Open rpavlik opened 3 years ago
A quick adaptation of the formulas on that page:
import math
def compute_dew_point(temperature, relative_humidity):
T = temperature
RH = relative_humidity
return (
243.04
* (math.log(RH / 100.0) + ((17.625 * T) / (243.04 + T)))
/ (17.625 - math.log(RH / 100.0) - ((17.625 * T) / (243.04 + T)))
)
def compute_relative_humidity(temperature, dew_point):
TD = dew_point
T = temperature
return 100 * (
math.exp((17.625 * TD) / (243.04 + TD)) /
math.exp((17.625 * T) / (243.04 + T))
)
def apply_temp_compensation_to_relative_humidity(temperature, relative_humidity, temp_adjust):
dew_point = compute_dew_point(temperature, relative_humidity)
return compute_relative_humidity(temperature + temp_adjust, dew_point)
That adjustment appears to work for me - if I offset the temp by -14 degrees C, the relative humidity works out the same.
I've ported my air-quality code from Clue to FunHouse, and in the process looked at what the on-board AHT humidity sensor says compared to what the SCD30 says.
I'm not sure if this is just the difference due to local heating of the sensor (relative humidity drops as temp rises, even if absolute humidity stays the same), or if there's an issue in the driver or something.
Hmm, according to https://bmcnoldy.rsmas.miami.edu/Humidity.html, the AHT's measurement is a dewpoint of 11.52, vs the scd30 of 11.82, which is pretty close - so probably the FunHouse (and other) guides that apply a temperature correction should also re-compute relative humidity. Might actually want to put the dew point calculations into a library of its own.
For reference, here's my setup - they're all basically right next to each other. The SCD data matches what I would expect from my other sensors around the house, which are a mix of HTU21D and SHT30D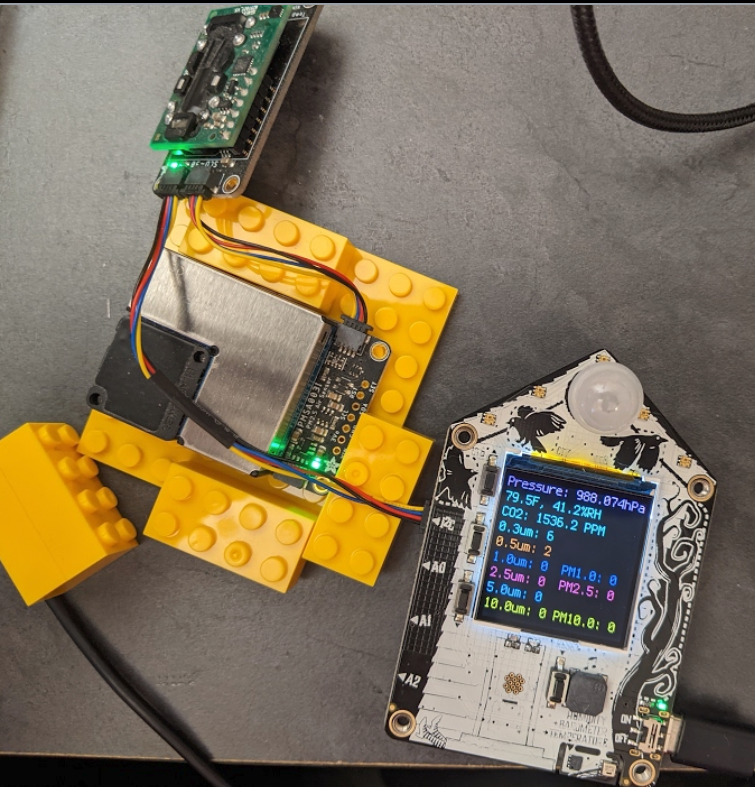