Closed edrewitz closed 2 years ago
What you're seeing is IPython's matplotlib integration, which automatically displays any figures newly created within a cell. This is separate from you asking for the animation to be rendered to html with the last anim
line in your cell. This StackOverflow question gives a variety of approaches to disable showing the figure. I was personally able to get the figure to not show by adding plt.ioff()
before creating the ArtistAnimation
.
What went wrong?
Hi! I was following the MetPy Monday Radar Animation video exactly but it added a random blank subplot below the animator buttons. I'm not sure why this is as in my code I only created one subplot. Please see attached screenshot. Thanks!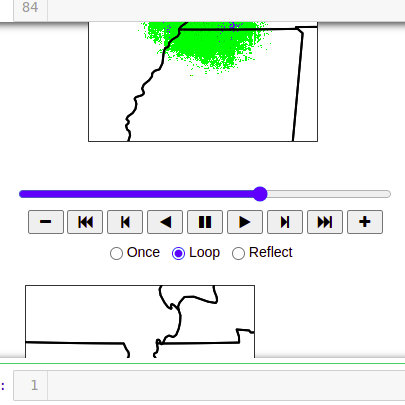
Operating System
Linux
Version
metpy 1.3.0
Python Version
Python 3.9.12
Code to Reproduce
Errors, Traceback, and Logs
No response