Closed SebiK91 closed 3 years ago
Hello @SebiK91,
Thanks for your interest with pyansys
. Would it be possible to upload the *.full file here for me to take a quick look at it? The mass matrix should be available, but I want to double check to see if it's not included in the file.
Hello @akaszynski, here my *.full file, which is from a thermal transient analysis in Ansys:
Thanks for that!
I got some help with this one from @FredAns:
When we perform a thermal transient analysis, the "Mass" matrix is not stored individually in the Full File. For performance concerns, Both K and C matrices are combined into a single "equivalent conductivity matrix".
Here is the description of this combination, and how to extract individual matrices:
Ansys writes the equivalent conductivity matrix to the *.full file for a transient analysis:
[K]
can be retrieved by:
time, 1
and nsbst, 1,1,1
)[C]
can be retrieved by setting k to a very small value for all materials
2 dummy full transient calculations have to be performed. Only *.full file written (wrfull,1)
APDL Math is used to retrieve the matrices [K]
and [C]
: *SMAT
I think if you run the analysis using the above settings and then read in the *.full file using pyansys
, you should be able to get the [K]
and [C]
matrices.
Let me know if you encounter any issues with this.
In a first step I tried your way to retrieve [K] and [C] and I think it works. This is the way I did it:
But I don't understand what I can do with the SMAT function. I think in my two .full files from the two calculations [K] and [C] are separated already.
In the second step reading the *.full files with pyansys works without any trouble. Do you know a way how I can double check if the values are correct? I already tried it with a simple cube but I don't know how to validate the matrices.
Thanks in advance!
The *SMAT
function is used to return the matrices within MAPDL, but it sounds like you'd like to do this outside MAPDL with pyansys
. Totally understandable and pyansys
can support that.
What I'll need is the two *full files to verify that the results are correct. Also, if possible can you upload the entire input file here as well?
Thanks!
I'd like to do it outside MAPDL in Python to do a model order reduction...
Attached I send you the entire input file (K_cross - equivalent conductivity matrix) and my result files for [K] and [C]. Furthermore I send you my cube model.
Thanks!
I've run this using:
import pyansys
c_full = pyansys.read_binary('C.full')
k_full = pyansys.read_binary('K.full')
_, c, _ = c_full.load_km()
_, k, _ = k_full.load_km()
However, I can't verify this as the workbench file isn't loading as it's missing several required files.
If possible, please include the full directory containing the entire workbench directory. Otherwise, you can always create an input file (or use the one workbench generates as a *.dat file) and I can run that instead.
Another way you can verify this is to generate the combined stiffness matrix which is generated by default and then load that as well within pyansys, and then verify the following equation holds true:
import pyansys
c_full = pyansys.read_binary('C.full')
_, c, _ = c_full.load_km()
k_full = pyansys.read_binary('K.full')
_, k, _ = k_full.load_km()
k_bin_eqv = pyansys.read_binary('file.full') # generated by default by MAPDL (equivalent conductivity matrix)
_, k_eqv, _ = k_bin_eqv.load_km()
assert k_eqv == k + c
Aaaah, this works... assert k_eqv == k + c is true :-)
Thank you very much!!!
Do you know if I can apply pyansys also for the input matrix/ load vector and output matrix/ result vector respectively?
Glad that worked out!
I've just added in the ability to access the load vector with:
vec = full_file.load_vector
You'll need pyansys==0.42.1
. As for the result vector, I don't think it's stored in the full file. I'm a bit unfamiliar with transient thermal analyses, but if you're looking for the results from the analysis, you can find them in the file.rst
file and can load that with:
pyansys.read_binary('file.rst')
If this isn't what you're looking for, I can ask internally on Monday. Or, if you could point me to some docs describing what you'd like, I can go searching for it as well.
The load vector command works basically while I get four values at the four nodes of my cube where I applied a temperature (I applied a temperature to one surface of the cube). Unfortunatelly the values I get from the fule_file.load_vector does not correspond to the temperature I am applying. I think the problem is that I have a transient thermal analysis. From this simulation I don't even get a .rst-file but a .rth-file. And I am not sure if 'pyansys' even works for a *.rth-file?
Here you can find a complete simulation result from ansys: cube_res.zip
Correct, the load vector isn't the boundary conditions for the thermal result. You can grab that from the thermal result file.
Actually, pyansys
does support ".rth" result files. They're the same format as a "*.rst" file, but I need to update the docs to reflect that.
You can get the thermal conditions for each result set with:
import pyansys
rth = pyansys.read_binary('file.rth')
temp = rth.nodal_solution(0)
# not supported just yet
# rth.boundary_conditions(0)
I need to add support for boundary conditions, and I think I'll be able to get around to it today (already halfway done).
I really appreciate your help with getting this prototyped, and I'm going to add better documentation to pyansys
to show how to read in thermal result files so that it's clear that pyansys
supports it.
Boundary conditions are now supported as of pyansys==0.42.2
. Should be out on pypi shortly:
>>> import pyansys
>>> rth = pyansys.read_binary('file.rth')
>>> nnum, dof, bc = rth.nodal_boundary_conditions(0)
>>> print(nnum)
>>> print(rth.result_dof(0), bc)
[1 4 3 2]
['TEMP'] [55. 55. 55. 55.]
Oh man - great job. Thank you!
I think I have almost reached my target. In the end I'd like to create the state-space of my Ansys-model in Python - ideally in the form like attached...
I tried different ways according to your descriptions and with approaches according to your method for the other matrices and vectors but it didn't work unfortunatelly. But in my opinion when thermal conductivity and capacity are exportable the it should be possible to export the other matrices as well. Can help me with this?
@SebiK91, sorry that I missed this! Can you show me what you have so far, and I can help you pull out the rest of the data either from MAPDL or from the result file?
No worries, I found a solution with MAPDL to get the matrices. Nevertheless it would be much easier to the matrix export just with Python. Furthermore I realized that I don't have/ need the E-matrix (see the equations above). Is there a way to do export the B- and D-matrix?
When you get a chance, post the code for how you extracted the matrix from MAPDL. We might be able to just transfer that from MAPDL to Python.
As for B, and D, I'm more familiar with the formulation of state space here: https://en.wikipedia.org/wiki/State-space_representation
Either way, it looks like you're looking for the input matrix and output matrix, which I think we figured out we can get from the full file's boundary conditions, right?
Closing soon due to inactivity. @SebiK91, I'll need a little more help with this to make some progress.
Hello everybody,
I am new in ansys and I am using it for my master thesis. For this I am simulating a model in a thermal transient analysis and try to get the mass and stiffness matrix as a dense matrix with the following code:
Unfortunately I just get a stiffness matrix but not mass matrix. Can anybody tell me what I am doing wrong?
Thanks
Markus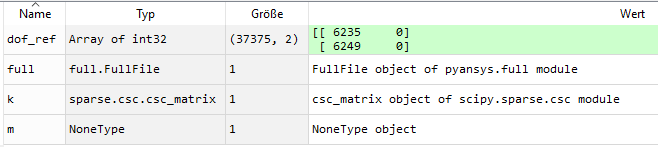