Open dawarepramod4 opened 7 months ago
I'm having the same issue with no solution so far :(
This issue may happen cuz your content inside the HTML(data: String)
have some special characters you can use a small function to refactor that String
to clear out the special characters in it.
String htmlContent = "";
String cleanedHtmlContent = "";
formatDescription() {
setState(
(() {
htmlContent = data.content;
cleanedHtmlContent = htmlContent.replaceAll(RegExp(r'<[^>]*>'), '');
}),
);
}
@override
void initState() {
super.initState();
formatDescription();
}
HTML(data: cleanedHtmlContent)
Just replace the data.content
with your desired String
and it should work after this. 😁
"I also encountered the same issue, and as a solution, I used the provided code."
data.replaceAll('font-feature-settings: normal;', '');
"If this method doesn't work, try finding the 'feature' part in the HTML data and remove it using the same approach."
Describe the bug:
When i am using certain HTML inside the widget i am getting error:
HTML to reproduce the issue:
use following widget in your code(with the data provided inside it) :
Html
widget configuration:provided above code. Expected behavior:
it should show html properly. FOR THE SAME HTML WE GET THE OUTPUT AS:
Screenshots:
Device details and Flutter/Dart/
flutter_html
versions:I am running this on chrome latest version. flutter run web
flutter doctor: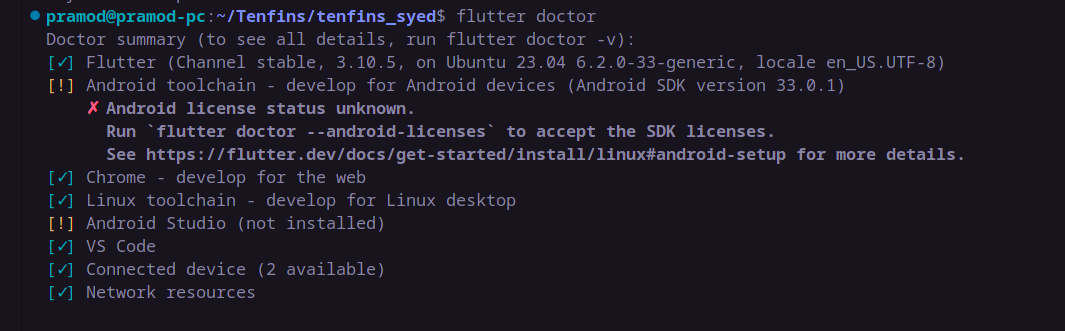
Stacktrace/Logcat
INFO: Api Call Initiated for : /getuserprofile (at xxxxxx/api/api.dart 413:13 issueRequest) ══╡ EXCEPTION CAUGHT BY WIDGETS LIBRARY ╞═══════════════════════════════════════════════════════════ The following assertion was thrown building Html(state: _HtmlState#9e569): Assertion failed: org-dartlang-sdk:///lib/ui/text.dart:77:16 feature.length == 4 "Feature tag must be exactly four characters long." The relevant error-causing widget was: Html Html:file: xxxxxx/lib/features/knowledge/knowledge_screen.dart:52:23 knowledge_screen.dart:52 When the exception was thrown, this was the stack: dart-sdk/lib/_internal/js_dev_runtime/private/ddcruntime/errors.dart 288:49 throw dart-sdk/lib/_internal/js_dev_runtime/private/ddc_runtime/errors.dart 29:3 assertFailed lib/ui/text.dart 77:34 new packages/flutter_html/src/css_parser.dart 948:42 expressionToFontFeatureSettings packages/flutter_html/src/css_parser.dart 318:33
dart-sdk/lib/_internal/js_dev_runtime/private/linked_hash_map.dart 21:13 forEach
packages/flutter_html/src/css_parser.dart 13:15 declarationsToStyle
packages/flutter_html/src/css_parser.dart 671:12 inlineCssToStyle
packages/flutter_html/src/html_parser.dart 309:11 [_styleTreeRecursive]
3
packages/flutter_html/src/html_parser.dart 325:7 [_styleTreeRecursive]
packages/flutter_html/src/html_parser.dart 294:5 styleTree
packages/flutter_html/src/html_parser.dart 166:5 prepareTree
packages/flutter_html/src/html_parser.dart 156:5 didChangeDependencies
packages/flutter/src/widgets/framework.dart 5237:11 [_firstBuild]
packages/flutter/src/widgets/framework.dart 5062:5 mount
packages/flutter/src/widgets/framework.dart 3971:15 inflateWidget
packages/flutter/src/widgets/framework.dart 3708:18 updateChild
Additional info:
A picture of a cute animal (not mandatory but encouraged)