Open helloyoucan opened 3 years ago
Describe the bug When I used Animation on the mobile side, there was a bug
To Reproduce Look at the gif.
Information
Versions - Look in your package.json for this information: "react": "^16.12.0", "react-dom": "^16.12.0", "react-draggable": "^4.4.3", "react-sortablejs": "^6.0.0", "sortablejs": "^1.12.0", "umi": "^3.2.27", Additional context
package.json
// index.tsx import React, { useState } from 'react'; import { ReactSortable } from 'react-sortablejs'; import styles from './index.less'; export default () => { const [listRow, setListRow] = useState([ { id: 1, name: 'shrek' }, { id: 2, name: 'fiona' }, { id: 3, name: 'mike' }, { id: 4, name: 'luxi' }, ]); const [listColumn, setListColumn] = useState<Array<{id:string,name:string}>>([]); return ( <div className={styles.container}> <ReactSortable className={styles.column} group="shared" list={listColumn} setList={setListColumn} fallbackOnBody={false} emptyInsertThreshold={30} invertSwap={true} dragClass={styles.dragClass} ghostClass={styles.ghostClass} > {listColumn.map(item => ( <div className={styles.block} key={item.id}>{item.name}</div> ))} </ReactSortable> <ReactSortable className={styles.row} group="shared" list={listRow} dragClass={styles.dragClass} ghostClass={styles.ghostClass} setList={setListRow} animation={150} > {listRow.map(item => ( <div className={styles.block} key={item.id}>{item.name}</div> ))} </ReactSortable> </div> ); };
// index.less .container { height: 100vh; width: 100vw; background-color: #eee; } .column{ position: absolute; left: 20vw; height: 10vw; min-width: 10vw; top: 10px; background-color: #fff; display: flex; } .row{ position: absolute; left: 10px; min-height: 10vw; width: 10vw; top: 1vw; background-color:#000; flex-wrap: wrap; } .block{ width: 10vw; height: 10vw; background-color: green; font-size: 20px; text-align: center; line-height: 10vw; } .dragClass{ background-color: hotpink; opacity: 1 !important; } .ghostClass{ background-color: yellow; }
Describe the bug When I used Animation on the mobile side, there was a bug
To Reproduce Look at the gif.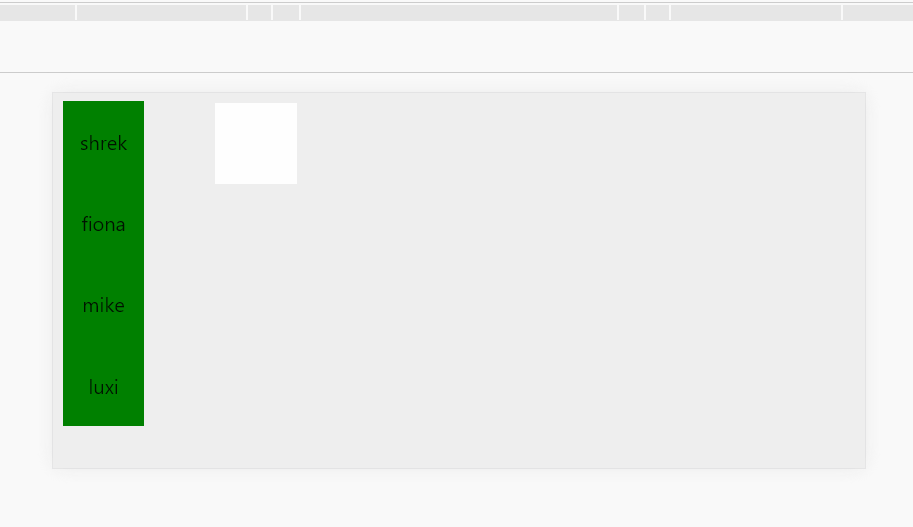
Information
Versions - Look in your
package.json
for this information: "react": "^16.12.0", "react-dom": "^16.12.0", "react-draggable": "^4.4.3", "react-sortablejs": "^6.0.0", "sortablejs": "^1.12.0", "umi": "^3.2.27", Additional context