Open Jez-Carter opened 1 year ago
Hi @Jez-Carter, thanks for digging into this. Sorry, I have not had time in the last month to dig into your previous issue #9. I think you are not missing anything; that dh code was actually developed prior to the CFM getting melt physics (i.e., it is pretty old!). If you want, you can dive into the code and try to fix the issue and make a pull request, or hopefully I can find a moment later this week or next week to take a look.
Hi @maximusjstevens, thanks for offering to take a look, I think that would be best and to get your further thoughts. I'll also get in contact with Vincent as I believe he's worked with cumulative elevation before. I won't be able to spend much time digging into the code myself over the next couple of weeks as I have another deadline approaching for a different piece of work but will keep you updated if I am able to.
Hello everyone, Jezz, I hard-coded my own variable for computing elevation changes. The CFM structure has changed since then, so I cannot simply push my (very) old code. However, I am happy to look into that in the coming days. I can probably work on this aspect in the coming days (or early next week). Jezz, I will email you to get all your input files.
I think that I fixed the problem. See the email I just sent to Max and you.
I've noticed some odd behaviour with cumulative elevation. That is cumulative elevation during the reference spin-up phase does not flatten (e.g. I would expect eventually cumulative elevation would stop changing as the state reaches an equilibrium). To show this behaviour I've created the following example script, where I've used the example csvs for the Summit station and have extended the spinup time even further to emphasise the result. Note, since the average snowfall is 0.23 mIeq/yr it's expected the number of years required to completely refresh the column is 120/0.23=522 (120m is the domain depth).
The result of running the above script is the following, where it can be seen no steady state is reached.
The equation for elevation change is given in firn_density_no_spin.py as:
This doesn't make complete sense to me as it feels like melt is being counted twice since (a similar issue as mentioned in issue #9), since self.sdz_new is labelled as including the impact of melt:
I tried adjusting the code to leave out self.dh_melt from the above:
Doing this I get the following result, where minimal difference is made since Summit receives minimal melt: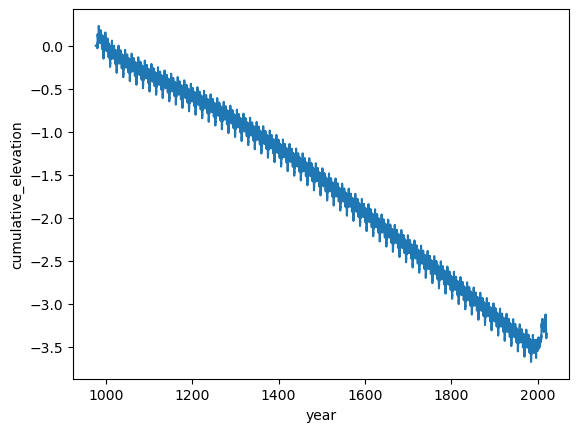
I've also tried replacing the above with a commented out line in the original code, which seems like it should work:
This does seem to result in a steady state reached at around 500 years: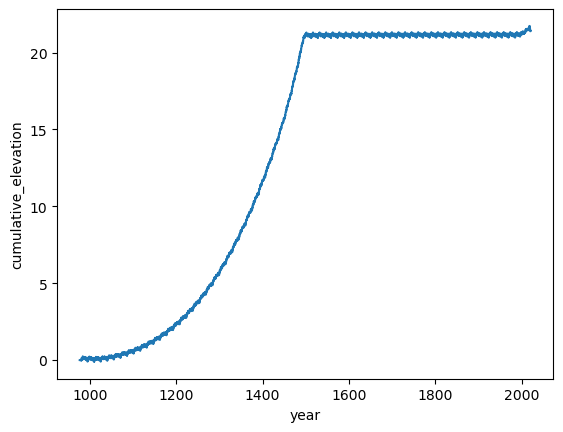
Although, this doesn't seem to work for high-melt sites, which I've simulated using the following example adjustment to the input data:
The above adjustment produces the following, which while it reaches an equilibrium shows no step change at the year 1452 where melt goes from approximately 2 to 5 m iEq/yr.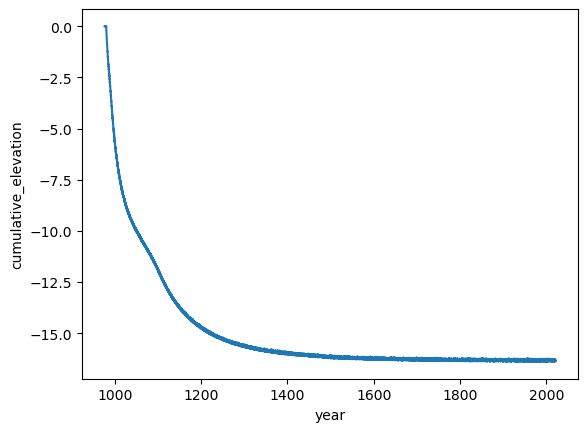
Am I missing something in all the above or is there an issue with the calculation of cumulative elevation? Best, Jez