Closed devbizzy closed 3 years ago
Check the logic of your commands/events. Maybe there's lacking exit condition and so it loops forever I've expirienced this before and it was a problem by my side.
Can you send me what commands did you typed and show me the code of them?
` const commaNumber = require('comma-number'); const humanizeDuration = require('humanize-duration'); const { MessageFlags } = require('discord.js');
module.exports = { name: 'play', aliases: ['p', 'stop', 'dc', 'song', 'np', 'skip', 'fs', 'clear', 'queue', 'q', 'loop'], description: 'Plays Music, just ask it whatever you want to play (links, name of song)', async execute(message, args, cmd, client, Discord, player) {
//Checking for the voicechannel and permissions.
const voice_channel = message.member.voice.channel;
if (!voice_channel) {
message.channel.send('Get in a vc first');
//Add auto joining once the author joins a VC in the future
return;
}
const permissions = voice_channel.permissionsFor(message.client.user);
if (!permissions.has('CONNECT')) return message.channel.send('You dont have the correct permissins');
if (!permissions.has('SPEAK')) return message.channel.send('You dont have the correct permissins');
let alreadySent = false;
client.player
.on('songAdd', (message, queue, song) => {
if (client.player.isPlaying) {
message.channel.send(`**${song.name}** has been added to the queue!`);
}
})
.on('songFirst', (message, song) => {
message.channel.send(`**${song.name}** is now playing!`);
})
.on('playlistAdd', (message, queue, playlist) => {
message.channel.send(`**${playlist.name}** playlist with **${playlist.videoCount}** songs has been added to the queue!`);
})
.on('queueEnd', (message) => {
message.channel.send(`The queue ended, nothing more to play!`)
})
.on('channelEmpty', (message, queue) => {
message.channel.send(`The **${queue.connection.channel}** was empty, music was removed!`)
})
.on('songChanged', (message, newSong, oldSong) => {
message.channel.send(`**${newSong.name}** is now playing!`)
})
.on('clientDisconnect', (message, queue) => {
if (!alreadySent) {
message.channel.send(`I got disconnected from the channel, music was removed!`)
} else return;
})
.on('error', (message, error) => {
switch (error) {
// Thrown when the YouTube search could not find any song with that query.
case 'SearchIsNull':
message.channel.send(`No song with that query was found.`);
break;
// Thrown when the provided YouTube Playlist could not be found.
case 'InvalidPlaylist':
message.channel.send(`No Playlist was found with that link.`);
break;
// Thrown when the provided Spotify Song could not be found.
case 'InvalidSpotify':
message.channel.send(`No Spotify Song was found with that link.`);
break;
// Thrown when the Guild Queue does not exist (no music is playing).
case 'QueueIsNull':
message.channel.send(`There is no music playing right now.`);
break;
// Thrown when the Members is not in a VoiceChannel.
case 'VoiceChannelTypeInvalid':
message.channel.send(`You need to be in a Voice Channel to play music.`);
break;
// Thrown when the current playing song was an live transmission (that is unsupported).
case 'LiveUnsupported':
message.channel.send(`We do not support YouTube Livestreams.`);
break;
// Thrown when the current playing song was unavailable.
case 'VideoUnavailable':
message.channel.send(`Something went wrong while playing the current song, skipping...`);
break;
// Thrown when provided argument was Not A Number.
case 'NotANumber':
message.channel.send(`The provided argument was Not A Number.`);
break;
// Thrown when the first method argument was not a Discord Message object.
case 'MessageTypeInvalid':
message.channel.send(`The Message object was not provided.`);
break;
// Thrown when the Guild Queue does not exist (no music is playing).
default:
message.channel.send(`**Unknown Error Ocurred:** ${error}`);
break;
}
});
if (cmd == 'play' || cmd == 'p') {
try {
if (client.player.isPlaying(message)) {
if (args.join(' ').indexOf('playlist') > -1 && args.join(' ').indexOf('spotify') > -1) {
let sliceNum = args.join(' ').search(/playlist\//i);
let spotifyPlaylistCode = args.join(' ').substring((sliceNum + 9))
await client.player.playlist(message, {
search: `https://open.spotify.com/playlist/${spotifyPlaylistCode}`,
maxSongs: -1,
})
} else {
let song = await client.player.addToQueue(message, args.join(' '));
}
} else {
try {
if (args.join(' ').indexOf('playlist') > -1 && args.join(' ').indexOf('spotify') > -1) {
let sliceNum = args.join(' ').search(/playlist\//i);
let spotifyPlaylistCode = args.join(' ').substring((sliceNum + 9))
await client.player.playlist(message, {
search: `https://open.spotify.com/playlist/${spotifyPlaylistCode}`,
maxSongs: -1,
})
} else {
await client.player.play(message, args.join(' '));
}
} catch (error) {
console.log(error)
}
}
} catch (error) {
console.log(error)
}
}
if (cmd == 'np' || cmd == 'song') {
if (client.player.isPlaying(message)) {
let song = await client.player.nowPlaying(message);
if (song) {
await message.channel.send(`Current Song: **${song.name}**`);
}
} else return;
}
if (cmd == 'stop' || cmd == 'dc') {
if (client.player.isPlaying(message)) {
let isDone = client.player.stop(message);
if (isDone) {
message.channel.send('Music stopped, the queue was cleared!');
alreadySent = true;
}
message.guild.me.voice.channel.leave();
} else return;
}
if (cmd == 'skip' || cmd == 'fs') {
if (client.player.isPlaying(message)) {
let song = client.player.skip(message);
if (song)
message.channel.send(`${song.name} was skipped!`);
} else return message.channel.send('No music being played currently.');
}
if (cmd == 'clear') {
if (client.player.isPlaying(message)) {
let isDone = client.player.clearQueue(message);
if (isDone) {
message.channel.send('Queue was cleared!');
}
}
}
if (cmd == 'queue' || cmd == 'q') {
if (client.player.isPlaying(message)) {
let queue = client.player.getQueue(message);
if (queue) {
message.channel.send('Queue:\n' + (queue.songs.map((song, i) => {
return `${i === 0 ? 'Now Playing' : `#${i + 1}`} - ${song.name} | ${song.author}`
}).join('\n')));
}
} else return;
}
if (cmd == 'loop') {
if (client.player.isPlaying(message)) {
let toggle = client.player.toggleQueueLoop(message);
if (toggle === null) return
else if (toggle) {
message.channel.send('Looping Queue!');
} else message.channel.send('Stopped Loop.');
}
} else return;
}
}`
i've sent my full play.js file if thats what you meant @SushiBtw
Here is another example
This is also in Heroku Console, if its helpful. I'm going to try see if I can figure out how to set the maxListeners
Check the logic of your commands/events. Maybe there's lacking exit condition and so it loops forever I've expirienced this before and it was a problem by my side.
I've checked my commands, they seem fine to me, in the events though I don't know much that I can do, thats why I asked here but ill try testing out different things.
Can you show me the commands?
the commands?
what do you mean? I've sent the full code for the commands up above
Can you show me the commands?
or do you mean like a screen shot? i've also sent that aswell
Oh shoot, my bad!
Player#on method
should be called in the index file once, not after every command.
Oh shoot, my bad!
Player#on method
should be called in the index file once, not after every command.
that has fixed it!! thank you so much :)
Bug Description Sends multiple messages of the command. Reproduction Steps Steps to reproduce the behavior:
Expected Result I expected it to just send one message, not multiple. Attachments If applicable, add screenshots to help explain your problem.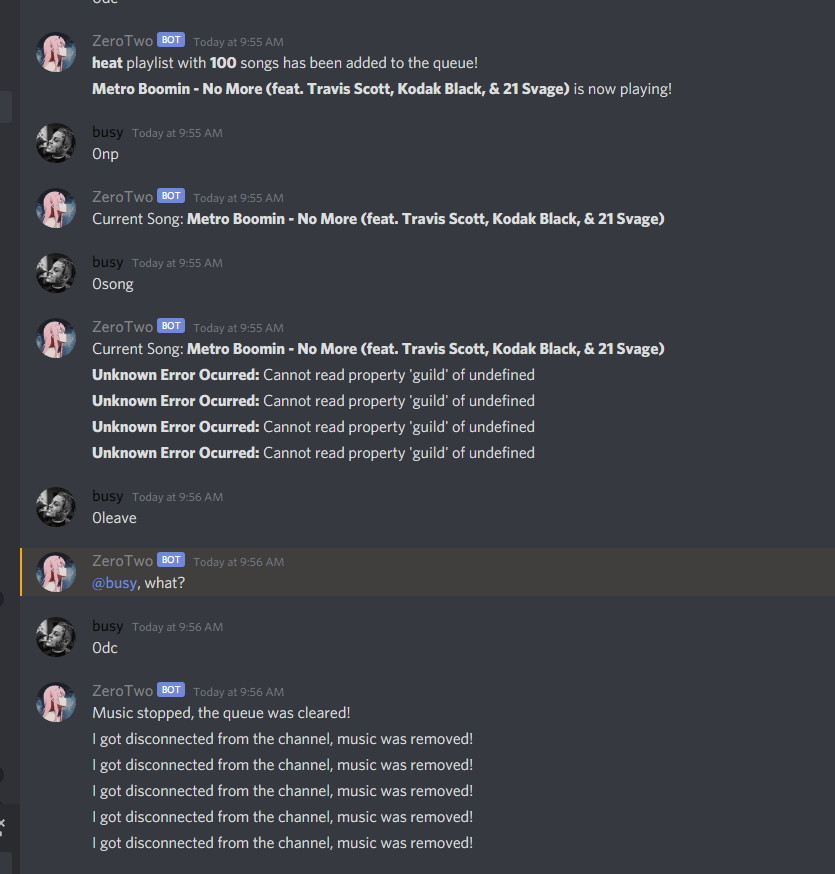
Versioning:
Additional Comments The Unknown Error Occurred: Cannot read property 'guild' of undefined. also happens but I didnt mention it because it has already been mentioned in a previous issue here