Closed mickeyvip closed 6 years ago
Looking into this, you definitely aren't doing anything wrong, this is a bug. Thank you for the detailed bug report, I will fix this asap.
Closing this issue published version 1.1.5 to npm which should correct this bug.
That was blazingly fast! Thank you!
just published 1.1.6 to fix the regression 1.1.5 introduced, I broke root actions getting the right actions object with that fix lol... just pulled get() from hyperapp source to correct this properly this time.
@andyrj , should @hyperapp/router
show the correct route view when traveling through states?
The view doesn't change...
UPDATE: looks like the view changes only when location
changes... so it's how @hyperapp/router
works. This is the problem with time traveling debugger and side effects.
@mickeyvip when I was more active with hyperapp I had my own router that wired up location into the state and would update to correct views when time traveling. I'm not sure what changed with hyperapp/router it used to store the location in the state something like this... https://github.com/ajces/router/blob/master/src/router.js#L18
It's only a problem if you're router decides to not store the routing data in the state...
Hello.
I am toying with https://zaceno.github.io/hypercraft/post/modular-apps/ and when adding
devtools
, the module's async action gets the correct state but incorrect actions - top level actions.I might be doing something wrong, but without wrapping the
app
withdevtools
the app works fine.Here is the Chrome's DevTools. I highlighted the "Local" section where it can be seen that the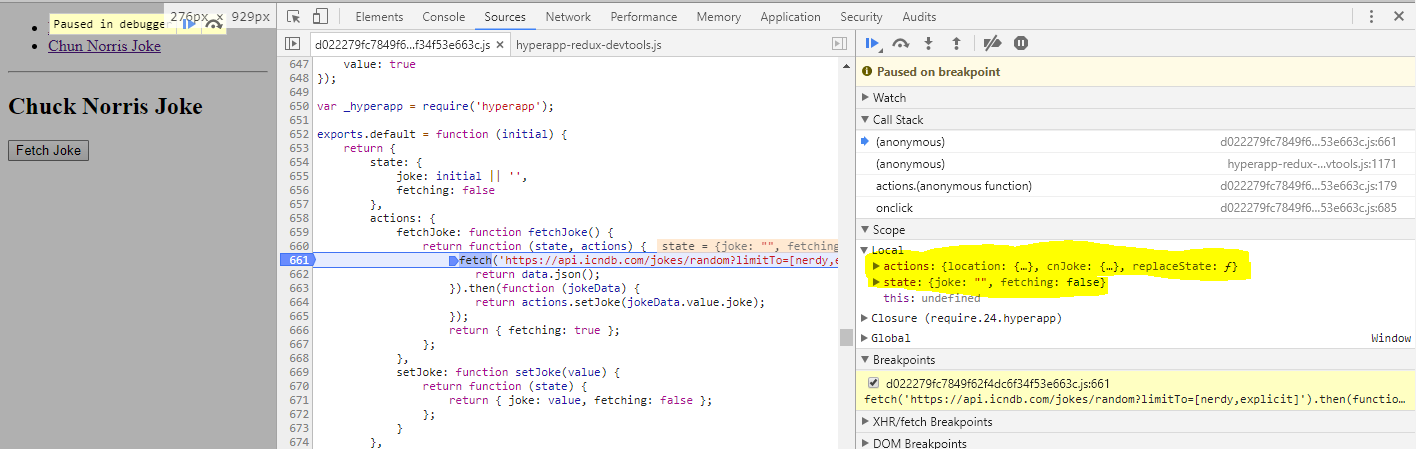
actions
are the wrong ones (top level) and the state is correct:And the exception:
Here is the code:
CNJoke.js
App.js