Open feifei-Liu opened 2 years ago
To test in the overlap region, I suggest you modify the code of the official evluation code here: https://github.com/nutonomy/nuscenes-devkit/blob/master/python-sdk/nuscenes/eval/detection/evaluate.py#L98
You should add some code here to filter out boxes.
Some sample code to test if a box is repeated in several images.
from nuscenes.eval.detection.data_classes import DetectionBox
from nuscenes.utils.geometry_utils import view_points, box_in_image, BoxVisibility
from pyquaternion import Quaternion
from nuscenes.utils.data_classes import Box
import numpy as np
from copy import deepcopy
def box_center_in_image(box, cam_intrinsic, imsize, vis_level=None):
center3d = box.center
center3d = center3d[:, np.newaxis]
center_img = view_points(center3d, cam_intrinsic, normalize=True)[:2, :]
visible = np.logical_and(center_img[0, :] > 0, center_img[0, :] < imsize[0])
visible = np.logical_and(visible, center_img[1, :] < imsize[1])
visible = np.logical_and(visible, center_img[1, :] > 0)
visible = np.logical_and(visible, center3d[2, :] > 1)
in_front = center3d[2, :] > 0.1
return all(visible) and all(in_front)
def is_box_repeated(nusc, box: DetectionBox):
cams = ['CAM_FRONT_LEFT', 'CAM_FRONT', 'CAM_FRONT_RIGHT','CAM_BACK_LEFT', 'CAM_BACK', 'CAM_BACK_RIGHT']
box_vis_level = BoxVisibility.ANY
sample_token = box.sample_token
rec = nusc.get('sample', sample_token)
if isinstance(box, DetectionBox):
box = Box(box.translation, box.size, Quaternion(box.rotation))
box_backup = deepcopy(box)
num_repeat = 0
for cam_name in cams:
box = deepcopy(box_backup)
cam_token = rec['data'][cam_name]
sample_data_token = cam_token
sd_record = nusc.get('sample_data', sample_data_token)
cs_record = nusc.get('calibrated_sensor', sd_record['calibrated_sensor_token'])
sensor_record = nusc.get('sensor', cs_record['sensor_token'])
pose_record = nusc.get('ego_pose', sd_record['ego_pose_token'])
# move box to ego vehicle coord system
box.translate(-np.array(pose_record['translation']))
box.rotate(Quaternion(pose_record['rotation']).inverse)
# Move box to sensor coord system.
box.translate(-np.array(cs_record['translation']))
box.rotate(Quaternion(cs_record['rotation']).inverse)
cam_intrinsic = np.array(cs_record['camera_intrinsic'])
imsize = (sd_record['width'], sd_record['height'])
#if box_in_image(box, cam_intrinsic, imsize, vis_level=box_vis_level):
if box_center_in_image(box, cam_intrinsic, imsize, vis_level=box_vis_level):
num_repeat += 1
#assert num_repeat > 0, 'box never occur on any images!'
if num_repeat > 1:
return True
return False
seems similar to function filter_eval_boxes_by_overlap in script projects/mmdet3d_plugin/datasets/nuscnes_eval.py
The paper compares FCOS3D and Detr3D in the overlap region. How to test the nuscenes only in the overlap region?Thank you very much!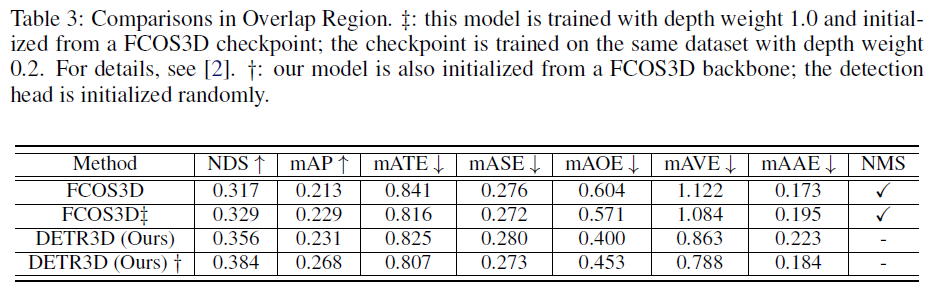