Closed zhijian-pro closed 2 years ago
ApplyMethod 和 ApplyMethodSeq 对于第三个参数的处理有些细节上的差异:(1)ApplyMethod第三个参数为一个匿名函数,用户可定制能力强,可以校验入参,赋值出参;(2)ApplyMethodSeq第三个参数为一个切片,这个切片元素就是make一个动态函数的返回值,而这个动态函数忽略入参和出参,仅能打桩返回值。 对于打桩的类型,建议定义为public。
有可能是你的Echo方法太短被内联了,看一下readme的第一条notes,试试
go test -gcflags=all=-l -v svc_test.go -test.run TestBizEcho
@agiledragon 能否解释下为什么很多方法都需要传一个匿名函数作为参数,我看代码中这个参数的作用是进行类型比对,事实上我更改部分代码,去掉了这部分的类型校验,单例模式的打桩就可以用了,那是不是所有的函数中的这个匿名函数参数都可以去掉那?这个类型的比对的原因是什么那?
我写了一个小 demo 模拟这个问题 首先目录结构如下图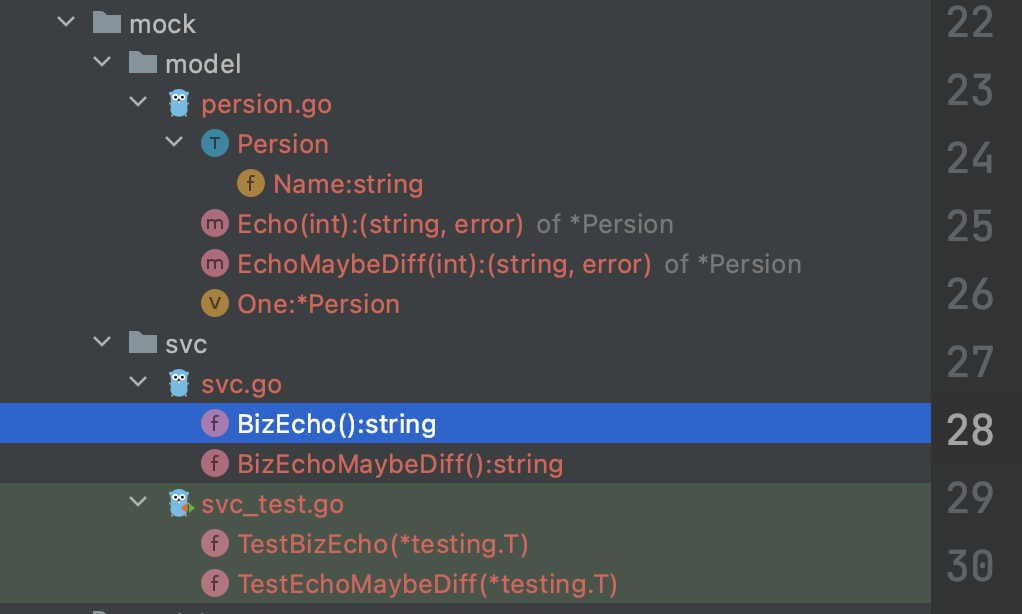
persion.go 代码如下
svc.go 代码如下
svc_test.go 代码如下
我觉得这种单例模式写法应该挺常见的,是不是我使用的姿势不对?