NumPy is a foundational package for numerical computing in Python.
NumPy هي حزمة أساسية للحوسبة الرقمية في بايثون
It provides efficient multidimensional arrays for fast array-oriented arithmetic operations and flexible broadcasting capabilities.
يوفر مصفوفات متعددة الأبعاد للحساب السريع الموجه نحو المصفوفة والبث المرن
NumPy also includes mathematical functions, tools for reading/writing array data, linear algebra, random number generation, and Fourier transform capabilities.
يحتوي NumPy على وظائف رياضية وأدوات لـ I / O والجبر الخطي وتوليد الأرقام العشوائية وقدرات تحويل فورييه
NumPy's easy-to-use C API allows for easy integration with external libraries written in C/C++/Fortran.
تسمح واجهة برمجة تطبيقات C الخاصة به بالتكامل السهل مع المكتبات المكتوبة بلغة C أو C ++ أو FORTRAN
NumPy is important for numerical computations in Python because it is designed for efficiency on large arrays of data.
يُستخدم NumPy لتغليف قواعد الرموز القديمة ومنحها واجهة ديناميكية
NumPy provides fast vectorized array operations, common array algorithms, efficient descriptive statistics, data alignment and relational data manipulations, and group-wise data manipulations.
NumPy مهم للمعالجة الفعالة لمصفوفات كبيرة من البيانات يوفر عمليات صفيف متجهية سريعة ، وخوارزميات مصفوفة مشتركة ، وإحصاءات وصفية فعالة ، ومحاذاة البيانات ومعالجة البيانات العلائقية ، ومعالجة البيانات على مستوى المجموعة.
While NumPy provides a computational foundation for general numerical data processing, pandas is typically used as the basis for statistics and analytics on tabular data.
NumPy was created in 2005 from the Numeric and Numarray projects to unify the array programming community in Python.
تم تصميم NumPy لتحقيق الكفاءة مع تخزين الذاكرة المتجاورة والحسابات المعقدة على المصفوفات بأكملها دون الحاجة إلى حلقات Python
NumPy arrays are significantly faster than Python lists for large datasets
تعد مصفوفات NumPy أسرع بكثير من قوائم Python لمجموعات البيانات الكبيرة
NumPy-based algorithms can be 10 to 100 times faster than pure Python counterparts
يمكن أن تكون الخوارزميات المستندة إلى NumPy أسرع من 10 إلى 100 مرة من نظيراتها في Python
NumPy arrays use significantly less memory than built-in Python sequences
تستخدم مصفوفات NumPy ذاكرة أقل بشكل ملحوظ من تسلسلات Python المضمنة
NumPy uses optimized C code to perform array operations, allowing for fast and efficient computation
يستخدم NumPy رمز C المحسن لأداء عمليات الصفيف ، مما يسمح بحساب سريع وفعال
import numpy as np
my_arr = np.arange(1000000)
my_list = list(range(1000000))
0]: %time for _ in range(10): my_arr2 = my_arr * 2 CPU times: user 20 ms, sys: 50 ms, total: 70 ms
Wall time: 72.4 ms
%time for _ in range(10): my_list2 = [x * 2 for x in my_list] CPU times: user 760 ms, sys: 290 ms, total: 1.05 s
Wall time: 1.05 s
المثال السابق يوضح الفرق في الأداء بين NumPy arrays وPython lists. تم إنشاء مصفوفة NumPy باستخدام np.arange() وتم إنشاء قائمة Python بإستخدام list(). تم ضرب كلا الكائنين بعد ذلك برقم 2 باستخدام * 2.
تم استخدام %time لقياس وقت التنفيذ لكلا العمليات، حيث وجد أن عملية ضرب المصفوفة NumPy برقم 2 قد استغرقت 70 ملي ثانية، بينما استغرقت عملية ضرب القائمة Python برقم 2 حوالي 1.05 ثانية.
يظهر هذا المثال فارق الأداء الكبير بين NumPy arrays وPython lists، حيث أن عمليات NumPy تتم بشكل أسرع بكثير وتستخدم أقل كمية من الذاكرة. هذا يجعل NumPy مفيدًا بشكل خاص للتعامل مع البيانات الكبيرة و التحليل العلمي
Q
1. Why is NumPy important for numerical computing in Python?
a. It provides modeling and scientific functionality
b. It is designed for efficiency on large arrays of data
c. It is easy to use with external libraries
d. It provides an easy-to-use interface for legacy codebases
2. Which of the following is an advantage of using NumPy arrays over built-in Python sequences?
a. NumPy arrays use more memory
b. NumPy arrays perform operations using Python for loops
c. NumPy arrays internally store data in a contiguous block of memory
d. NumPy arrays cannot be used with external libraries
3. What are some of the things you'll find in NumPy?
a. Descriptive statistics and aggregating/summarizing data
b. Tools for reading/writing array data to disk
c. Mathematical functions for fast operations on entire arrays of data
d. All of the above
4. What is an advantage of using NumPy's C API?
a. It makes it possible to use Python to wrap legacy C/C++/Fortran codebases
b. It provides an easy-to-use interface for scientific functionality
c. It allows for easy installation of external libraries
d. It makes it possible to write array expressions using loops
5. What is an example of array-oriented computing in Python?
a. Using if-elif-else loops for conditional logic
b. Performing complex computations on entire arrays with NumPy
c. Using built-in Python sequences for data manipulation
d. None of the above
6. What is the example given in the paragraph comparing?
a. NumPy arrays and Python lists
b. NumPy arrays and NumPy arrays with broadcasting
c. Python lists and Python dictionaries
d. NumPy arrays and NumPy arrays with slicing
2. What is the benefit of using NumPy-based algorithms?
a. They use more memory than their pure Python counterparts
b. They are generally slower than their pure Python counterparts
c. They are generally faster than their pure Python counterparts and use significantly less memory
d. They are more difficult to use than their pure Python counterparts
3. What is the purpose of using %time in the example?
a. To measure the time it takes to execute a block of code
b. To compare the performance of different algorithms
c. To debug errors in the code
d. To write array expressions using loops
4. What is the significance of the performance difference between NumPy arrays and Python lists?
a. NumPy arrays are always faster than Python lists
b. NumPy arrays are sometimes faster than Python lists depending on the use case
c. Python lists are always faster than NumPy arrays
d. It has no significance
5. What is the advantage of using NumPy arrays over Python lists?
a. NumPy arrays are easier to use than Python lists
b. NumPy arrays use more memory than Python lists
c. NumPy arrays are generally faster than Python lists
d. NumPy arrays are more flexible than Python lists
The NumPy ndarray: A Multidimensional Array Object
NumPy is a Python library for numerical computing.
NumPy هي مكتبة Python للحوسبة الرقمية.
Its key feature is the N-dimensional array object or ndarray, which is a fast, flexible container for large datasets in Python.
ميزته الرئيسية هي كائن المصفوفة N-dimensional أو ndarray ، وهي حاوية سريعة ومرنة لمجموعات البيانات الكبيرة في Python.
Arrays enable mathematical operations on whole blocks of data using similar syntax to the equivalent operations between scalar elements.
تتيح المصفوفات العمليات الحسابية على كتل كاملة من البيانات باستخدام بناء جملة مماثل للعمليات المكافئة بين العناصر العددية.
The numpy namespace contains a number of functions whose names conflict with built-in Python functions, so using import numpy as np is recommended.
تحتوي مساحة الاسم numpy على عدد من الوظائف التي تتعارض أسماؤها مع وظائف Python المضمنة ، لذا يوصى باستخدام import numpy كـ np.
An ndarray is a generic multidimensional container for homogeneous data, with a shape and dtype indicating the size of each dimension and the data type of the array.
ndarray عبارة عن حاوية عامة متعددة الأبعاد للبيانات المتجانسة ، مع شكل ونوع dtype يشير إلى حجم كل بُعد ونوع بيانات المصفوفة.
Proficiency in array-oriented programming and thinking is a key step towards becoming a scientific Python guru.
تعد الكفاءة في البرمجة والتفكير الموجهين نحو المصفوفة خطوة أساسية نحو أن تصبح خبيرًا علميًا في بايثون.
Q
_ 1. What is the key feature of NumPy?
a. Its ability to use external libraries
b. Its N-dimensional array object, or ndarray
c. Its ability to work with non-homogeneous data
d. Its built-in machine learning algorithms
What is the advantage of using NumPy arrays for mathematical operations?
a. They use different syntax than scalar values on built-in Python objects
b. They allow you to perform mathematical operations on whole blocks of data
c. They are slower than equivalent operations between scalar elements
d. They are only useful for non-numeric data
What is the significance of the examples given in the paragraph?
a. They demonstrate how to use NumPy arrays for non-mathematical operations
b. They highlight the similarities between scalar values and NumPy arrays in terms of syntax
c. They show that NumPy arrays are slower than equivalent operations between scalar elements
d. They demonstrate how to use built-in Python objects for mathematical operations
What is the purpose of using import numpy as np instead of from numpy import *?
a. To make the code shorter and easier to read
b. To avoid conflicts with built-in Python functions
c. To make NumPy functions run faster
d. To make it easier to use external libraries with NumPy
What is an ndarray?
a. A container for non-homogeneous data
b. A container for scalar values in Python
c. A generic multidimensional container for homogeneous data
d. A container for non-numeric data_
Creating ndarrays
The easiest way to create an array in NumPy is to use the array function, which accepts any sequence-like object and produces a new NumPy array containing the passed data.
أسهل طريقة لإنشاء مصفوفة في NumPy هي استخدام دالة المصفوفة ، والتي تقبل أي كائن يشبه التسلسل وتنتج مصفوفة NumPy جديدة تحتوي على البيانات التي تم تمريرها.
Nested sequences like a list of equal-length lists will be converted into a multidimensional array.
سيتم تحويل التسلسلات المتداخلة مثل قائمة قوائم متساوية الطول إلى مصفوفة متعددة الأبعاد.
The shape of the array is inferred from the data, and can be confirmed using the ndim and shape attributes.
يُستدل على شكل المصفوفة من البيانات ، ويمكن تأكيده باستخدام سمات ndim و shape.
Unless explicitly specified, np.array tries to infer a good data type for the array that it creates.
ما لم ينص صراحة ، np. يحاول المصفوفة استنتاج نوع بيانات جيد للمصفوفة التي يقوم بإنشائها.
Other functions for creating new arrays include zeros, ones, and empty, which create arrays of 0s or 1s with a given length or shape, or an array without initializing its values to any particular value.
تتضمن الوظائف الأخرى لإنشاء مصفوفات جديدة الأصفار ، والآحاد ، والفارغة ، والتي تنشئ مصفوفات من 0 أو 1 بطول أو شكل معين ، أو مصفوفة بدون تهيئة قيمها إلى أي قيمة معينة.
To create a higher dimensional array with these methods, pass a tuple for the shape.
لإنشاء مصفوفة ذات أبعاد أعلى بهذه الطرق ، قم بتمرير مجموعة للشكل.
It's not safe to assume that np.empty will return an array of all zeros, as it may return uninitialized "garbage" values.
ليس من الآمن افتراض أن np. سيعيد فارغ مصفوفة من جميع الأصفار ، لأنه قد يُرجع قيمًا غير مهيأة "غير صالحة".
arange is an array-valued version of the built-in Python range function.
arange هو نسخة ذات قيمة مصفوفة لوظيفة نطاق Python المدمجة.
NumPy has a short list of standard array creation functions, focused on numerical computing.
يحتوي NumPy على قائمة قصيرة من وظائف إنشاء المصفوفات القياسية ، تركز على الحوسبة الرقمية.
If the data type is not specified, it will in many cases be float64 (floating point).
إذا لم يتم تحديد نوع البيانات ، فسيكون في كثير من الحالات float64 (النقطة العائمة).
Array creation functions
Here are some examples of array creation functions in NumPy:
np.array(): Create an array from a Python list or tuple.
These functions can be useful for a variety of tasks, such as creating coordinate grids, repeating arrays, and creating arrays of indices.
Q
Write a Python function that takes an integer n as input and returns an n-by-n identity matrix as a NumPy array.
import numpy as np
def identity_matrix(n):
"""
Returns the n-by-n identity matrix as a NumPy array.
"""
return np.eye(n)
# Example usage:
arr = identity_matrix(3)
print(arr)
Output:
array([[1., 0., 0.],
[0., 1., 0.],
[0., 0., 1.]])
Write a Python function that takes a NumPy array as input and returns a new array with all of the negative elements set to zero.
import numpy as np
def set_negatives_to_zero(arr):
"""
Returns a new array with all of the negative elements of arr set to zero.
"""
arr[arr < 0] = 0
return arr
# Example usage:
arr = np.array([-1, 2, -3, 4, -5])
new_arr = set_negatives_to_zero(arr)
print(new_arr)
Output:
array([0, 2, 0, 4, 0])
Write a Python function that takes a NumPy array as input and returns a new array with the same shape as the input array, but with all of the elements set to zero.
import numpy as np
def create_zero_array(arr):
"""
Returns a new array with the same shape as arr, but with all elements set to zero.
"""
return np.zeros_like(arr)
# Example usage:
arr = np.array([[1, 2, 3], [4, 5, 6]])
new_arr = create_zero_array(arr)
print(new_arr)
Output:
array([[0, 0, 0],
[0, 0, 0]])
Write a Python function that takes a NumPy array as input and returns a new array with the same shape as the input array, but with all of the elements set to one.
import numpy as np
def create_one_array(arr):
"""
Returns a new array with the same shape as arr, but with all elements set to one.
"""
return np.ones_like(arr)
# Example usage:
arr = np.array([[1, 2, 3], [4, 5, 6]])
new_arr = create_one_array(arr)
print(new_arr)
Output:
array([[1, 1, 1],
[1, 1, 1]])
Write a Python function that takes two NumPy arrays as input and returns a new array that is the concatenation of the two input arrays along the second axis.
import numpy
6. Write a Python function that takes a NumPy array as input and returns a new array with the same shape as the input array, but with each element replaced by its absolute value.
```python
import numpy as np
def absolute_array(arr):
"""
Returns a new array with the same shape as arr, but with each element replaced by its absolute value.
"""
return np.abs(arr)
# Example usage:
arr = np.array([-1, 2, -3, 4, -5])
new_arr = absolute_array(arr)
print(new_arr)
Output:
array([1, 2, 3, 4, 5])
Write a Python function that takes a NumPy array as input and returns a new array with all of the elements normalized to have a mean of zero and a standard deviation of one.
import numpy as np
def normalize_array(arr):
"""
Returns a new array with all elements of arr normalized to have a mean of zero and a standard deviation of one.
"""
arr_mean = np.mean(arr)
arr_std = np.std(arr)
return (arr - arr_mean) / arr_std
# Example usage:
arr = np.array([1, 2, 3, 4, 5])
new_arr = normalize_array(arr)
print(new_arr)
Write a Python function that takes a NumPy array as input and returns a new array with the same shape as the input array, but with all of the elements squared.
import numpy as np
def square_array(arr):
"""
Returns a new array with the same shape as arr, but with all elements squared.
"""
return np.square(arr)
# Example usage:
arr = np.array([1, 2, 3, 4, 5])
new_arr = square_array(arr)
print(new_arr)
Output:
array([ 1, 4, 9, 16, 25])
Write a Python function that takes a NumPy array as input and returns a new array with the same shape as the input array, but with all of the elements cubed.
import numpy as np
def cube_array(arr):
"""
Returns a new array with the same shape as arr, but with all elements cubed.
"""
return np.power(arr, 3)
# Example usage:
arr = np.array([1, 2, 3, 4, 5])
new_arr = cube_array(arr)
print(new_arr)
Output:
array([ 1, 8, 27, 64, 125])
Write a Python function that takes a NumPy array as input and returns a new array with the same shape as the input array, but with all of the elements rounded to the nearest integer.
import numpy as np
def round_array(arr):
"""
Returns a new array with the same shape as arr, but with all elements rounded to the nearest integer.
"""
return np.round(arr)
# Example usage:
arr = np.array([1.2, 2.7, 3.5, 4.8, 5.1])
new_arr = round_array(arr)
print(new_arr)
Output:
array([1., 3., 4., 5., 5.])
Arithmetic with NumPy Arrays
Write a Python function that takes two NumPy arrays as input and returns a new array that is the element-wise sum of the two input arrays.
import numpy as np
def add_arrays(arr1, arr2):
"""
Returns a new array that is the element-wise sum of arr1 and arr2.
"""
return np.add(arr1, arr2)
# Example usage:
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([5, 4, 3, 2, 1])
new_arr = add_arrays(arr1, arr2)
print(new_arr)
Output:
array([6, 6, 6, 6, 6])
Write a Python function that takes two NumPy arrays as input and returns a new array that is the element-wise difference of the two input arrays.
import numpy as np
def subtract_arrays(arr1, arr2):
"""
Returns a new array that is the element-wise difference of arr1 and arr2.
"""
return np.subtract(arr1, arr2)
# Example usage:
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([5, 4, 3, 2, 1])
new_arr = subtract_arrays(arr1, arr2)
print(new_arr)
Output:
array([-4, -2, 0, 2, 4])
Write a Python function that takes two NumPy arrays as input and returns a new array that is the element-wise product of the two input arrays.
import numpy as np
def multiply_arrays(arr1, arr2):
"""
Returns a new array that is the element-wise product of arr1 and arr2.
"""
return np.multiply(arr1, arr2)
# Example usage:
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([5, 4, 3, 2, 1])
new_arr = multiply_arrays(arr1, arr2)
print(new_arr)
Output:
array([5, 8, 9, 8, 5])
Write a Python function that takes two NumPy arrays as input and returns a new array that is the element-wise division of the two input arrays.
import numpy as np
def divide_arrays(arr1, arr2):
"""
Returns a new array that is the element-wise division of arr1 and arr2.
"""
return np.divide(arr1, arr2)
# Example usage:
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([5, 4, 3, 2, 1])
new_arr = divide_arrays(arr1, arr2)
print(new_arr)
Output:
array([0.2 , 0.5 , 1. , 2. , 5. ])
Write a Python function that takes a NumPy array as input and returns a new array that is the element-wise square root of the input array.
import numpy as np
def sqrt_array(arr):
"""
Returns a new array that is the element-wise square root of arr.
"""
return np.sqrt(arr)
# Example usage:
arr = np.array([1, 4, 9, 16, 25])
new_arr = sqrt_array(arr)
print(new_arr)
Introduction to the numpy library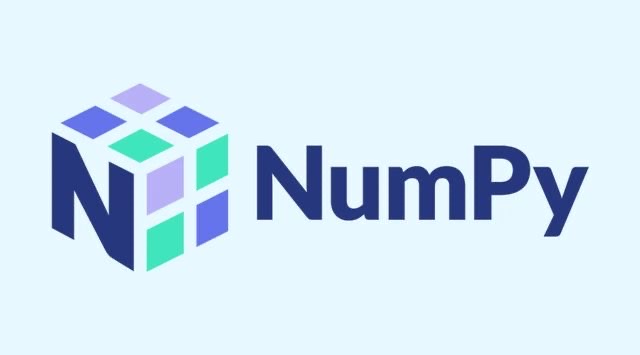
المثال السابق يوضح الفرق في الأداء بين NumPy arrays وPython lists. تم إنشاء مصفوفة NumPy باستخدام
np.arange()
وتم إنشاء قائمة Python بإستخدامlist()
. تم ضرب كلا الكائنين بعد ذلك برقم 2 باستخدام* 2
. تم استخدام%time
لقياس وقت التنفيذ لكلا العمليات، حيث وجد أن عملية ضرب المصفوفة NumPy برقم 2 قد استغرقت 70 ملي ثانية، بينما استغرقت عملية ضرب القائمة Python برقم 2 حوالي 1.05 ثانية. يظهر هذا المثال فارق الأداء الكبير بين NumPy arrays وPython lists، حيث أن عمليات NumPy تتم بشكل أسرع بكثير وتستخدم أقل كمية من الذاكرة. هذا يجعل NumPy مفيدًا بشكل خاص للتعامل مع البيانات الكبيرة و التحليل العلمي Q1. Why is NumPy important for numerical computing in Python? a. It provides modeling and scientific functionality b. It is designed for efficiency on large arrays of data c. It is easy to use with external libraries d. It provides an easy-to-use interface for legacy codebases 2. Which of the following is an advantage of using NumPy arrays over built-in Python sequences? a. NumPy arrays use more memory b. NumPy arrays perform operations using Python for loops c. NumPy arrays internally store data in a contiguous block of memory d. NumPy arrays cannot be used with external libraries 3. What are some of the things you'll find in NumPy? a. Descriptive statistics and aggregating/summarizing data b. Tools for reading/writing array data to disk c. Mathematical functions for fast operations on entire arrays of data d. All of the above
4. What is an advantage of using NumPy's C API? a. It makes it possible to use Python to wrap legacy C/C++/Fortran codebases b. It provides an easy-to-use interface for scientific functionality c. It allows for easy installation of external libraries d. It makes it possible to write array expressions using loops
5. What is an example of array-oriented computing in Python? a. Using if-elif-else loops for conditional logic b. Performing complex computations on entire arrays with NumPy c. Using built-in Python sequences for data manipulation d. None of the above
6. What is the example given in the paragraph comparing? a. NumPy arrays and Python lists b. NumPy arrays and NumPy arrays with broadcasting c. Python lists and Python dictionaries d. NumPy arrays and NumPy arrays with slicing
2. What is the benefit of using NumPy-based algorithms? a. They use more memory than their pure Python counterparts b. They are generally slower than their pure Python counterparts c. They are generally faster than their pure Python counterparts and use significantly less memory d. They are more difficult to use than their pure Python counterparts
3. What is the purpose of using
%time
in the example? a. To measure the time it takes to execute a block of code b. To compare the performance of different algorithms c. To debug errors in the code d. To write array expressions using loops4. What is the significance of the performance difference between NumPy arrays and Python lists? a. NumPy arrays are always faster than Python lists b. NumPy arrays are sometimes faster than Python lists depending on the use case c. Python lists are always faster than NumPy arrays d. It has no significance
5. What is the advantage of using NumPy arrays over Python lists? a. NumPy arrays are easier to use than Python lists b. NumPy arrays use more memory than Python lists c. NumPy arrays are generally faster than Python lists d. NumPy arrays are more flexible than Python lists
The NumPy ndarray: A Multidimensional Array Object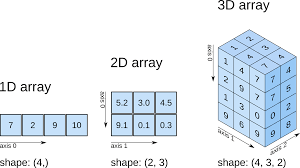
What is the advantage of using NumPy arrays for mathematical operations? a. They use different syntax than scalar values on built-in Python objects b. They allow you to perform mathematical operations on whole blocks of data c. They are slower than equivalent operations between scalar elements d. They are only useful for non-numeric data
What is the significance of the examples given in the paragraph? a. They demonstrate how to use NumPy arrays for non-mathematical operations b. They highlight the similarities between scalar values and NumPy arrays in terms of syntax c. They show that NumPy arrays are slower than equivalent operations between scalar elements d. They demonstrate how to use built-in Python objects for mathematical operations
What is the purpose of using import numpy as np instead of from numpy import *? a. To make the code shorter and easier to read b. To avoid conflicts with built-in Python functions c. To make NumPy functions run faster d. To make it easier to use external libraries with NumPy
What is an ndarray? a. A container for non-homogeneous data b. A container for scalar values in Python c. A generic multidimensional container for homogeneous data d. A container for non-numeric data_ Creating ndarrays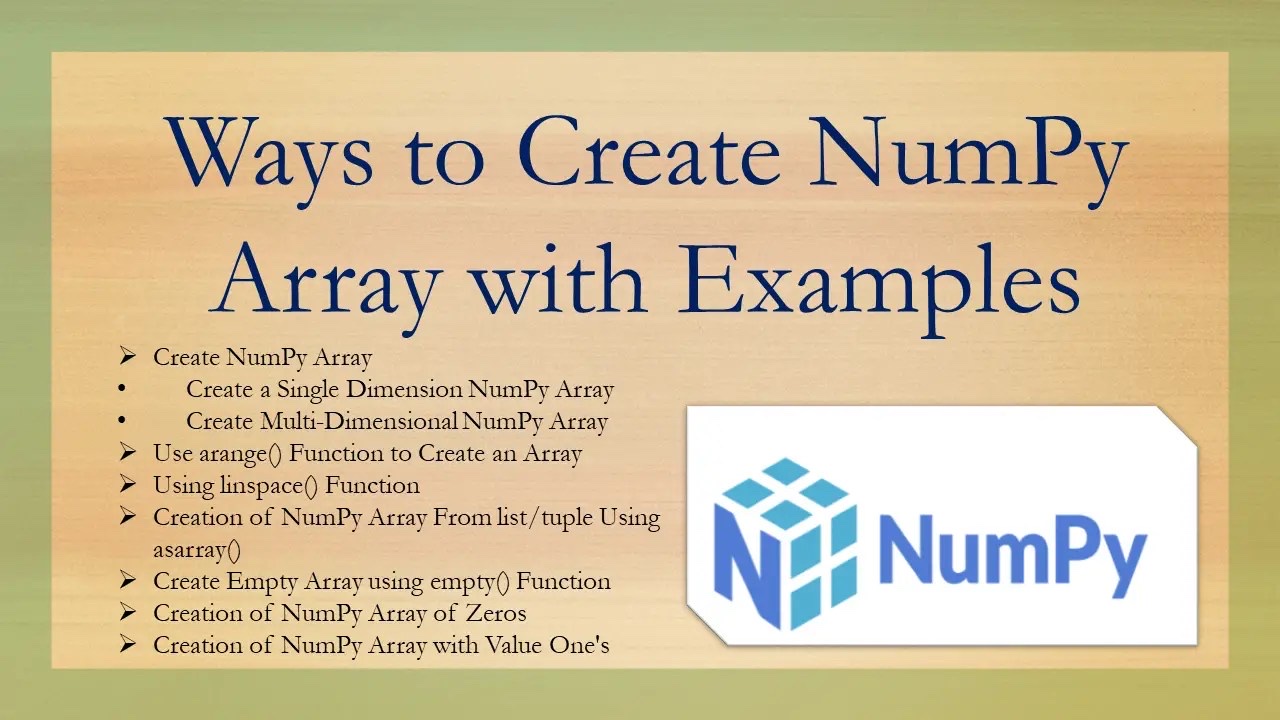
np.array(): Create an array from a Python list or tuple.
np.zeros(): Create an array of zeros with a specified shape.
np.ones(): Create an array of ones with a specified shape.
np.empty(): Create an uninitialized array with a specified shape. Note that the values in the array are not set to zero and may be random.
np.arange(): Create an array with evenly spaced values within a given interval.
np.linspace(): Create an array with a specified number of evenly spaced values within a given interval.
np.eye(): Create a 2-D array with ones on the diagonal and zeros elsewhere.
np.diag(): Create a diagonal array with specified diagonal values.
np.random.rand(): Create an array of random values with a specified shape, drawn from a uniform distribution over [0, 1).
np.random.randn(): Create an array of random values with a specified shape, drawn from a standard normal distribution (mean=0, std=1).
np.random.randint(): Create an array of random integers with a specified shape, drawn from a discrete uniform distribution over a specified interval.
These functions can be very useful for creating arrays with specific shapes and values for use in various mathematical and statistical operations.
Sure, here are a few more array creation functions in NumPy:
np.full(): Create an array with a specified shape and fill value.
np.tile(): Create a new array by repeating an existing array a specified number of times along specified axes.
np.meshgrid(): Create coordinate matrices from coordinate vectors.
np.indices(): Create an array of indices into an array with a specified shape.
These functions can be useful for a variety of tasks, such as creating coordinate grids, repeating arrays, and creating arrays of indices. Q
Output:
Output:
Output:
Output:
Output:
Output:
Output:
Output:
Output:
Arithmetic with NumPy Arrays
Output:
Output:
Output:
Output:
Output: