Open JackinMS opened 3 years ago
Please check in vimba Viewer what is the value of DeviceLinkThroughputLimit, is it set to 380 Mbit/s. The frame rate depends on the exposure time, pixel format, DeviceLinkThroughputLimit and resolution.
Thank you for your answer! It is working now. and FPS is going little bit faster but even I fixed exposure time and resolution, it is still slow like 4 FPS. I cannot find DeviceLinkThroughputLimit in VimbaViewer 5.0. There is DeviceStatus and there are only about DeviceTemperature.
Please find the DeviceLinkThroughputLimit as in the attached screenshot below
In the above attached image Device Line Speed is the Maximum speed. Please set Device Link Throughput Limit value to the Device Line Speed to obtain higher fps.
I tried to look for the DeviceControl section on the right side but as in the attached screenshot below, my vimba viewer does not have whole section.
From the above image I see that you are using GiGE camera. For further support and process we would like to add this issue to our ticket system. I request you to write an email to support@alliedvision.com.
Hello,
I have the same problem with a Mako G-032B with only 1 FPS. The Device Status Section looks the same to me in the Viewer.
How was the solution for the tread starter ?
Thank you very much
@AxeSie I am sorry that I don't use Mako G-032B anymore..because it is difficult to handle.
@AxeSie This issue is because your Auto Exposure is on or your camera is not configured properly. Can you please write us an email to support@alliedvision.com.
import threading import sys import cv2 import timeit import time import os import tensorflow as tf import numpy as np from typing import Optional from vimba import *
WORKSPACE_PATH = '/home/kibi/Tensorflow/workspace/training_demo' SCRIPTS_PATH = 'scripts' APIMODEL_PATH = 'models' ANNOTATION_PATH = WORKSPACE_PATH+'/annotations/test/new' IMAGE_PATH = WORKSPACE_PATH+'/images' CONFIG_PATH ='/home/kibi/Tensorflow/workspace/training_demo/exported-models/my_model/test/d2/pipeline.config' CHECKPOINT_PATH ='/home/kibi/Tensorflow/workspace/training_demo/exported-models/my_model/test/d2/checkpoint'
Load pipeline config and build a detection model
configs = config_util.get_configs_from_pipeline_file(CONFIG_PATH) detection_model = model_builder.build(model_config=configs['model'], is_training=False)
Restore checkpoint
ckpt = tf.compat.v2.train.Checkpoint(model=detection_model) ckpt.restore(os.path.join(CHECKPOINT_PATH, 'ckpt-0')).expect_partial()
category_index = label_map_util.create_category_index_from_labelmap('/home/kibi/Tensorflow/workspace/training_demo/annotations/test/new/label_map.pbtxt')
def detect_fn(image): image, shapes = detection_model.preprocess(image) prediction_dict = detection_model.predict(image, shapes) detections = detection_model.postprocess(prediction_dict, shapes) return detections, prediction_dict, tf.reshape(shapes, [-1])
def parse_args() -> Optional[str]: args = sys.argv[1:] argc = len(args) for arg in args: if arg in ('/h', '-h'): print_usage() sys.exit(0)
def get_camera(camera_id: Optional[str]) -> Camera: with Vimba.get_instance() as vimba: if camera_id: try: return vimba.get_camera_by_id(camera_id) except VimbaCameraError: abort('Failed to access Camera \'{}\'. Abort.'.format(camera_id)) else: cams = vimba.get_all_cameras() if not cams: abort('No Cameras accessible. Abort.')
def setup_camera(cam: Camera): with cam:
Enable auto exposure time setting if camera supports it
class Handler: def init(self): self.shutdown_event = threading.Event()
def main(): cam_id = parse_args()
if name == 'main': main()
It works and I got the screen like :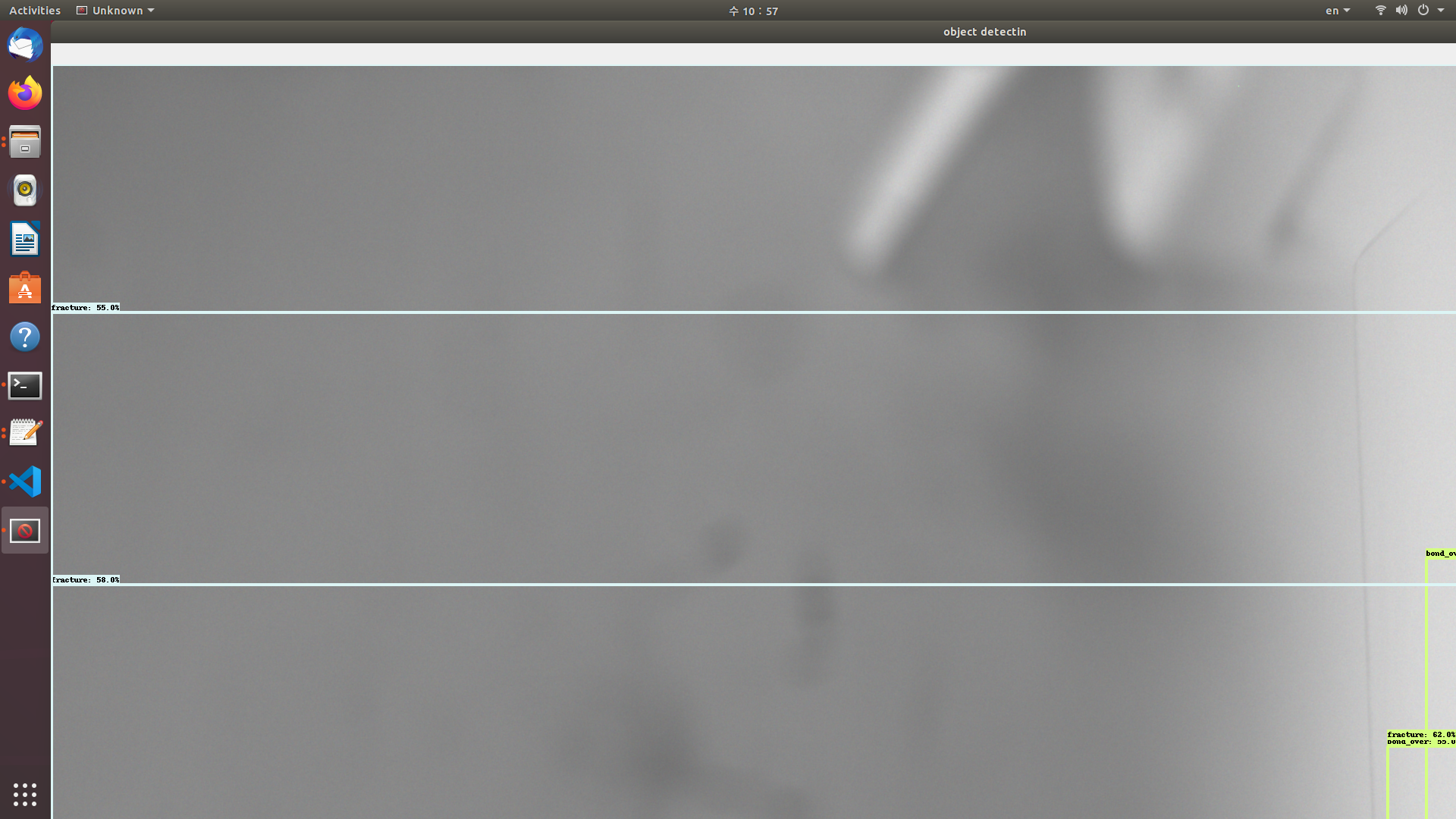
but after 10frame, it stopped and I got the error message like :
I have been tried to change the frame part but it doesn't work. Could you let me know how to handle it? Thank you.