Open nurhani208 opened 6 years ago
Don't really know how to do. Anyone know please help to explain.
import java.util.Scanner;
class Node { protected int data; protected Node link;
public Node()
{
link = null;
data = 0;
}
public Node(int d,Node n)
{
data = d;
link = n;
}
public void setLink(Node n)
{
link = n;
}
public void setData(int d)
{
data = d;
}
public Node getLink()
{
return link;
}
public int getData()
{
return data;
}
}
class linkedList { protected Node start; protected Node end ; public int size ;
public linkedList()
{
start = null;
end = null;
size = 0;
}
public boolean isEmpty()
{
return start == null;
}
public int getSize()
{
return size;
}
public void insertAtStart(int val)
{
Node nptr = new Node(val, null);
size++ ;
if(start == null)
{
start = nptr;
end = start;
}
else
{
nptr.setLink(start);
start = nptr;
}
}
public void insertAtEnd(int val)
{
Node nptr = new Node(val,null);
size++ ;
if(start == null)
{
start = nptr;
end = start;
}
else
{
end.setLink(nptr);
end = nptr;
}
}
public void insertAtPos(int val , int pos)
{
Node nptr = new Node(val, null);
Node ptr = start;
pos = pos - 1 ;
for (int i = 1; i < size; i++)
{
if (i == pos)
{
Node tmp = ptr.getLink() ;
ptr.setLink(nptr);
nptr.setLink(tmp);
break;
}
ptr = ptr.getLink();
}
size++ ;
}
public void deleteAtPos(int pos)
{
if (pos == 1)
{
start = start.getLink();
size--;
return ;
}
if (pos == size)
{
Node s = start;
Node t = start;
while (s != end)
{
t = s;
s = s.getLink();
}
end = t;
end.setLink(null);
size --;
return;
}
Node ptr = start;
pos = pos - 1 ;
for (int i = 1; i < size - 1; i++)
{
if (i == pos)
{
Node tmp = ptr.getLink();
tmp = tmp.getLink();
ptr.setLink(tmp);
break;
}
ptr = ptr.getLink();
}
size-- ;
}
public void display()
{
System.out.print("\nSingly Linked List = ");
if (size == 0)
{
System.out.print("empty\n");
return;
}
if (start.getLink() == null)
{
System.out.println(start.getData() );
return;
}
Node ptr = start;
System.out.print(start.getData()+ "->");
ptr = start.getLink();
while (ptr.getLink() != null)
{
System.out.print(ptr.getData()+ "->");
ptr = ptr.getLink();
}
System.out.print(ptr.getData()+ "\n");
}
}
public class SinglyLinkedList
{
public static void main(String[] args)
{
Scanner scan = new Scanner(System.in);
linkedList list = new linkedList();
System.out.println("List Test");
char ch;
do
{
System.out.println("\n List Operations\n");
System.out.println("1. insert at begining");
System.out.println("2. insert at end");
System.out.println("3. insert at position");
System.out.println("4. delete at position");
System.out.println("5. check empty");
System.out.println("6. get size");
int choice = scan.nextInt();
switch (choice)
{
case 1 :
System.out.println("Enter integer element to insert");
list.insertAtStart( scan.nextInt() );
break;
case 2 :
System.out.println("Enter integer element to insert");
list.insertAtEnd( scan.nextInt() );
break;
case 3 :
System.out.println("Enter integer element to insert");
int num = scan.nextInt() ;
System.out.println("Enter position");
int pos = scan.nextInt() ;
if (pos <= 1 || pos > list.getSize() )
System.out.println("Invalid position\n");
else
list.insertAtPos(num, pos);
break;
case 4 :
System.out.println("Enter position");
int p = scan.nextInt() ;
if (p < 1 || p > list.getSize() )
System.out.println("Invalid position\n");
else
list.deleteAtPos(p);
break;
case 5 :
System.out.println("Empty status = "+ list.isEmpty());
break;
case 6 :
System.out.println("Size = "+ list.getSize() +" \n");
break;
default :
System.out.println("Wrong Entry \n ");
break;
}
list.display();
System.out.println("\nDo you want to continue (Type y or n) \n");
ch = scan.next().charAt(0);
} while (ch == 'Y'|| ch == 'y');
}
}
we suppose to use Contiguous List not linked list,right?
Lee Pui Kuan 252990
import java.util.*;
public class Question1 {
public static void main(String[] args) {
// Creae a list and add some colors to the list
List<String> list_Strings = new ArrayList<String>();
list_Strings.add("red");
list_Strings.add("green");
list_Strings.add("orange");
list_Strings.add("white");
list_Strings.add("black");
list_Strings.add("yellow");
list_Strings.add("purple");
list_Strings.add("blue");
list_Strings.add("grey");
list_Strings.add("brown");
// Print the list
System.out.println(list_Strings);
// Now insert a color at the first position of the list
list_Strings.add(0, "pink");
// Print the list
System.out.println(list_Strings);
// Update the forth element with "light blue"
list_Strings.set(3, "light blue");
// Print the list again
System.out.println(list_Strings);
// Remove the third element from the list.
list_Strings.remove(2);
// Print the list again
System.out.println("After removing third element from the list:\n"+list_Strings);
// Search an element
if (list_Strings.contains("pink")) {
System.out.println("Found the element");
} else {
System.out.println("There is no such element");
}
}
}
```java
253123 Wong Kin Sin
import java.util.Scanner;
public class Exe5Contiguous {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
// Task i
String colorList [] = new String [10];
colorList[1]="Red";
colorList[3]="Blue";
colorList[9]="Green";
// Task ii
System.out.print("Color for first position: ");
colorList[0]=scan.nextLine();
System.out.println("\nCurrent color in the list: ");
for(int i = 0; i<colorList.length;i++){
System.out.println(i+". "+colorList[i]);
}
// Task iii
System.out.print("\nPlease update color in element(4): ");
colorList[3]=scan.nextLine();
// Task iv
colorList[2]=null;
System.out.println("\nRemoved third element in the list.");
System.out.println("Current color in the list: ");
for(int i = 0; i<colorList.length;i++){
System.out.println(colorList[i]);
}
// Task v
System.out.print("\nSelect 1 color from the list: ");
String search = scan.nextLine();
for(int k = 0; k<colorList.length;k++){
if(search.equals(colorList[k])){
System.out.println("Found the element");
System.out.println("Index of the element: "+ k);
}
}
}
}
```java
Output:
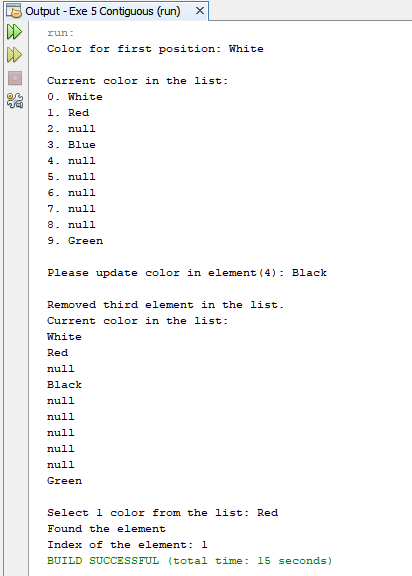
So Wai Jing 253234 '''import java.util.Scanner; public class Contiguous_List { public static void main(String[] args) { String []element = new String[10]; int i=0; Scanner scan = new Scanner(System.in);
System.out.printf("Enter the number of color wanted to input : ");
int num = scan.nextInt();
for(i =0;i<num;i++){
System.out.printf("Enter the color %d : ",i+1);
element[i]=scan.next();
}
System.out.printf("\nEnter the First element : ");
for(i=num;i>=0;i--){
element[i+1]=element[i];
}
element[0]=scan.next();
num=num+1;
System.out.println("\nElement\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
System.out.printf("\nEnter the new color for element 5 : ");
element[4]=scan.next();
System.out.println("Element\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
int count = num;
for(i=num-1;i>=0;i--){
if((count-i)>=2){
element[count-i]=element[(count-i)+1];
}
}
num=num-1;
System.out.println("\nElement\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
System.out.println("\nThe third element is removed.");
System.out.printf("\nEnter the element wanted to search : ");
String search = scan.next();
for(i=0;i<num;i++){
if(search.equals(element[i])){
System.out.println("Found the element!");
System.out.printf("The index of the element is %d.\n", i);
}
}
}
}'''java
`package data_structure; import java.util.*;
public class Data_Structure {
public static void main(String[] args) {
ArrayList<String> list_Strings = new ArrayList<String>();
list_Strings.add("purple"); list_Strings.add("Turqouise"); list_Strings.add("red"); list_Strings.add("White"); list_Strings.add("Black"); list_Strings.add("Blue"); list_Strings.add("Brown"); list_Strings.add("Orange"); list_Strings.add("Cream"); list_Strings.add("Red"); // Print the list System.out.println(list_Strings); // Now insert a color at the first and last position of the list list_Strings.add(0, "Pink");
// Print the list System.out.println(list_Strings); // Update the forth element with "Yellow" list_Strings.set(3, "yellow"); // Print the list again System.out.println(list_Strings); // Remove the third element from the list. list_Strings.remove(2); // Print the list again System.out.println("After removing third element from the list:\n"+list_Strings); // Search an element if (list_Strings.contains("pink")) { System.out.println("Found the element"); } else { System.out.println("There is no such element"); } } } `
253920 Amiera Syazlin Binti Md Azhar `public static void main(String[] args) {
String [] colors = new String [10] ;
int i = 0 ;
Scanner scan = new Scanner (System.in) ;
System.out.printf("Enter number of colors : ");
int num = scan.nextInt();
for(i =0;i<num;i++)
{
System.out.printf("Enter Color %d : ",i+1);
colors[i] = scan.next();
}
System.out.printf("\nEnter First Color : ");
for(i=num; i >= 0; i--)
{
colors[i + 1]=colors[i];
}
colors[0]=scan.next();
num = num + 1;
System.out.println("Color");
for(i=0; i < num; i++)
{
System.out.printf(" %-15d \t%s\n",i+1,colors[i]);
}
System.out.printf("\nEnter new color for color 5 : ");
colors[4]=scan.next();
System.out.println("Color");
for(i=0;i<num;i++)
{
System.out.printf(" %-15d \t%s\n",i+1,colors[i]);
}
int count = num;
for(i = num-1; i >= 0; i--)
{
if((count-i) >= 2)
{
colors[count-i] = colors[(count-i)+1];
}
}
num=num-1;
System.out.println("\n Color");
for(i=0; i < num; i++){
System.out.printf(" %-15d \t%s\n",i+1,colors[i]);
}
System.out.println("\nThird Color is Removed.");
System.out.printf("\nEnter color to search : ");
String search = scan.next();
for(i=0; i<num; i++){
if(search.equals(colors[i]))
{
System.out.println("Found the color!");
System.out.printf("The index of the color is %d.\n", i);
}
}
}
}`
Output ; run: Enter number of colors : 4 Enter Color 1 : pink Enter Color 2 : blue Enter Color 3 : green Enter Color 4 : purple
Enter First Color : brown Color 1 brown 2 pink 3 blue 4 green 5 purple
Enter new color for color 5 : black Color 1 brown 2 pink 3 blue 4 green 5 black
Color 1 brown 2 pink 3 green 4 black
Third Color is Removed.
Enter color to search : pink Found the color! The index of the color is 1. BUILD SUCCESSFUL (total time: 24 seconds)
254318 noradilla binti aidi amin ``java import java.util.Scanner;
/*
@author user */ public class NewArray {
/**
@param args the command line arguments */ public static void main(String[] args) { // TODO code application logic here Scanner scan = new Scanner(System.in); String[] StringColour= new String[]{"pink","green","white","yellow","orange","purple","turqoise","blue","black","red"};
List
StringList.add("black");
StringList.add("red");
System.out.println("List :"+ StringList);
//update the element System.out.print("Enter the colour list that you to update:"); String searchList = scan.nextLine();
int arrayLength = 0; for (int i =0; i< arrayLength; i++) { if (StringColour[i].equals(searchList)) { System.out.println("You want to update "+ searchList+",it is in index "+i); System.out.print("Enter the new colour that you want replace with"+searchList +":"); String updateColour = scan.nextLine(); StringColour[i] = updateColour; int flag = 1; break; } } int flag = 0; if (flag == 0) System.out.println("The colour does not exist"); } //remove the element System.out.println("StringColour"); StringColour.remove(2);
System.out.println("After remove the third element from the list:"+StringColour); // Search an element if (StringColour.contains("light blue")){ System.out.println("Found the element"); } else{ System.out.println("There is no such element"); }
} ``java
muhammad sufyan bin ahmad kamal 255541
import java.util.*; public class colour {
public static void main(String[] args) {
List<String> list_Strings = new ArrayList<String>();
list_Strings.add("blue"); list_Strings.add("brown"); list_Strings.add("black"); list_Strings.add("white"); list_Strings.add("yellow"); list_Strings.add("orange"); list_Strings.add("purple"); list_Strings.add("red"); list_Strings.add("grey"); list_Strings.add("pink"); System.out.println(list_Strings);
list_Strings.add(0, "grey"); System.out.println(list_Strings);
list_Strings.set(3, "gold"); System.out.println(list_Strings);
list_Strings.remove(2); System.out.println("latest list:\n"+list_Strings);
if (list_Strings.contains("yellow")) { System.out.println("Found the element"); } else { System.out.println("There is no such element");
}
}
}
Nuraisya binti Kamaruzzaman (255730) '''java package contiguouslist;
import java.util.Scanner;
public class ContiguousList {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String [] colour = new String[10];
System.out.print("Enter number of colour :> ");
int num = scan.nextInt();
for(int i=0; i<num; i++){
System.out.print("Colour "+(i+1) +" :> ");
colour[i] = scan.next();
}
System.out.println("Element\t\t\tColor");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t\t "+colour[i]);
}
System.out.print("Enter the first element :> ");
for (int i=num; i>=0; i--){
colour[i+1]=colour[i];
}
colour[0] = scan.next();
num=num+1;
System.out.println("Element\t\t\tColor");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t\t "+colour[i]);
}
System.out.print("Enter the new color for element 4 :> ");
colour[3]=scan.next();
System.out.println("Element\t\t\tColor");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t\t "+colour[i]);
}
int j = num;
for(int i=num-1;i>=0;i--){
if((j-i)>=2){
colour[j-i]=colour[(j-i)+1];
}
}
num=num-1;
System.out.println("Element\t\t\tColor");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t\t "+colour[i]);
}
System.out.println("The third element is removed.");
System.out.print("Enter the element wanted to search :> ");
String search = scan.next();
for(int i=0;i<num;i++){
if(search.equals(colour[i])){
System.out.println("Found the element!");
System.out.println("The index of the element is " +i);
}
}
}
} '''java
Ling Zhong Li 252931
*/import java.util.Scanner;
public class Colors {
/**
* @param args the command line arguments
*/static Scanner scan=new Scanner(System.in);
public static void main(String[] args) {
// TODO code application logic here
String[]color=new String[10];
int size=4;
String newColor;
//input the color
System.out.println("Enter 4 type of color: ");
for(int i=0;i<size;i++)
{
color[i]=scan.next();
}
System.out.println("");
//Display color
System.out.println("Display color in the list");
for(int i=0;i<size;i++)
{
System.out.println(color[i]);
}
System.out.println("");
System.out.print("Add new color in the first index: ");
newColor=scan.next();
System.out.println("");
addColor(color,size,newColor);
System.out.println("");
String changeColor=color[3];
editColor(color,changeColor,size);
String target=color[2];
removeColor(color,target,size);
System.out.println("");
System.out.print("Enter search color: ");
String searchColor=scan.next();
for(int i=0;i<size;i++)
{
if(color[i].equals(searchColor))
{
System.out.println("");
System.out.println("The color is found");
System.out.println("The color "+color[i]+" is at index "+i);
}
else
{
System.out.println("The color is not in the list");
}
}
}
//add new color in the first index
public static void addColor(String []color,int size,String newColor)
{
if(size==0)
{
color[0]=newColor;
size++;
}
else if(size==10)
{
System.out.println("The list is full");
}
else
{
int index=0;
for(int x=size;x>index;x--)
{
color[x]=color[x-1];
}
color[index]=newColor;
size++;
}
System.out.println("Display color in the list after change the new color ");
for(int i=0;i<size;i++)
{
System.out.println(color[i]);
}
}
public static void editColor(String []color,String changeColor,int size)
{
boolean edit=false;
for(int i=0;i<size;i++)
if(color[i].equals(changeColor))
{
System.out.print("Enter new color:");
color[i]=scan.next();
edit=true;
break;
}
if(edit==false){
System.out.println("The color is not in the list ");}
System.out.println("");
System.out.println("Display color in the list after element 4 change to new color");
for(int i=0;i<5;i++)
{
System.out.println(color[i]);
}
}
public static void removeColor(String []color,String target,int size)
{
int i,j;
boolean delete=false;
for(i=0;i<size;i++){
if(color[i].equals(target))
{
for(j=i;j<5-1;j++)
color[j]=color[j+1];
delete=true;
break;
}
}
if(delete==false)
{
System.out.println("The color is not in the list");
}
System.out.println("");
System.out.println("Display color in the list after delate the third element in list color");
for( i=0;i<size;i++)
{
System.out.println(color[i]);
}
}
}
java```
Shum Chen Yau 254854
package list; import java.util.ArrayList; import java.util.*; /**
@author YAU */ public class List { /**
@param args the command line arguments
*/
public static void main(String[] args) {
Scanner sc= new Scanner (System.in);
ArrayList
//insert an element list.add(0, "jade green"); System.out.println("new list : " + list);
//update the 4th color into aqua list.set(3, "aqua"); System.out.println("After changing : "+list);
//remove third color list.remove(2); System.out.println("After removing : "+ list);
//searching element System.out.println("Please enter a color : "); String color = sc.nextLine(); if (list.contains(color)) { System.out.println("Found the element!"); System.out.println("The index of "+ color +" is "+ list.indexOf(color)); } else { System.out.println("Error input"); } }
Output:
[red, yellow, orange, green, blue, purple, pink, black, white, grey] new list : [jade green, red, yellow, orange, green, blue, purple, pink, black, white, grey] After changing : [jade green, red, yellow, aqua, green, blue, purple, pink, black, white, grey] After removing : [jade green, red, aqua, green, blue, purple, pink, black, white, grey]
Please enter a color : purple Found the element! The index of purple is 5 BUILD SUCCESSFUL (total time: 5 seconds)
baktajivan pillay a/l kanegekumar 252709 '''java import java.util.Scanner;
public class Color { public static void main(String [] args){ Scanner read = new Scanner(System.in); String [] colorList = new String [10];
System.out.print("Enter number of Color: \n");
int num = read.nextInt();
for(int i = 0;i<num;i++){
System.out.print("Enter the Color: \n");
colorList[i] = read.next();
}//add the color
//print for(int i =0;i<colorList.length;i++){ System.out.print(colorList[i]);
}
// insert color at first index
System.out.print("Enter the new color: ");
for(int i=num;i>=0;i--){
colorList[i] = colorList[i+1];//reversalable
}
colorList[0] = read.next();
//print for(int i =0;i<colorList.length;i++){ System.out.print(colorList[i]);
//update index 4 System.out.print("Enter the new color to update index 4: "); colorList[3] = read.next();
//print for (i = 0;i<colorList.length;i++) { System.out.print(colorList[i]); } //remove 3rd index colorList[3] = null; for(int j=4;i<num;j--){ colorList[j] = colorList[j-1];
}
//print
for (i = 0;i<colorList.length;i++) {
System.out.print(colorList[i]);
}
//search
System.out.print("Enter the color that you want to search: ");
String target = read.next();
for (int index=0; index <num; index++){
if (colorList[index].equals(target)){
System.out.println("Found the element!");
System.out.println("The index of the element is "+index);
}
else
System.out.println("Data is not in the element!");
}
}
}//end main
}//end class '''java
/*
@author User */ public class ContiguousList {
/**
Kammalesh 253198 '''import java.util.Scanner; public class Contiguous_List { public static void main(String[] args) { String []element = new String[10]; int i=0; Scanner scan = new Scanner(System.in);
System.out.printf("Enter the number of color wanted to input : ");
int num = scan.nextInt();
for(i =0;i<num;i++){
System.out.printf("Enter the color %d : ",i+1);
element[i]=scan.next();
}
System.out.printf("\nEnter the First element : ");
for(i=num;i>=0;i--){
element[i+1]=element[i];
}
element[0]=scan.next();
num=num+1;
System.out.println("\nElement\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
System.out.printf("\nEnter the new color for element 5 : ");
element[4]=scan.next();
System.out.println("Element\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
int count = num;
for(i=num-1;i>=0;i--){
if((count-i)>=2){
element[count-i]=element[(count-i)+1];
}
}
num=num-1;
System.out.println("\nElement\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
System.out.println("\nThe third element is removed.");
System.out.printf("\nEnter the element wanted to search : ");
String search = scan.next();
for(i=0;i<num;i++){
if(search.equals(element[i])){
System.out.println("Found the element!");
System.out.printf("The index of the element is %d.\n", i);
}
}
} }'''java
(i)
import java.util.*;
public class DataStructure
{
public static void main(String[]args)
{
ArrayList color = new ArrayList();
color.add("Black");
color.add("White");
color.add("Blue");
color.add("Red");
color.add("Pink");
color.add("Yellow");
color.add("Purple");
color.add("Green");
color.add("Orange");
color.add("Grey");
System.out.println(color);
}
}
(ii)
import java.util.*;
public class DataStructure
{
public static void main(String[]args)
{
ArrayList <String> color = new ArrayList<String>();
color.add(0, "Black");
System.out.println(color);
}
}
(iii)
import java.util.*;
public class DataStructure
{
public static void main(String[]args)
{
ArrayList <String> color = new ArrayList<String>();
color.add("Black"); //(0)
color.add("White"); //(1)
color.add("Blue"); //(2)
color.add("Red"); //(3)
color.add("Pink"); //(4)
color.add("Yellow"); //(5)
color.add("Purple"); //(6)
color.add("Green"); //(7)
color.add("Orange"); //(8)
color.add("Grey"); //(9)
color.add(4,"Brown");
System.out.println(color);
}
}
(iv)
import java.util.*;
public class DataStructure
{
public static void main(String[]args)
{
ArrayList <String> color = new ArrayList<String>();
color.add("Black"); //(0)
color.add("White"); //(1)
color.add("Blue"); //(2)
color.add("Red"); //(3)
color.add("Pink"); //(4)
color.add("Yellow"); //(5)
color.add("Purple"); //(6)
color.add("Green"); //(7)
color.add("Orange"); //(8)
color.add("Grey"); //(9)
color.remove(2);
System.out.println(color);
}
}
(v)
import java.util.*;
public class DataStructure
{
public static void main(String[]args)
{
Scanner scan = new Scanner(System.in);
ArrayList <String> color = new ArrayList<String>();
color.add("Black"); //(0)
color.add("White"); //(1)
color.add("Blue"); //(2)
color.add("Red"); //(3)
color.add("Pink"); //(4)
color.add("Yellow"); //(5)
color.add("Purple"); //(6)
color.add("Green"); //(7)
color.add("Orange"); //(8)
color.add("Grey"); //(9)
System.out.print("Enter color you want to search: ");
String searchColor = scan.next();
for (int i=0; i<color.size(); i++){
if (searchColor.equals(color.get(i))){
System.out.println("Found the element");
System.out.println("The index for "+color.get(i)+" is "+i);
break;
}
}
}
}
HASVENI A/P SUBRAMANIAM (255301) '''import java.util.Scanner; public class Exercise5 { public static void main(String[] args) { String []element = new String[10]; int i=0; Scanner scan = new Scanner(System.in);
System.out.printf("Enter the number of color wanted to input : "); int num = scan.nextInt(); for(i =0;i<num;i++){ System.out.printf("Enter the color %d : ",i+1); element[i]=scan.next(); }
System.out.printf("\nEnter the First element : "); for(i=num;i>=0;i--){ element[i+1]=element[i]; } element[0]=scan.next(); num=num+1;
System.out.println("\nElement\t\t\tColor"); for(i=0;i<num;i++){ System.out.printf(" %-15d \t%s\n",i+1,element[i]); } System.out.printf("\nEnter the new color for element 5 : "); element[4]=scan.next();
System.out.println("Element\t\t\tColor"); for(i=0;i<num;i++){ System.out.printf(" %-15d \t%s\n",i+1,element[i]); }
int count = num; for(i=num-1;i>=0;i--){ if((count-i)>=2){ element[count-i]=element[(count-i)+1]; } } num=num-1;
System.out.println("\nElement\t\t\tColor"); for(i=0;i<num;i++){ System.out.printf(" %-15d \t%s\n",i+1,element[i]); } System.out.println("\nThe third element is removed.");
System.out.printf("\nEnter the element wanted to search : "); String search = scan.next();
for(i=0;i<num;i++){ if(search.equals(element[i])){ System.out.println("Found the element!"); System.out.printf("The index of the element is %d.\n", i); } } }
package ex5.contiguous.list;
/*
@author USER /import java.util.; public class Ex5ContiguousList {
/**
@param args the command line arguments */ public static void main(String[] args) { List list_String = new ArrayList(); list_String.add("purple"); list_String.add("blue"); list_String.add("yellow"); list_String.add("orange"); list_String.add("red"); list_String.add("violent"); list_String.add("tiffany"); list_String.add("black"); list_String.add("grey"); list_String.add("brown");
//print the list System.out.println(list_String); //insert a colour in the first position element in the list list_String.add(0,"light purple"); //print the list System.out.println(list_String); //update colour in element fourth list_String.set(3,"dark blue"); //print the list System.out.println(list_String); //remove the third element from the list list_String.remove(2); //print the list after third element deleted System.out.println("Third element removed:\t"+ list_String); //search an element if(list_String.contains("purple")){ System.out.println("Found the element"); }else{ System.out.println("There is no such element"); } } } output run: [purple, blue, yellow, orange, red, violent, tiffany, black, grey, brown] [light purple, purple, blue, yellow, orange, red, violent, tiffany, black, grey, brown] [light purple, purple, blue, dark blue, orange, red, violent, tiffany, black, grey, brown] Third element removed: [light purple, purple, dark blue, orange, red, violent, tiffany, black, grey, brown] Found the element BUILD SUCCESSFUL (total time: 0 seconds)
TAN WEI HOONG 253701 '''java import java.util.ArrayList; import java.util.*;
public class Colour {
public static void main(String[] args) {
Scanner sc= new Scanner (System.in); ArrayList list = new ArrayList<>(); list.add("red"); list.add("yellow"); list.add("white"); list.add("green"); list.add("blue"); list.add("purple "); list.add("grey"); list.add("black"); list.add("orange"); list.add("pink"); System.out.println(list);
list.add(0, "aero"); System.out.println("\nnew list : \n" + list);
list.set(3, "apple green"); System.out.println("After changing : \n"+list);
list.remove(2); System.out.println("After removing : \n"+ list);
System.out.println("Please enter a color : ");
String color = sc.nextLine();
if (list.contains(color))
{
System.out.println("Found the element!");
System.out.println("The index of "+ color +" is "+ list.indexOf(color));
}
else {
System.out.println("Error input");
}
}
}
'''java
FAUZIA ELSA FARAH (249865)
package contiguouslist;
import java.util.*;
/**
*
* @author A S U S
*/
public class ContiguousList {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String [] listcolors = new String[10];
//input number of list colors
System.out.print("Enter number of listcolors : ");
int num = scan.nextInt();
for(int i=0; i<num; i++){
System.out.print("Color "+(i+1) +" = ");
listcolors [i] = scan.next();
}
//Display number of list colors
System.out.println("List of Element\t\t List of Colors");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t\t "+listcolors[i]);
}
System.out.print("Enter the first element : ");
for (int i=num; i>=0; i--){
listcolors[i+1]=listcolors[i];
}
listcolors[0] = scan.next();
num=num+1;
System.out.println("List of Element\t\t List of Colors");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t "+listcolors[i]);
}
//add new color in element 4 wirh different color
System.out.print("Enter the new color for element 4 : ");
listcolors[3]=scan.next();
System.out.println("List of Element\t\t List of Colors");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t "+listcolors[i]);
}
int j = num;
for(int i=num-1;i>=0;i--){
if((j-i)>=2){
listcolors[j-i]=listcolors[(j-i)+1];
}
}
num=num-1;
System.out.println("List of Element\t\t List of Colors");
for(int i=0;i<num;i++){
System.out.println((i+1)+"\t\t "+listcolors[i]);
}
System.out.println("The third element is removed.");
System.out.print("Enter the element wanted to search : ");
String search = scan.nextLine();
//find and display the index of element
for(int i=0;i<num;i++){
if(search.equals(listcolors[i])){
System.out.println("Found the element");
System.out.println("The index of the element is " +i);
}
else
System.out.println("Element is not found!");
}
}
}
}
WEI JIACHENG (701465)
class List public class List { public int index; public String data; public List next;
public List(int id,String s) {
this.index=id;
this.data=s;
}
public void displayLink() {
System.out.println("{"+index+","+data+"}");
}
}
class LinkList public class LinkList { private List first; public LinkList() { first=null; } public void insertFirst(int id,String s) { List newLink=new List(id,s); newLink.next=first; first=newLink;
}
public void displayList()
{
System.out.print("List(first..>last):");
List current=first;
while(current!=null)
{
current.displayLink();
current=current.next;
}
System.out.println();
}
public void delete(int key)
{
List current=first;
List previous=first;
while(current.index!=key)
{
if(current.next==null)
System.out.println("not found");
else
{
previous=current;
current=current.next;
}
}
if(current==first)
first=first.next;
else
previous.next=current.next;
System.out.println();
}
public void find(String key) {
List current=first;
while(current.data!=key)
{
if(current.next==null)
{ System.out.println("not found");
return ;
}
else
current=current.next;
}
System.out.println("Found the element"+current.index);
}
}
*class ListApp public class LinkApp {
public static void main(String[] args) {
LinkList a=new LinkList();
a.insertFirst(9, "blue");
a.insertFirst(8, "green");
a.insertFirst(7, "yellow");
a.insertFirst(6, "pink");
a.insertFirst(5, "red");
a.insertFirst(4, "purple");
a.insertFirst(3, "gray");
a.insertFirst(2, "white");
a.insertFirst(1, "black");
a.insertFirst(0, "orange");
a.displayList();
a.insertFirst(10, "brown");
a.displayList();
a.delete(3);
a.insertFirst(3, "PeachPuff");
a.delete(1);
a.displayList();
a.find("red");
a.displayList();
a.find("ccc");
a.displayList();
}
}
Beh Choon Huat 253302 import java.util.Scanner; public class Contiguous_List { public static void main(String[] args) { String []element = new String[10]; int i=0; Scanner scan = new Scanner(System.in);
System.out.printf("Enter the number of color wanted to input : ");
int num = scan.nextInt();
for(i =0;i<num;i++){
System.out.printf("Enter the color %d : ",i+1);
element[i]=scan.next();
}
System.out.printf("\nEnter the First element : ");
for(i=num;i>=0;i--){
element[i+1]=element[i];
}
element[0]=scan.next();
num=num+1;
System.out.println("\nElement\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
System.out.printf("\nEnter the new color for element 5 : ");
element[4]=scan.next();
System.out.println("Element\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
int count = num;
for(i=num-1;i>=0;i--){
if((count-i)>=2){
element[count-i]=element[(count-i)+1];
}
}
num=num-1;
System.out.println("\nElement\t\t\tColor");
for(i=0;i<num;i++){
System.out.printf(" %-15d \t%s\n",i+1,element[i]);
}
System.out.println("\nThe third element is removed.");
System.out.printf("\nEnter the element wanted to search : ");
String search = scan.next();
for(i=0;i<num;i++){
if(search.equals(element[i])){
System.out.println("Found the element!");
System.out.printf("The index of the element is %d.\n", i);
}
}
}
Mohammad Faris Bin Ahmad Shukri 255312
Exercise 1
public class Exercise1 {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
List
Output :+1:
Exercise 2
import java.util.ArrayList; import java.util.List;
/*
Output :+1:
Exercise 3
import java.util.ArrayList; import java.util.List;
/*
@author root */ public class exercise3 {
public static void main(String[] args) {
// Creae a list and add some colors to the list
List
Output :+1:
Exercise 4
import java.util.*;
/*
Output :+1:
Exercise 5
import java.util.*; import static jdk.nashorn.internal.objects.NativeString.search;
/*
Output :+1:
import java.util.Scanner;
public class colours
{
public static void main(String[]args)
{
Scanner scan = new Scanner(System.in);
String [] colour = new String [10];
int size = 4;
String newColour;
System.out.println("Enter 4 colours: ");
for(int i=0 ; i<size ; i++)
{
colour[i] = scan.next();
}
System.out.println("Colours");
for(int i=0; i<size ; i++)
{
System.out.println(colour[i]);
}
System.out.print("Add new colour in the first index: ");
newColour=scan.next();
System.out.println("");
addColour(colour,size,newColour);
System.out.println("");
String changeColour=colour[3];
editColour(colour,changeColour,size);
String target=colour[2];
removeColour(colour,target,size);
System.out.println("");
System.out.print("Search: ");
String searchColour=scan.next();
for(int i=0;i<size;i++)
{
if(colour[i].equals(searchColour))
{
System.out.println("The colour "+colour[i]+" is at index "+i);
}
else
{
System.out.println("The colour is not listed");
}
}
}
public static void addColour(String []colour,int size,String newColour)
{
if(size==0)
{
colour[0]=newColour;
size++;
}
else if(size==10)
{
System.out.println("The list is full");
}
else
{
int index=0;
for(int x=size;x>index;x--)
{
colour[x]=colour[x-1];
}
colour[index]=newColour;
size++;
}
System.out.println("Display colour in the list after change the new colour ");
for(int i=0;i<size;i++)
{
System.out.println(colour[i]);
}
}
public static void editColour(String []colour,String changeColour,int size)
{
boolean edit=false;
for(int i=0;i<size;i++)
if(colour[i].equals(changeColour))
{
System.out.print("Enter new colour:");
string colour=scan.next();
edit=true;
break;
}
if(edit==false){
System.out.println("The colour is not in the list ");}
System.out.println("");
System.out.println("Display colour in the list after element 4 change to new colour");
for(int i=0;i<5;i++)
{
System.out.println(colour[i]);
}
}
public static void removeColour(String []colour,String target,int size)
{
int i,j;
boolean delete=false;
for(i=0;i<size;i++){
if(colour[i].equals(target))
{
for(j=i;j<5-1;j++)
colour[j]=colour[j+1];
delete=true;
break;
}
}
if(delete==false)
{
System.out.println("The colour is not listed");
}
System.out.println("");
System.out.println("Display colour in the list after delate the third element in list colour");
for( i=0;i<size;i++)
{
System.out.println(colour[i]);
}
}}
```java
`
import java.util.*;
public class Exercise1 {
public static void main(String[] args) {
List
list_Strings.add(0, "BROWN");
System.out.println(list_Strings);
list_Strings.set(3, "PINK");
System.out.println(list_Strings);
list_Strings.remove(2); System.out.println(list_Strings);
if (list_Strings.contains("WHITE")) {
System.out.println("Found the element");
}
else {
System.out.println("There is no such element");
}
} } `
package exercise5;
import java.util.Scanner;
public class Exercise5 {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
String myList[]= new String[10]; //create new list
System.out.print("Number of Color > ");
int num = scan.nextInt();
//add
for(int i=0; i<num; i++){
System.out.print("Color "+ (i+1)+" : ");
myList[i] = scan.next();
}
//insert element into 1st position
System.out.print("\nFirst Color : ");
for(int i=num; i>=0; i--){
myList[i+1] = myList[i];
}
myList[0] = scan.next();
num += 1;
for(int i=0; i<num; i++){
System.out.println("Color "+ (i+1)+" : "+ myList[i]);
}
//update element(index of 4)
System.out.print("\nNew 5th Color : ");
myList[4] = scan.next();
for(int i=0; i<num; i++){
System.out.println("Color "+ (i+1)+" : "+ myList[i]);
}
//remove third element(index of 2)
int count = num;
for(int i=num-1; i>=0; i--){
if((count-i)>=2)
myList[count-i] = myList[(count-i)+1];
}
num -= 1;
System.out.println();
for(int i=0; i<num; i++){
System.out.println("Color "+ (i+1)+" : "+ myList[i]);
}
//search & find index of element
System.out.print("\nSearching Element : ");
String search = scan.next();
for(int i=0; i<num; i++){
if(search.equals(myList[i])){
System.out.println("Found the element!");
System.out.println("Index of the element is "+(i));
}
}
}
}
```java
(i) Write a java program to create a new list of 10 elements, add some colors and print the list. (ii) Insert an element into the array list at the first position,print the list. (iii) Update color in element (4) with different color, print the list. (iv) remove the third element from the list, print the list (v) search an element in the array list, print out "Found the element" if it is found. Find the index of that element and print the index no.