Closed josepdecid closed 3 years ago
This bug is caused due to state.images
in useEffect dependency array
// inside Annotator/index.js line:165
useEffect(() => {
if (selectedImage === undefined) return
console.log('Inside Select Image useEffect');
dispatchToReducer({
type: "SELECT_IMAGE",
imageIndex: selectedImage,
image: state.images[selectedImage],
})
}, [selectedImage , state.images])
so what we can do?
use selectedImage
prop and write your own custom Next and Prev function like this
const [images, setImages] = useState( /** your images (array) will comes come here */ )
const [selectedImage, setSelectedImage] = useState(0)
const handleNext = ()=>{
if ( selectedImage === images.length-1)
return
setSelectedImage(selectedImage + 1)
}
const handlePrev = ()=>{
if (selectedImage === 0)
return
setSelectedImage(selectedImage - 1)
}
. . . .
<ReactImageAnnotate
selectedImage={selectedImage}
onNextImage={handleNext}
onPrevImage={handlePrev}
images={images}
. . . .
Great, this works now, but there should be a way to have this already working without overwriting the handlers, as the functionality is a pretty common and straightforward one.
When using the
prev
andnext
buttons to change between images, the index remains the first. It changes for a moment to Image 2 but it goes back to the first one without showing any error. I'm trying to render it inside the main App component.I'm using Node v12.18.0 on Windows with the following library versions:
I attach here a GIF showing the problem: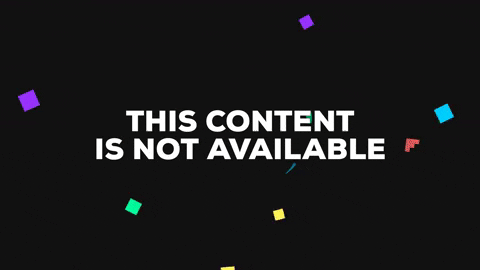
You can see that there are no errors but sometimes the following warning is raised:
I'm wondering what can cause the problem or how can I fix it.