Open pmi123 opened 5 years ago
The parameter number_of_times_to_upsample
is for the face_locations()
function, not the face_encodings()
function. To use it, you need to break out face detection as a separate step. Try changing your code to this:
image_path = os.path.join(document_path, document_file_name)
image = face_recognition.load_image_file(image_path)
face_locations = face_recognition.face_locations(image, number_of_times_to_upsample=2)
face_encodings = face_recognition.face_encodings(image, known_face_locations=face_locations)
And by splitting it into two steps like this, this also gives you an array of face locations that you could save in a database (so you know which face is which)
Thanks!! I totally missed that very important point about face_locations versus face_encodings.
My code does look just like yours, and I do save the locations in the database along with the encodings to make recognizing the faces easier. I just left out those details in my first question.
Unfortunately, making both the changes you described still did not find the baby's face in image 052_002.
Any examples of how to tell face_recognition there is a missing face - something like facebook where one can click on the missing face? Or other mechanisms for pointing out a region of a missed face?
Thanks!
Mark
Instead of this:
face_locations = face_recognition.face_locations(image, number_of_times_to_upsample=2)
You could try the CNN model like this:
face_locations = face_recognition.face_locations(image, model="cnn")
It's more robust in difficult situations.
You can also pass in your own list of face_locations
to face_encodings()
, but that probably won't work very well unless the face boxes are perfect.
Unfortunately, I don't have an nvidia graphics card to make that work. At least, I assume I need one to use the CNN model. I am running on a headless machine.
Mark
You don't need a gpu. It will just run more slowly without one.
OK, I have CUDA and cudnn installed and dlib setup with them on Ubuntu 18.04. I tried
face_locations = face_recognition.face_locations(image, model="cnn")
and got this error:
File "/home/mark/.virtualenvs/memorabilia-JSON/lib/python3.6/site-packages/face_recognition/api.py", line 116, in face_locations
return [_trim_css_to_bounds(_rect_to_css(face.rect), img.shape) for face in _raw_face_locations(img, number_of_times_to_upsample, "cnn")]
File "/home/mark/.virtualenvs/memorabilia-JSON/lib/python3.6/site-packages/face_recognition/api.py", line 100, in _raw_face_locations
return cnn_face_detector(img, number_of_times_to_upsample)
MemoryError: std::bad_alloc
I have this much RAM (in GB)
total used free shared buff/cache available
Mem: 15 7 4 0 2 6
Swap: 0 0 0
Any suggestions on how to fix this would be greatly appreciated.
Mark
try passing in face_locations = face_recognition.face_locations(image, model="cnn", number_of_times_to_upsample=0)
and seeing if that fits in RAM.
That worked, and tie software found the missing baby's face! number_of_times_to_upsample=0 and number_of_times_to_upsample=1 worked. number_of_times_to_upsample=2 failed. Hmmm 16 GB of total memory is too small. Time to upgrade! Thanks for all your help! Mark
Thank you so much guys, your conversation works for me CNN is not working in my lappy i have 8 GB ram, i tried the HOG and it works easily. i really am finding the reason and you and i got it, Thanks again.
I'm using facerec_from_webcam_faster.py from the examples for some testing and if I change the model from hog to cnn, it doesn't recognize the face and lags hard. I'm also seeing my GPU usage happening but it's 10%-15% of the power. I'm not sure how to intelligently ask how to configure this to work with my GPU. I do have a Nvidia RTX3080. I have downloaded the CUDA drivers as well.
Description
My python program detects faces in many photographs (not from cell phone, but scanned images). The baby's face is not detected in one photo (052_002), but was detected in a similar photo (052_003) (both are attached). All the other faces were detected in both photos (including the baby in 052_003). 052_002 - baby looking at mother 052_003 - baby looking at camera
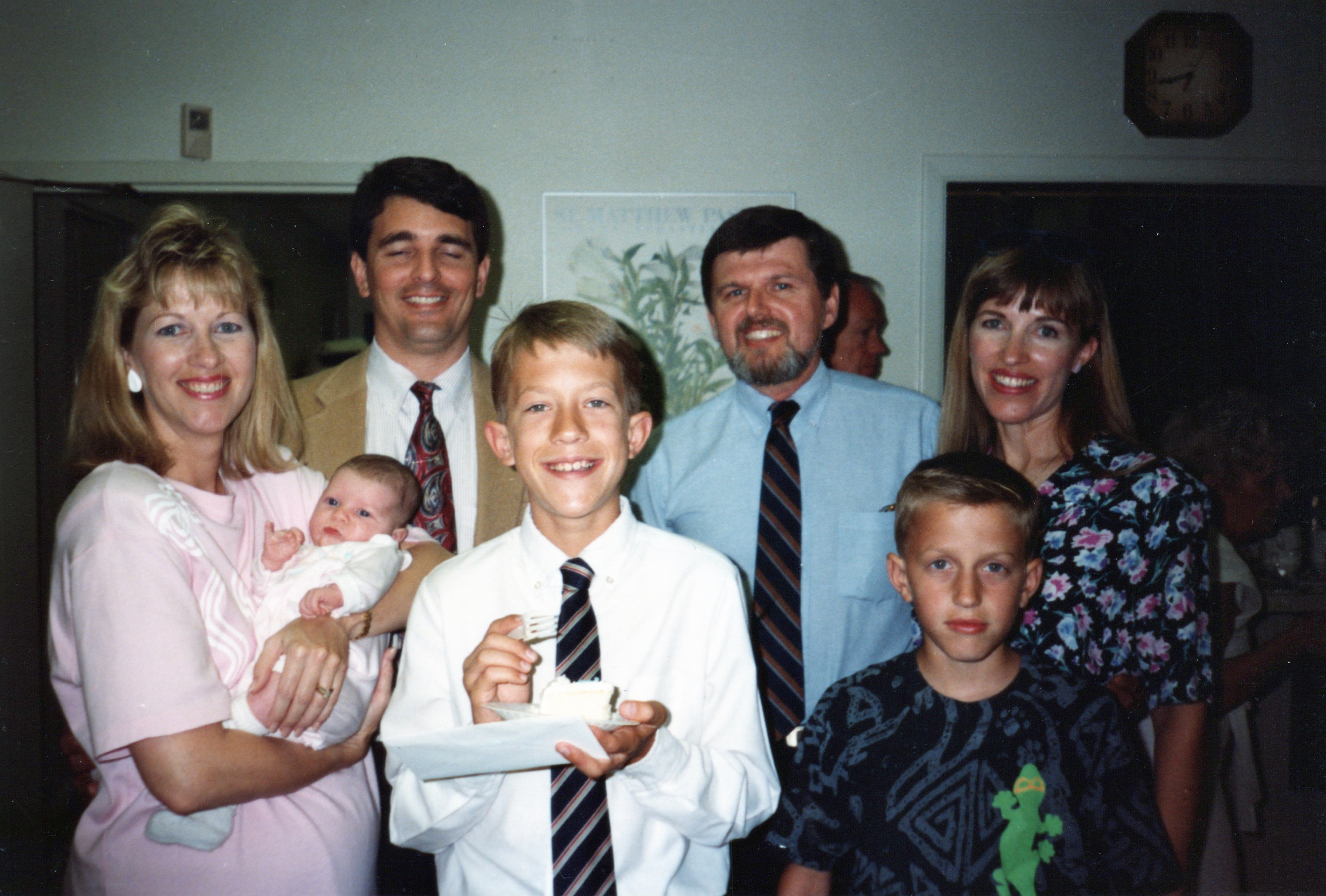
What I Did
When the baby's face in 052_002 was not found, I looked through your web site and found this article - https://github.com/ageitgey/face_recognition/wiki/Face-Detection-Problems, so I tried adding the parameter "number_of_times_to_upsample=2" in face_encodings, but got this error: face_encodings() got an unexpected keyword argument 'number_of_times_to_upsample'
image_path = os.path.join(document_path, document_file_name) image = face_recognition.load_image_file(image_path) face_encodings = face_recognition.face_encodings(image, number_of_times_to_upsample=2)
[2018-11-12 11:06:04,077: ERROR/ForkPoolWorker-1] Task biometric_identification.tasks.find_faces_task[dd9be9ee-6529-4a1c-8327-332a5b0b9532] raised unexpected: TypeError("face_encodings() got an unexpected keyword argument 'number_of_times_to_upsample'",) Traceback (most recent call last): File "/home/mark/.virtualenvs/memorabilia-JSON/lib/python3.4/site-packages/celery/app/trace.py", line 382, in trace_task R = retval = fun(*args, *kwargs) File "/home/mark/.virtualenvs/memorabilia-JSON/lib/python3.4/site-packages/celery/app/trace.py", line 641, in __protected_call__ return self.run(args, **kwargs) File "/home/mark/python-projects/memorabilia-JSON/biometric_identification/tasks.py", line 55, in find_faces_task face_encodings = face_recognition.face_encodings(image, number_of_times_to_upsample=2) TypeError: face_encodings() got an unexpected keyword argument 'number_of_times_to_upsample'
Is it possible to change the parameters for face_encodings to find this face?
Is there a way to select the face along the lines of Facebook tagging, and then get the location and encodings so I can add them to my database?
Thanks!
Mark