Open Swearinbag opened 2 years ago
How can you get the files which were labeled in detect.py?
I used a global variable to accumulate everything into a list of detections. I then added the files by appending it when the loop goes over the files.
Could you specify what issue that you have? sorry for the delayed reply!
Have you checked your bbox format is cxcywh or xyxy or another format?
I have indeed checked the format before- but I've actually did it another way. I finished my work with YOLOR atm, so this thread can be closed if Weitsung50110 doesn't reply soon.
I've been trying to implement a correct confusion matrix with a selfmade IoU calculation and such but, since I'm doing the same your algorithm does in utils.general.box_iou, I'm still getting different results when comparing the end result of what the script prints when detect.py is run. The following is how I do it, after I've converted the tensor variables to just a numpy array and feed them one by one in the following function:
Then I use this function in the following function to create my confusion matrix:
With this function I then use preds,labs,names,IoUtresh,conftresh from the variables in detects.py and the exclude is just so the values that don't fit the treshold are not printed (for comparison with every label vs only confident labels). I call the function above as seen below, with detects and labs being predicitons and labels respectively, converted to a list of lists.
The result when printing this confusion matrix is the following: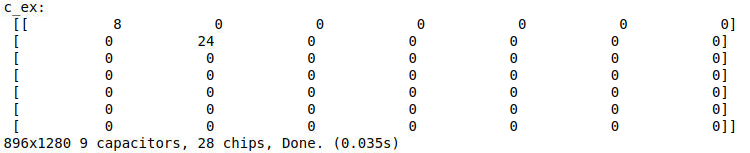
It's clear that some classes seemingly disappear in my confusion matrix, but I have no idea why. I'm seemingly following the correct (?) steps. Can someone assist me? :)
ps: structure of classes is the following: [capacitor,chips,crystal,diode,inductor,resistor,transistor]