We lack a delay here i think. Because MODEM.waitforesponse won't block the code for 50 ms but just until the modem respond with "OK" (wich just means that the modem stored the data and placed it in a buffer in order to send it) for example, i had a really lenghty JSON that was sended via a http post request and between each write of a byte of data to the MODEM was about 30 ms of "delay", in the end the modem couldn't keep up and responded just with "ERROR". That leads to a state where the modem stops responding. That could be one of the reason why so much people have issues with MKRGSM that stop responding after a random period of time ie: https://github.com/arduino-libraries/MKRGSM/issues/66https://github.com/arduino-libraries/MKRGSM/issues/30.
in the SARA_U201 module page 595 we can read:
After the @ prompt reception, wait for a minimum of 50 ms before sending data.
I think we are in the case of the "Binary section" so we should wait 50ms before sending the data (to be more precise we should send the begining of the command, wait a bit more than 50ms then send the data). So i changed the code a bit:
I was looking at the write function of GSMCLIENT and noticed something :
We lack a delay here i think. Because MODEM.waitforesponse won't block the code for 50 ms but just until the modem respond with "OK" (wich just means that the modem stored the data and placed it in a buffer in order to send it) for example, i had a really lenghty JSON that was sended via a http post request and between each write of a byte of data to the MODEM was about 30 ms of "delay", in the end the modem couldn't keep up and responded just with "ERROR". That leads to a state where the modem stops responding. That could be one of the reason why so much people have issues with MKRGSM that stop responding after a random period of time ie: https://github.com/arduino-libraries/MKRGSM/issues/66 https://github.com/arduino-libraries/MKRGSM/issues/30. in the SARA_U201 module page 595 we can read: After the @ prompt reception, wait for a minimum of 50 ms before sending data.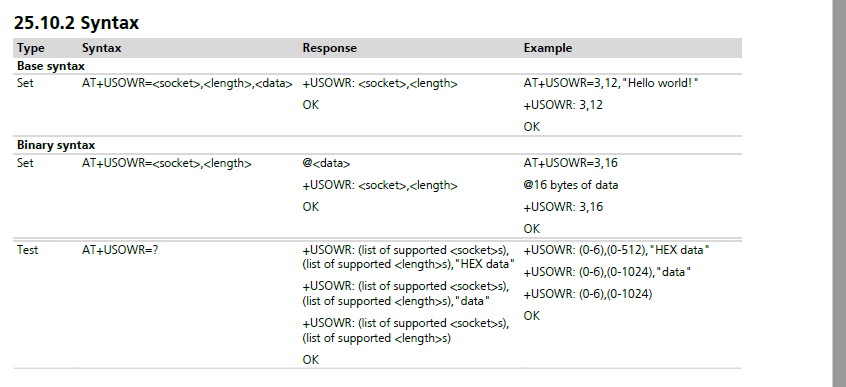
I think we are in the case of the "Binary section" so we should wait 50ms before sending the data (to be more precise we should send the begining of the command, wait a bit more than 50ms then send the data). So i changed the code a bit:
Although i wait after sending the data, it works better for me. What do you guys think?