Closed piechoo closed 3 years ago
The problem is you're pushing the same event object 100 times in the event array. You need to create individual objects for each event. Correct would be:
const myEvents = [];
for (let i = 0; i < 100; i++) {
myEvents.push({
start: '2021-09-02T09:00',
end: '2021-09-02T18:00',
title: 'Business of Software Conference',
color: '#ff6d42',
});
}
Hi, I am facing some issue when I have many events in one day. Problem is visible in callendar view
Changing view:
Changing view again:
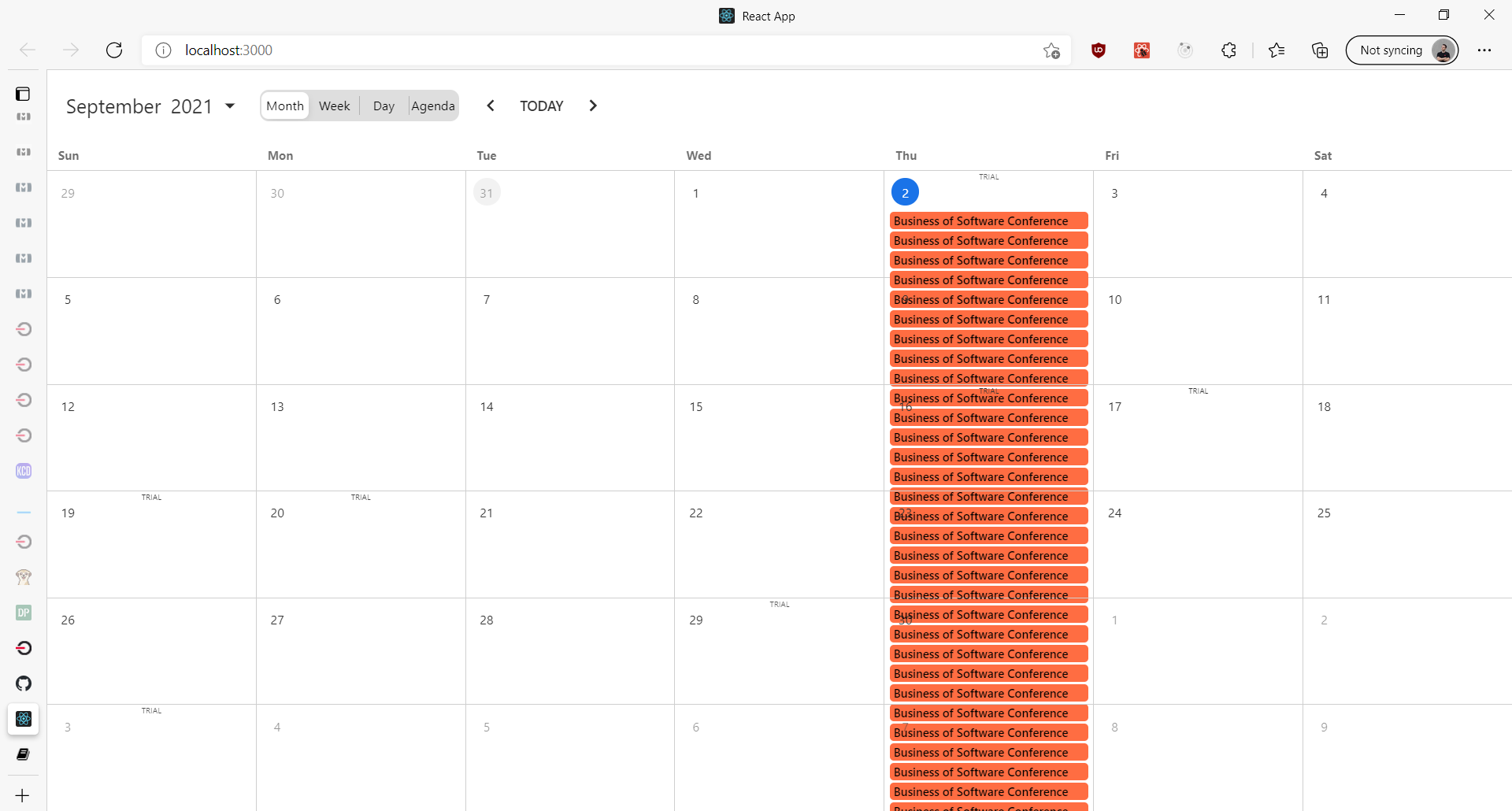
calendar: { type: 'week' }
. My default view iscalendar: { labels: true }
, I have code used in yours demo and when I change view to week callendar events overflow the day grid and when I chage the view back to month callendar, the overflow is even bigger. First render :Here is my code: