Closed wizzar closed 4 years ago
Will take a look at this
Could you explain the issue a little bit more? Not sure that I'm following. Issue is that scroll remains on same position even after you call setState?
TL;DR; Set a random unique "key" on InfiniteList so that the scroll offset is reset everytime.
Ok, I finally figured it out. The thing here is that the ScrollController saves it's scroll position in the PageStorage of the list it is attached to. So, what is happening, is that the list is rebuilt, but now the new month is at offset 0, but the scroll position is maintained, which throws it off. What must be done here is reset the list scroll offset when it is rebuilt, in my case. The way to do this here, is to set a different key to the list when it is created. This way the widget will not maintain the offset, and everything will work.
This question on SO discusses this well too: https://stackoverflow.com/questions/56364950/listview-doesnt-scroll-to-the-correct-offset-position-after-its-built-again#56365266
Initially, the InfiniteList builder is called with indexes -1, 0 and 1, and the displayed item is the 0, naturally. However, after using setState() inside the InfiniteList items, causing a rebuild, the list item being displayed first is not 0 anymore, it is always the last one, and this gets progressively worse if you traverse the scroll too much before tapping.
To reproduce: click on any date on the first month (should be december at the time of the writing). You will see that the text at the top changes, and the scroll behaves as expected. Now scroll further, to month 2, 3, etc, and click on a day. You will see that the scroll changes it offset weirdly, even thou the date computation is correct.
Upon running the app: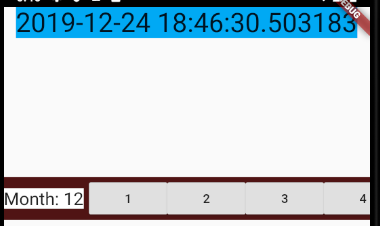
After clicking on Month 12, day 15: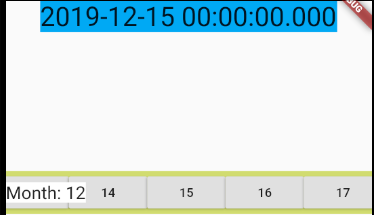
After clicking on Month 1, day 8: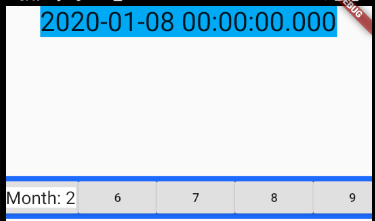
I don't understand why month 2 is being rendered first here, since month 1 is still my first item (index 0 if you will).
This is a bit complex to explain, but this code does exactly that: