Closed MGlolenstine closed 4 years ago
I'm not sure which menu bar you're talking about, but if you want to hide the header of the tree view, please use this:
tree_view.set_headers_visible(false);
(By the way, please ask gtk-related questions on the gtk+ or gtk-rs related channel, to only keep relm-related issues here.)
Yes, sorry... I thought that it was an empty menu bar, but now I see that it's the header of the tree view... Thanks for the information and in the future I'll make sure to report it on other issue trackers than here, if it's not related to the RELM.
Thanks!
Is there a way to remove the menu bar, which appears after I remove the title bar?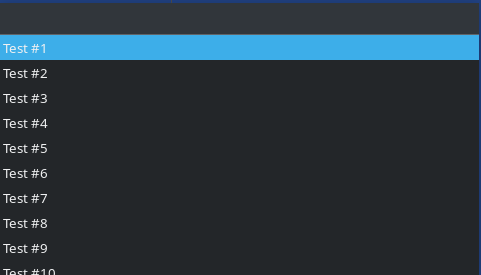
This is my code and I'm not setting any top-padding anywhere.
The code is just slightly modified
treeview.rs
example.