Open renilbabu03 opened 6 years ago
I've had a similar problem. It looks like the TransferHttpCacheModule
only works correctly for full urls. When I changed the call from this.http.get<any>('/api/blogposts')
to this.http.get
I'm not sure if it's a bug. It kind of make sense to have full urls when the server is rendering the page. But it should be documented I've spend few hours figuring that out.
Here is a simple HttpInterceptor that adds the url before API request only on server, that I now use
import { Injectable, Inject } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
import { PLATFORM_ID } from '@angular/core';
import { isPlatformServer } from '@angular/common';
@Injectable()
export class ApiHttpInterceptor implements HttpInterceptor {
constructor(@Inject(PLATFORM_ID) private platformId: Object) { }
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
if (isPlatformServer(this.platformId)) {
const url = `http://localhost:4000${req.url}`;
req = req.clone({url: url});
}
return next.handle(req);
}
}
@jandeu can you make an example repo or sth ? cause i got the same problem here .
@jandeu Thanks. I wasted my 5 hours to debug this issue.
Dynamic data is not showing up in Page Source Viewer All tags with angular code in template not showing in source viewer
I had some code which was throwing error on server side (most of which was about browser activities such as mouseover, click etc) I wrap up that code snippets in the following condition and everything worked as charm
if (isPlatformBrowser(this._platformId)) {
// Code snippets specific to browser
}
Recommended: You should always check for server status as it indicates the error
Dynamic data is not showing up in Page Source Viewer All tags with angular code in template not showing in source viewer
Have you found solution for this?
Have you found solution for this?
I had some code which was browser-specif that was causing error at server-side, so I wrapped that code-blocks in condition.
Is there any error in terminal? If yes, please provide it.
Hi Guys, any update on this?
Hi Guys, any update on this?
Hi, Can you check if there any error in the console (terminal)
@BruneXX I solved my problem this way.
I had some code which was throwing error on server side (most of which was about browser activities such as mouseover, click etc) I wrap up that code snippets in the following condition and everything worked as charm
if (isPlatformBrowser(this._platformId)) { // Code snippets specific to browser }
Recommended: You should always check for server status as it indicates the error
I have implemented Angular Universal for Angular 5. Everything has compiled well enough. The only places where I see the universal work ( html with content in view source) is in static pages i.e where no data is fetched from the server. Views having data fetched from resolve or any other means is not shown in the view source of the page. I am not quite sure what I am missing. Can you please help.
Below is the screen shot of the view source of page having data from server.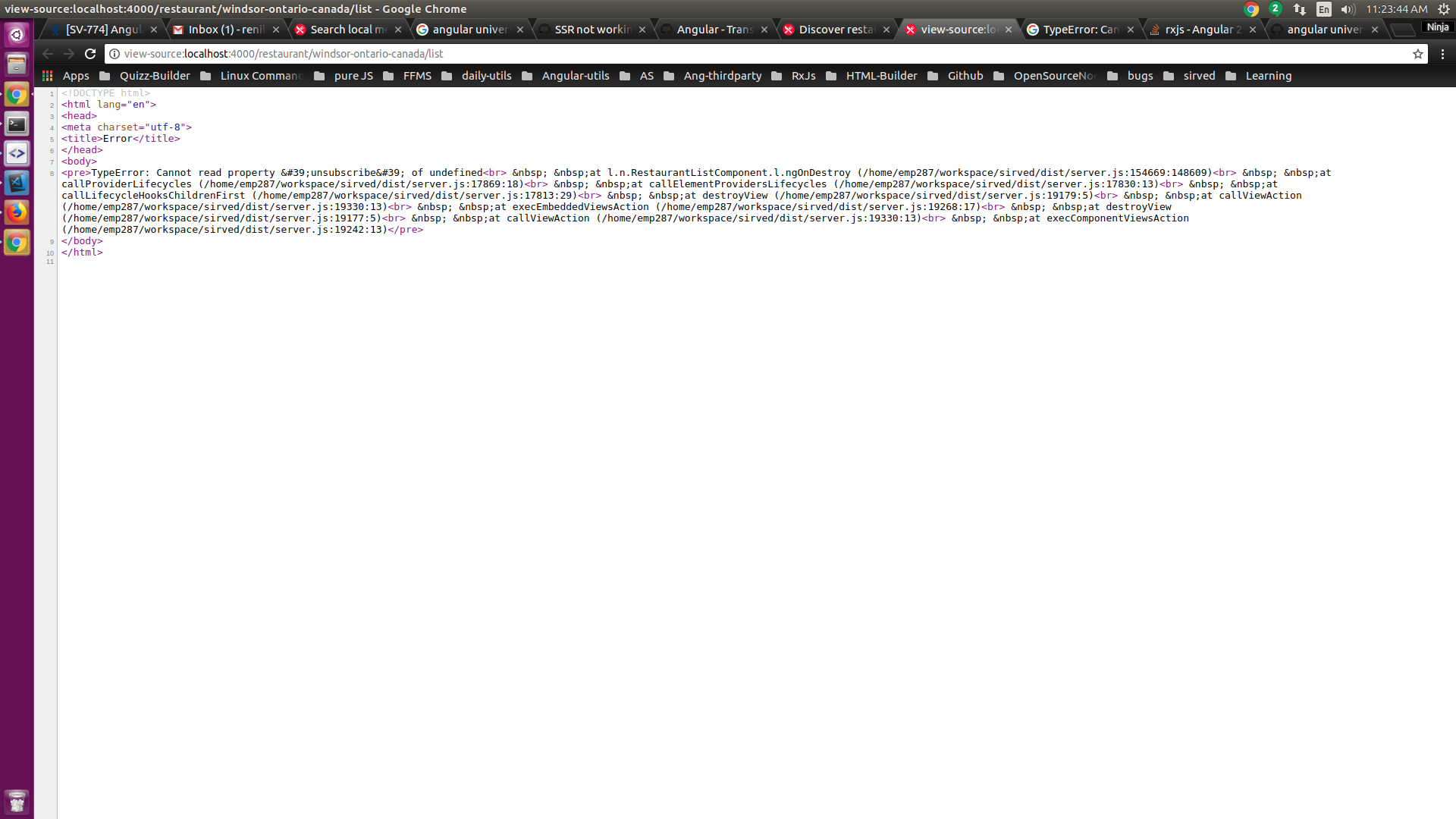
Can anyone look into this issue. Have been stuck in this for past week. No results.
Below are my files .
### 1. angular-cli.json
### 2. server.ts
### 3. prerender.ts
### 4. package.json
I have done exactly same as the angular universal starter project is done but I am not getting the required results. Could anyone please look into this!