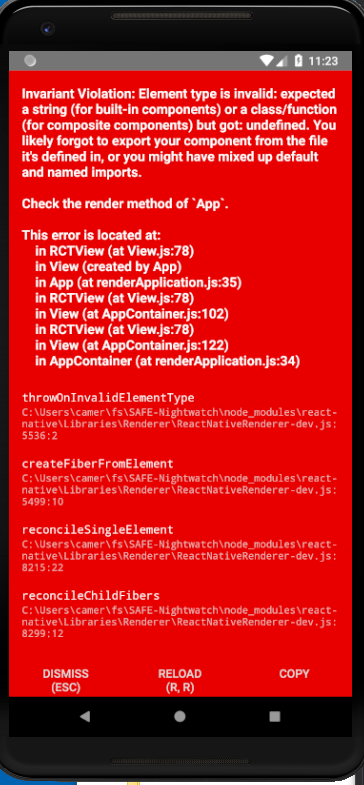
cc @forki @alfonsogarciacaro