Closed sehqlr closed 2 years ago
Hey y'all, I was wondering if there was anything that I can do, or more information that I could provide, to help in solving this issue. Thanks!
I left a comment in https://github.com/JedWatson/react-select/issues/4893#issuecomment-1007551596 which might help – I ran into the same issue and found that I needed to add react
and react-dom
to my Webpack configuration's externals
option as described in this article:
const webpackConfig = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'build'),
filename: 'myplugin.build.js',
libraryTarget: 'window',
},
externals: {
'react': 'React',
'react-dom': 'ReactDOM',
},
};
Given the solution that @montchr provided and the lack of recent activity, I am going to close this issue. If anyone feels it needs to be reopened, feel free to do so.
Description
I will begin by saying that I've been hitting my head against the wall on this, and I hope that I can get some help on this. I've opened up a support forum topic here and JedWatson/react-select#4893 there. I've gotten some help on IRC as well. But I've come to the conclusion that I need to open up this bug report as well.
My hypothesis is that there's a bug in Gutenberg that is triggered by a custom block using react-select's AsyncSelect for a dropdown within the InspectorControls component. This error only occurs when the Gutenberg plugin is active. It seems to be directly related to the upgrade to React 17. I don't want to get bit by this bug when Gutenberg core is upgraded to React 17, which is why I've been working on this for weeks now.
When the bug does trigger, the React error I get is this: https://reactjs.org/docs/error-decoder.html/?invariant=321 I've explored all of the possibilities, and I think it might be the multiple copies of React one? But if I knew for sure, I wouldn't be opening this issue.
Step-by-step reproduction instructions
Unfortunately, there isn't an easy way for y'all to reproduce this bug, since the site I'm working on uses a custom theme and plugin, and both are proprietary. Hopefully the screenshots and snippets below explain this enough to diagnose.
Screenshots, screen recording, code snippet
Screenshots
This is what the editor looks like before the bug triggers. This is taken from my local dev env.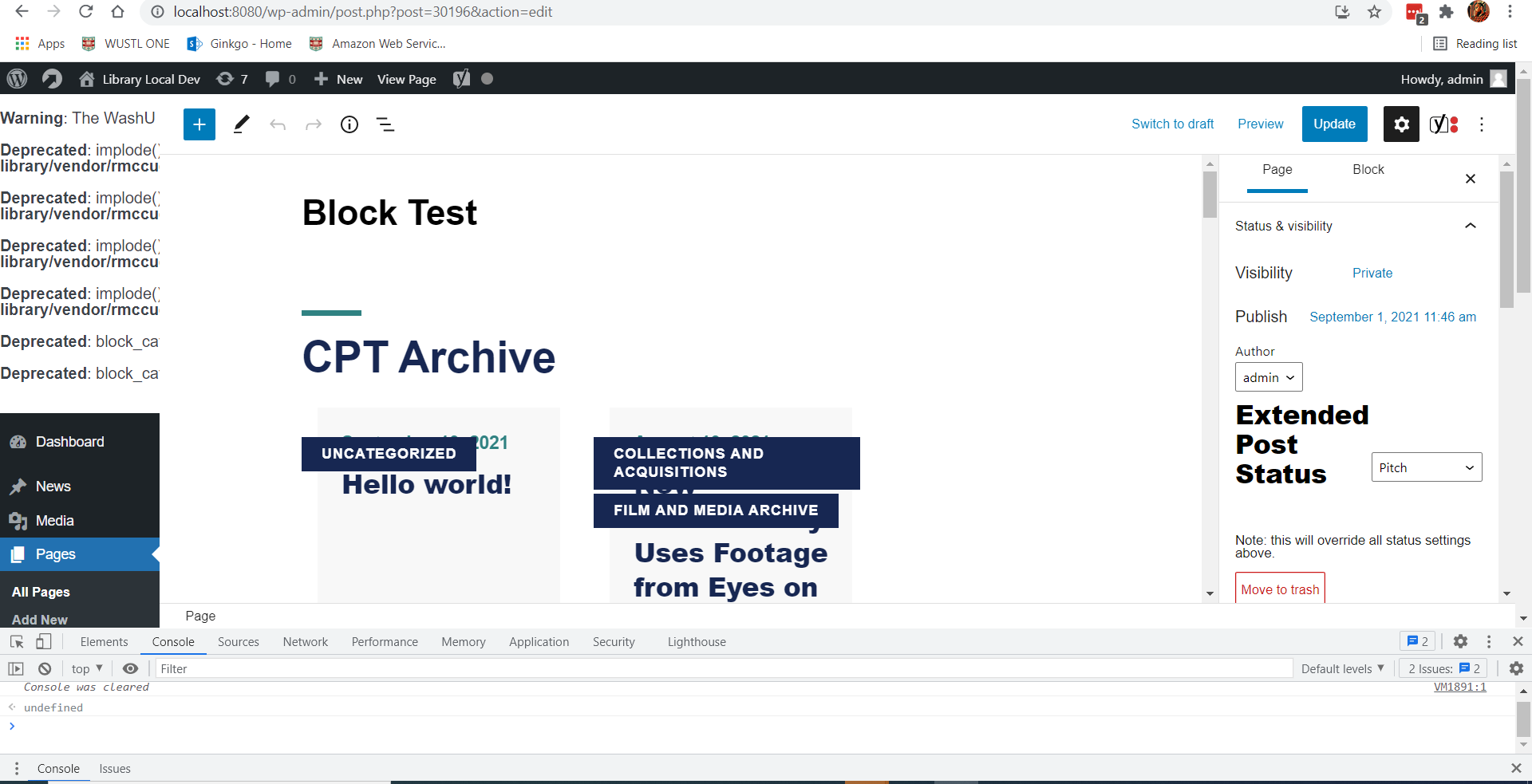
This is what the editor looks like after you click on the CPT Archive custom block. This is production, with Gutenberg plugin deactivated.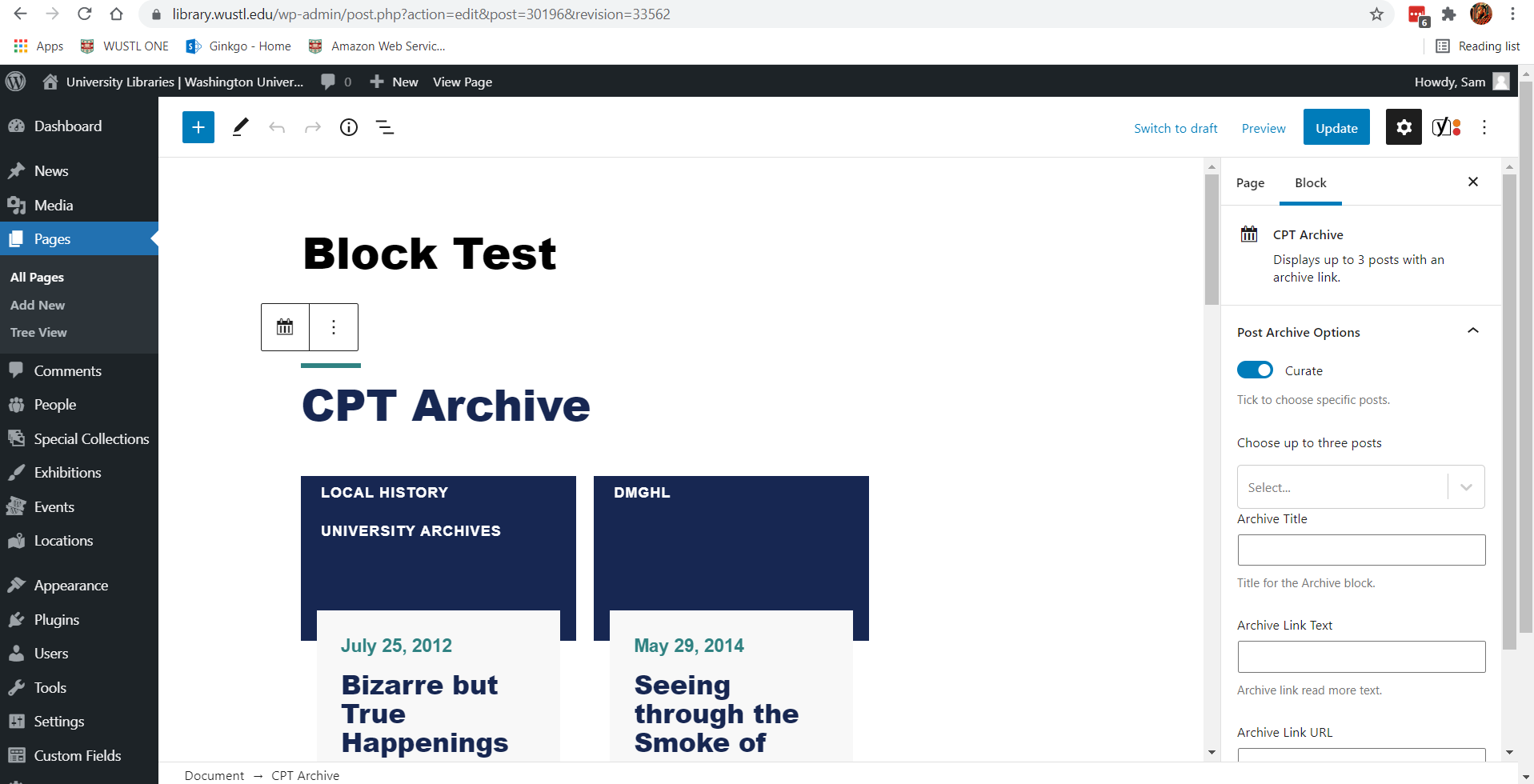
This is what happens when the Gutenberg plugin is active and you click on the block.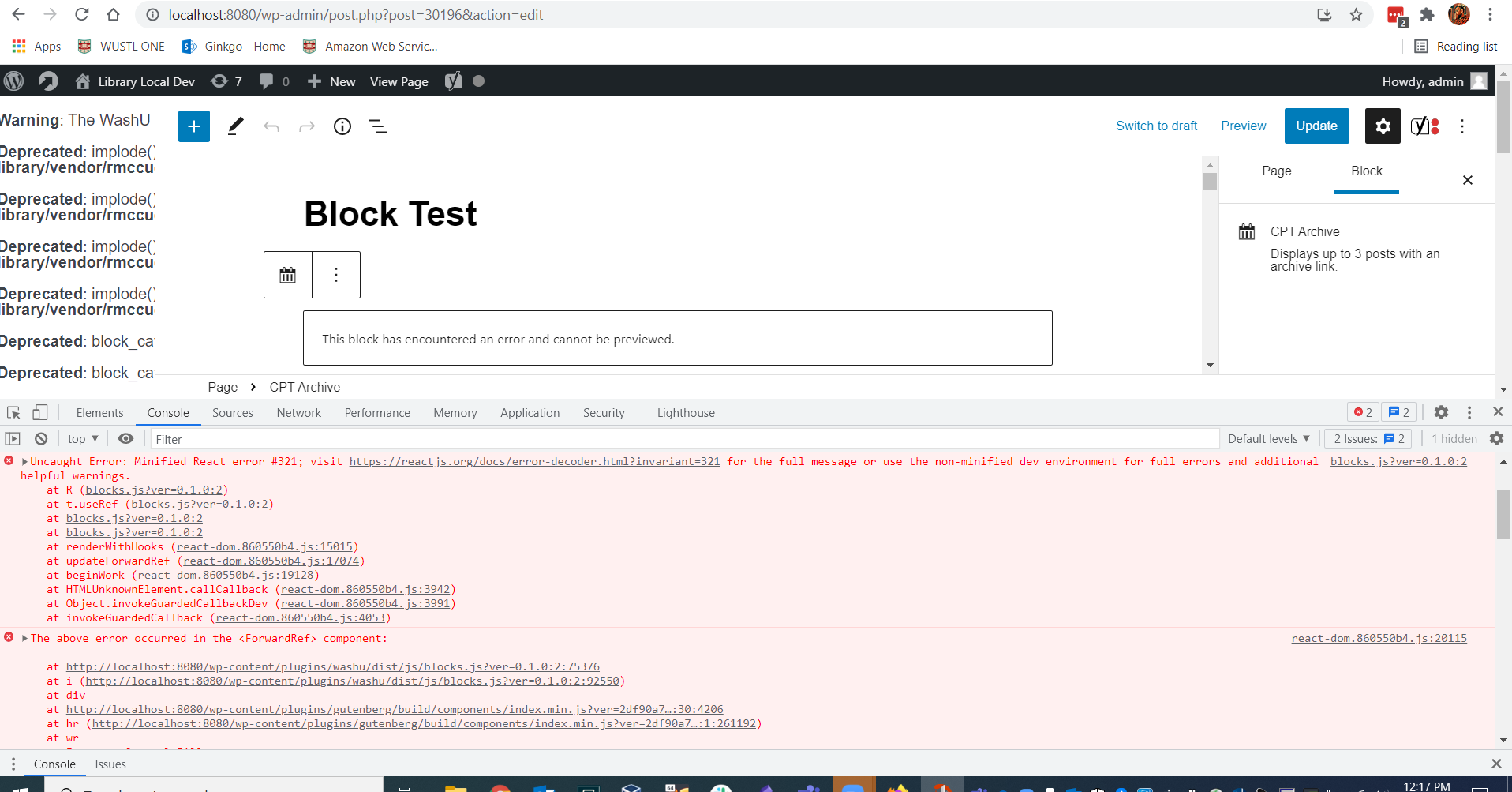
Snippets
Like I said, I can't share everything since it's proprietary, but I will share two relevant files:
Environment info
Wordpress version: 5.8 Gutenberg version: 11.6 Custom theme and plugin are both version 0.1
Please confirm that you have searched existing issues in the repo.
Yes
Please confirm that you have tested with all plugins deactivated except Gutenberg.
Yes