Open sunxirui310 opened 5 years ago
Hi, sorry for the late answer. You can use
points_transformed = al.Points.transform(points, displacement)
to transform points. Hope this could help you.
thx,i've got pytorch transform matrix -> opencv transform matrix . seems to make a detour. just 2D works.
def theta2param(theta, w,h):
if theta.shape == (2,3):
theta = np.vstack((theta,[0,0,1]))
theta = np.linalg.inv(theta)
param = np.zeros([2,3])
param[0,0] = theta[0,0]
param[0,1] = theta[0,1] / h * w
param[0,2] = (theta[0,2] - theta[0,0] - theta[0,1] + 1) * w / 2
param[1,0] = theta[1,0] / w * h
param[1,1] = theta[1,1]
param[1,2] = (theta[1,2] - theta[1,0] - theta[1,1] + 1) * h / 2
return param
Hi, sorry for the late answer. You can use
points_transformed = al.Points.transform(points, displacement)
to transform points. Hope this could help you.
Hi, Thanks for sharing!
I'm trying to find corresponding point in registration. Could you please tell me the displacement meaning in your implementation? I check the value of displacement and find values between [-0.01,0.01] in both x and y direction(in kernel_registration_2d.py). So displacement not mean pixel translation in each direction?
I'm trying this way but encounter error...
Traceback (most recent call last):
File "kernel_registration_2d.py", line 139, in
my code:
displacement = transformation.get_displacement()
warped_image = al.transformation.utils.warp_image(moving_image, displacement)
polybox = [[654, 260], [654, 290], [700, 290], [700, 260]]
warped_polybox = al.Points.transform(np.array(polybox).reshape([-1, 2]), displacement)
displacement = al.create_displacement_image_from_image(displacement, moving_image)
error:
Traceback (most recent call last):
File "/Users/yangxue/Desktop/yangxue/code/airlab/examples/demo.py", line 151, in <module>
main()
File "/Users/yangxue/Desktop/yangxue/code/airlab/examples/demo.py", line 99, in main
warped_polybox = al.Points.transform(np.array(polybox).reshape([-1, 2]), displacement)
File "/Users/yangxue/Desktop/yangxue/code/airlab/airlab/utils/points.py", line 105, in transform
raise Exception("Datatype of displacement field not supported.")
Exception: Datatype of displacement field not supported.
So I used the wrong displacement. How do I get the correct displacement? Can you give an example?
Im trying to get a point warped in moving image. something goes wrong. someone give me a hand? thx!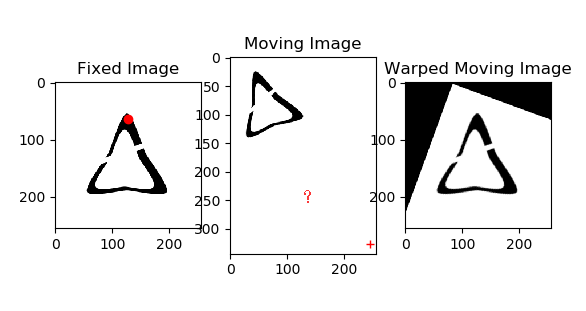
in file 'affine_registration_2d.py' i added: