Closed woophi closed 3 years ago
I recommend not using this in dev mode where you want/need fast compilation, 6 seconds is brutal enough.
I have no strong intentions to make this plugin faster because I recommend it to be used to trigge errors in CI and if the duration is 12 seconds in CI, that's not important enough to micro-optimize.
Is it possible to make this plugin to run in separate thread, similar with the ForkTsCheckerWebpackPlugin?
up
Upgrading to latest circular-dependency-plugin causes performance degradation on recompile in dev mode.
with plugin ~12s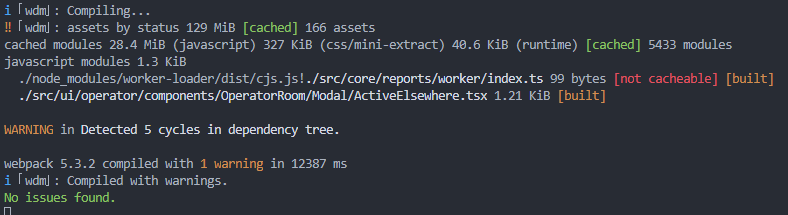
without plugin ~2.5s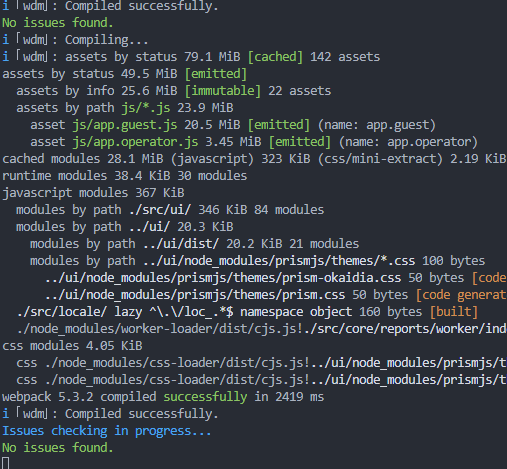
current webpack configuration:
in comparison with old versions of webpack and this plugin, recompile tooks ~6s "webpack": "^4.44.2" and "circular-dependency-plugin": "^5.2.0",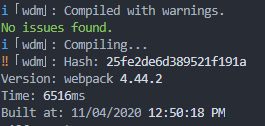
package.json