Open MNS26 opened 3 years ago
I've tested this using a standard Raspberry Pi Pico board and I was able to get the Pico to use a 2nd serial port (as well as still use USB/CDC Virtual Serial), but it requires modifying ArduinoCore-mbed code.
However, I think that this mod requires plenty of testing as on my first few tests it appears unstable. When I reset my board using my own reset button I get an mbed error on the Serial1 port only. But then this error clears on 2nd reset and my test sketch works. The error only happens with a manual reset otherwise my mod appears to work.
++ MbedOS Error Info ++
Error Status: 0x80010133 Code: 307 Module: 1
Error Message: Mutex: 0x2000C274, Not allowed in ISR context
Location: 0x10004007
Error Value: 0x2000C274
Current Thread: rtx_idle Id: 0x2000CE30 Entry: 0x10004011 StackSize: 0x200 StackMem: 0x2000B9B8 SP: 0x2003FD14
For more info, visit: https://mbed.com/s/error?error=0x80010133&osver=61000&core=0x410CC601&comp=2&ver=100300&tgt=RASPBERRY_PI...
-- MbedOS Error Info --
So what did I do... First, you need to open up pin_arduino.h found in the variants/RASPBERRY_PI_PICO folder: https://github.com/arduino/ArduinoCore-mbed/blob/master/variants/RASPBERRY_PI_PICO/pins_arduino.h
Then for my Serial pins I used the following (I believe you are quite limited on choice of pins to prevent clashing with SPI/I2C):
// Serial
#define PIN_SERIAL_TX (0ul)
#define PIN_SERIAL_RX (1ul)
#define PIN_SERIAL_TX2 (8ul)
#define PIN_SERIAL_RX2 (9ul)
Then you add in some new additions. First increase SERIAL_HOWMANY to 2 and then add in SERIAL2 TX/RX
#define SERIAL_HOWMANY 2
#define SERIAL1_TX (digitalPinToPinName(PIN_SERIAL_TX))
#define SERIAL1_RX (digitalPinToPinName(PIN_SERIAL_RX))
#define SERIAL2_TX (digitalPinToPinName(PIN_SERIAL_TX2))
#define SERIAL2_RX (digitalPinToPinName(PIN_SERIAL_RX2))
And that is all I did.
I was not sure about this code though and what it was doing internally:
#define SERIAL_PORT_HARDWARE Serial1
#define SERIAL_PORT_HARDWARE_OPEN Serial1
Then for my Arduino sketch, I simply modified the existing SerialPassthrough sketch as follows:
void setup() {
Serial.begin(115200);
Serial1.begin(115200);
Serial2.begin(115200);
delay(100);
Serial.println("Lets start");
// Just a quick initial test to make sure I am seeing text in both my serial terminals
for (auto i = 0; i < 6; i++) {
delay(3000);
Serial1.println("Hello world 1");
Serial2.println("Hello world 2");
}
Serial.println("We are finished first test");
}
void loop() {
if (Serial2.available()) { // If anything comes in Serial (USB),
Serial1.write(Serial2.read()); // read it and send it out Serial1 (pins 0 & 1)
Serial.print("."); // output via usb just in case we don't get anything
}
if (Serial1.available()) { // If anything comes in Serial1 (pins 0 & 1)
Serial2.write(Serial1.read()); // read it and send it out Serial (USB)
Serial.print("."); // output via usb just in case we don't get anything
}
}
Hi @MNS26 and @Gerriko , I just posted a PR https://github.com/arduino/ArduinoCore-mbed/pull/240 to ease the creation of additional serial objects without touching the core. By applying it the resulting sketch will look like
UART Serial2(8,9);
void setup() {
Serial.begin(115200);
Serial1.begin(115200);
Serial2.begin(115200);
delay(100);
Serial.println("Lets start");
// Just a quick initial test to make sure I am seeing text in both my serial terminals
for (auto i = 0; i < 6; i++) {
delay(3000);
Serial1.println("Hello world 1");
Serial2.println("Hello world 2");
}
Serial.println("We are finished first test");
}
void loop() {
if (Serial2.available()) { // If anything comes in Serial (USB),
Serial1.write(Serial2.read()); // read it and send it out Serial1 (pins 0 & 1)
Serial.print("."); // output via usb just in case we don't get anything
}
if (Serial1.available()) { // If anything comes in Serial1 (pins 0 & 1)
Serial2.write(Serial1.read()); // read it and send it out Serial (USB)
Serial.print("."); // output via usb just in case we don't get anything
}
}
I tested it on a Pico and couldn't reproduce the crash that @Gerriko is reporting. Could you test if the issue is reproducible after the PR is applied? Thx
Hi @MNS26 and @Gerriko , I just posted a PR #240 to ease the creation of additiona serial objects without touching the core. By apply it the resulting sketch will look like
UART Serial2(8,9); void setup() { Serial.begin(115200); Serial1.begin(115200); Serial2.begin(115200); delay(100); Serial.println("Lets start"); // Just a quick initial test to make sure I am seeing text in both my serial terminals for (auto i = 0; i < 6; i++) { delay(3000); Serial1.println("Hello world 1"); Serial2.println("Hello world 2"); } Serial.println("We are finished first test"); } void loop() { if (Serial2.available()) { // If anything comes in Serial (USB), Serial1.write(Serial2.read()); // read it and send it out Serial1 (pins 0 & 1) Serial.print("."); // output via usb just in case we don't get anything } if (Serial1.available()) { // If anything comes in Serial1 (pins 0 & 1) Serial2.write(Serial1.read()); // read it and send it out Serial (USB) Serial.print("."); // output via usb just in case we don't get anything } }
I tested it on a Pico and couldn't reproduce the crash that @Gerriko is reporting. Could you test if the issue is reproducible after the PR is applied? Thx
@facchinm after i edited the pins_arduino.h file and tried to use your example i cant get it to compile, everytime i try to do it i get this
> Executing task: C:\Users\MS26\.platformio\penv\Scripts\platformio.exe run --environment pico helicopter <
Processing pico helicopter (platform: https://github.com/platformio/platform-raspberrypi.git; framework: arduino; board: pico)
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------Verbose mode can be enabled via `-v, --verbose` option
CONFIGURATION: https://docs.platformio.org/page/boards/raspberrypi/pico.html
PLATFORM: Raspberry Pi RP2040 (1.0.0+sha.095da74) > Raspberry Pi Pico
HARDWARE: RP2040 133MHz, 264KB RAM, 2MB Flash
DEBUG: Current (cmsis-dap) External (cmsis-dap, jlink, raspberrypi-swd)
PACKAGES:
- framework-arduino-mbed 2.1.0
- tool-rp2040tools 1.0.2
- toolchain-gccarmnoneeabi 1.90201.191206 (9.2.1)
LDF: Library Dependency Finder -> http://bit.ly/configure-pio-ldf
LDF Modes: Finder ~ chain, Compatibility ~ soft
Found 31 compatible libraries
Scanning dependencies...
Dependency Graph
|-- <Wire>
|-- <TinyGPSPlus> 1.0.2
Building in release mode
Compiling .pio\build\pico helicopter\src\main.cpp.o
Compiling .pio\build\pico helicopter\src\main1.cpp.o
Compiling .pio\build\pico helicopter\lib1b0\Wire\Wire.cpp.o
Compiling .pio\build\pico helicopter\libf0e\TinyGPSPlus\TinyGPS++.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduinoVariant\double_tap_usb_boot.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduinoVariant\variant.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\Interrupts.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\Serial.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\Tone.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\USB\PluggableUSBDevice.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\USB\USBCDC.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\USB\USBSerial.cpp.o
In file included from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/platform_defs.h:12,
from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/pico_platform/include/pico/platform.h:12,
from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/common/pico_base/include/pico.h:19,
from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\variants\RASPBERRY_PI_PICO\double_tap_usb_boot.cpp:2:
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h: In function 'uint32_t time_us_32()':
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/regs/addressmap.h:56:20: warning: type qualifiers ignored on cast result type [-Wignored-qualifiers]
56 | #define TIMER_BASE 0x40054000
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_structs/include/hardware/structs/timer.h:33:38: note: in expansion of macro 'TIMER_BASE'
33 | #define timer_hw ((timer_hw_t *const)TIMER_BASE)
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h:66:12: note: in expansion of macro 'timer_hw'
66 | return timer_hw->timerawl;
| ^~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h: In function 'bool time_reached(absolute_time_t)':
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/regs/addressmap.h:56:20: warning: type qualifiers ignored on cast result type [-Wignored-qualifiers]
56 | #define TIMER_BASE 0x40054000
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_structs/include/hardware/structs/timer.h:33:38: note: in expansion of macro 'TIMER_BASE'
33 | #define timer_hw ((timer_hw_t *const)TIMER_BASE)
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h:110:19: note: in expansion of macro 'timer_hw'
110 | uint32_t hi = timer_hw->timerawh;
| ^~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/regs/addressmap.h:56:20: warning: type qualifiers ignored on cast result type [-Wignored-qualifiers]
56 | #define TIMER_BASE 0x40054000
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_structs/include/hardware/structs/timer.h:33:38: note: in expansion of macro 'TIMER_BASE'
33 | #define timer_hw ((timer_hw_t *const)TIMER_BASE)
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h:111:33: note: in expansion of macro 'timer_hw'
111 | return (hi >= hi_target && (timer_hw->timerawl >= (uint32_t) target || hi != hi_target));
| ^~~~~~~~
src\main1.cpp:16: warning: "PIN_SERIAL_TX" redefined
16 | #define PIN_SERIAL_TX (4)
|
In file included from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Arduino.h:79,
from src\main1.cpp:1:
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\variants\RASPBERRY_PI_PICO/pins_arduino.h:41: note: this is the location of the previous definition
41 | #define PIN_SERIAL_TX (0ul)
|
src\main1.cpp:17: warning: "PIN_SERIAL_RX" redefined
17 | #define PIN_SERIAL_RX (5)
|
In file included from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Arduino.h:79,
from src\main1.cpp:1:
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\variants\RASPBERRY_PI_PICO/pins_arduino.h:42: note: this is the location of the previous definition
42 | #define PIN_SERIAL_RX (1ul)
|
src\main.cpp:3:17: error: no matching function for call to 'arduino::UART::UART(int, int)'
3 | UART Serial2(4,5);
| ^
In file included from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Arduino.h:105,
from src\main.cpp:1:
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:42:3: note: candidate: 'arduino::UART::UART()'
42 | UART() {
| ^~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:42:3: note: candidate expects 0 arguments, 2 provided
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:41:3: note: candidate: 'arduino::UART::UART(int, int, int, int)'
41 | UART(int tx, int rx, int rts, int cts) : _tx((PinName)tx), _rx((PinName)rx), _rts((PinName)rts), _cts((PinName)cts) {}
| ^~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:41:3: note: candidate expects 4 arguments, 2 provided
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate: 'constexpr arduino::UART::UART(const arduino::UART&)'
39 | class UART : public HardwareSerial {
| ^~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate expects 1 argument, 2 provided
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate: 'constexpr arduino::UART::UART(arduino::UART&&)'
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate expects 1 argument, 2 provided
.pio\libdeps\pico helicopter\TinyGPSPlus\src\TinyGPS++.cpp: In member function 'bool TinyGPSPlus::encode(char)':
.pio\libdeps\pico helicopter\TinyGPSPlus\src\TinyGPS++.cpp:63:12: warning: this statement may fall through [-Wimplicit-fallthrough=]
63 | parity ^= (uint8_t)c;
| ~~~~~~~^~~~~~~~~~~~~
.pio\libdeps\pico helicopter\TinyGPSPlus\src\TinyGPS++.cpp:64:3: note: here
64 | case '\r':
| ^~~~
*** [.pio\build\pico helicopter\src\main.cpp.o] Error 1
================================================================================================ [FAILED] Took 16.64 seconds ================================================================================================
The terminal process "C:\Users\MS26\.platformio\penv\Scripts\platformio.exe 'run', '--environment', 'pico helicopter'" terminated with exit code: 1.
Terminal will be reused by tasks, press any key to close it.
and the code i used
#include <Arduino.h>
UART Serial2(4,5);
void setup() {
Serial.begin(115200);
Serial1.begin(115200);
Serial2.begin(115200);
delay(100);
Serial.println("Lets start");
// Just a quick initial test to make sure I am seeing text in both my serial terminals
for (auto i = 0; i < 6; i++) {
delay(3000);
Serial1.println("Hello world 1");
Serial2.println("Hello world 2");
}
Serial.println("We are finished first test");
}
void loop() {
if (Serial2.available()) { // If anything comes in Serial (USB),
Serial.write(Serial2.read()); // read it and send it out Serial1 (pins 0 & 1)
Serial.println(); // output via usb just in case we don't get anything
}
/*
if (Serial1.available()) { // If anything comes in Serial1 (pins 0 & 1)
Serial2.write(Serial1.read()); // read it and send it out Serial (USB)
Serial.print("."); // output via usb just in case we don't get anything
}*/
}
@MNS26 you say " i edited the pins_arduino.h file "
When looking through the error log it tells you "_warning: "PIN_SERIALTX" redefined" and "_warning: "PIN_SERIALRX" redefined".
That suggests to me that Serial2 and Serial1 and trying to use the same PIN_SERIAL_TX and PIN_SERIAL_RX.
Maybe check your pins_arduino.h file again.
@Gerriko I have pin GP4 and pin GP5 for serial 1 (GPS) and plan on using GP12 and GP13 for serial 0 (RC receiver). In the pins_arduino.h file pin definition
// Serial
#define PIN_SERIAL_TX (0ul)
#define PIN_SERIAL_RX (1ul)
#define PIN_SERIAL_TX2 (8ul)
#define PIN_SERIAL_RX2 (9ul)
serialX definition
//SERIAL Setup
#define SERIAL_HOWMANY 2
#define SERIAL1_TX (digitalPinToPinName(PIN_SERIAL_TX))
#define SERIAL1_RX (digitalPinToPinName(PIN_SERIAL_RX))
#define SERIAL2_TX (digitalPinToPinName(PIN_SERIAL_TX2))
#define SERIAL2_RX (digitalPinToPinName(PIN_SERIAL_RX2))
i used GP0 and GP1 for a I2C sensor (mpu6050 with auxiliary I2C enabled for compass).
EDIT: Found the redefine issue (a file i forgot to remove in the project folder). but now the output changed to
> Executing task: C:\Users\MS26\.platformio\penv\Scripts\platformio.exe run --environment pico helicopter <
Processing pico helicopter (platform: https://github.com/platformio/platform-raspberrypi.git; framework: arduino; board: pico)
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------Verbose mode can be enabled via `-v, --verbose` option
CONFIGURATION: https://docs.platformio.org/page/boards/raspberrypi/pico.html
PLATFORM: Raspberry Pi RP2040 (1.0.0+sha.095da74) > Raspberry Pi Pico
HARDWARE: RP2040 133MHz, 264KB RAM, 2MB Flash
DEBUG: Current (cmsis-dap) External (cmsis-dap, jlink, raspberrypi-swd)
PACKAGES:
- framework-arduino-mbed 2.1.0
- tool-rp2040tools 1.0.2
- toolchain-gccarmnoneeabi 1.90201.191206 (9.2.1)
LDF: Library Dependency Finder -> http://bit.ly/configure-pio-ldf
LDF Modes: Finder ~ chain, Compatibility ~ soft
Found 31 compatible libraries
Scanning dependencies...
No dependencies
Building in release mode
Compiling .pio\build\pico helicopter\src\main.cpp.o
Archiving .pio\build\pico helicopter\libFrameworkArduinoVariant.a
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_analog.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_digital.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_pulse.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_shift.cpp.o
src\main.cpp:3:17: error: no matching function for call to 'arduino::UART::UART(int, int)'
3 | UART Serial2(4,5);
| ^
In file included from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Arduino.h:105,
from src\main.cpp:1:
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:42:3: note: candidate: 'arduino::UART::UART()'
42 | UART() {
| ^~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:42:3: note: candidate expects 0 arguments, 2 provided
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:41:3: note: candidate: 'arduino::UART::UART(int, int, int, int)'
41 | UART(int tx, int rx, int rts, int cts) : _tx((PinName)tx), _rx((PinName)rx), _rts((PinName)rts), _cts((PinName)cts) {}
| ^~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:41:3: note: candidate expects 4 arguments, 2 provided
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate: 'constexpr arduino::UART::UART(const arduino::UART&)'
39 | class UART : public HardwareSerial {
| ^~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate expects 1 argument, 2 provided
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate: 'constexpr arduino::UART::UART(arduino::UART&&)'
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/Serial.h:39:7: note: candidate expects 1 argument, 2 provided
*** [.pio\build\pico helicopter\src\main.cpp.o] Error 1
==================================================================================================== [FAILED] Took 5.55 seconds ====================================================================================================The terminal process "C:\Users\MS26\.platformio\penv\Scripts\platformio.exe 'run', '--environment', 'pico helicopter'" terminated with exit code: 1.
Terminal will be reused by tasks, press any key to close it.
But when i remove the line
UART Serial2(4,5);
will compile without any issues at all.
Is there anything else i can try to get it to work?
@facchinm do you have any idea why it does compile (but not work) when removing the UART Serial2(4,5);
line?
Ive been trying things out in my free time here and there but i either fail to compile it (same thing as previous message) or the serial port is not made.
Should i see this project as a lost cause and "drop it"? or is there still a light somewhere at the end of the tunnel.
The proposed code did not compile as it was shown there.
UART Serial2(8, 9); // did not compile
I got the following error:
no matching function for call to 'arduino::UART::UART(int, int)'
...
note: candidate: arduino::UART::UART(int, int, int, int)
What worked for me was:
UART Serial2(8, 9, 0, 0);
Full code:
#include <Arduino.h>
UART Serial2(8, 9, 0, 0);
void setup() {
Serial2.begin(9600);
// ...
Serial2.write("hello world");
}
void loop {}
@resolvethis Did you also edit the pins_arduino.h file? When i try and use your code (and adding the () to loop) i still get a error.
Processing pico helicopter (platform: https://github.com/platformio/platform-raspberrypi.git; framework: arduino; board: pico)
----------------------------------------------------------------------------------------------------------------------------------------------------------------------Verbose mode can be enabled via `-v, --verbose` option
CONFIGURATION: https://docs.platformio.org/page/boards/raspberrypi/pico.html
PLATFORM: Raspberry Pi RP2040 (1.1.0+sha.3c6cc84) > Raspberry Pi Pico
HARDWARE: RP2040 133MHz, 264KB RAM, 2MB Flash
DEBUG: Current (cmsis-dap) External (cmsis-dap, jlink, raspberrypi-swd)
PACKAGES:
- framework-arduino-mbed 2.1.0
- tool-rp2040tools 1.0.2
- toolchain-gccarmnoneeabi 1.90201.191206 (9.2.1)
LDF: Library Dependency Finder -> http://bit.ly/configure-pio-ldf
LDF Modes: Finder ~ chain, Compatibility ~ soft
Found 31 compatible libraries
Scanning dependencies...
Dependency Graph
|-- <TinyGPSPlus> 1.0.2
Building in release mode
Compiling .pio\build\pico helicopter\src\main.cpp.o
Compiling .pio\build\pico helicopter\libf0e\TinyGPSPlus\TinyGPS++.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduinoVariant\double_tap_usb_boot.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduinoVariant\variant.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\Interrupts.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\Serial.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\Tone.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\USB\PluggableUSBDevice.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\USB\USBCDC.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\USB\USBSerial.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\main.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\pinToIndex.cpp.o
In file included from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/platform_defs.h:12,
from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/pico_platform/include/pico/platform.h:12,
from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/common/pico_base/include/pico.h:19,
from C:\Users\MS26\.platformio\packages\framework-arduino-mbed\variants\RASPBERRY_PI_PICO\double_tap_usb_boot.cpp:2:
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h: In function 'uint32_t time_us_32()':
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/regs/addressmap.h:56:20: warning: type qualifiers ignored on cast result type [-Wignored-qualifiers]
56 | #define TIMER_BASE 0x40054000
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_structs/include/hardware/structs/timer.h:33:38: note: in expansion of macro 'TIMER_BASE'
33 | #define timer_hw ((timer_hw_t *const)TIMER_BASE)
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h:66:12: note: in expansion of macro 'timer_hw'
66 | return timer_hw->timerawl;
| ^~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h: In function 'bool time_reached(absolute_time_t)':
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/regs/addressmap.h:56:20: warning: type qualifiers ignored on cast result type [-Wignored-qualifiers]
56 | #define TIMER_BASE 0x40054000
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_structs/include/hardware/structs/timer.h:33:38: note: in expansion of macro 'TIMER_BASE'
33 | #define timer_hw ((timer_hw_t *const)TIMER_BASE)
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h:110:19: note: in expansion of macro 'timer_hw'
110 | uint32_t hi = timer_hw->timerawh;
| ^~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_regs/include/hardware/regs/addressmap.h:56:20: warning: type qualifiers ignored on cast result type [-Wignored-qualifiers]
56 | #define TIMER_BASE 0x40054000
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2040/hardware_structs/include/hardware/structs/timer.h:33:38: note: in expansion of macro 'TIMER_BASE'
33 | #define timer_hw ((timer_hw_t *const)TIMER_BASE)
| ^~~~~~~~~~
C:\Users\MS26\.platformio\packages\framework-arduino-mbed\cores\arduino/mbed/targets/TARGET_RASPBERRYPI/TARGET_RP2040/pico-sdk/rp2_common/hardware_timer/include/hardware/timer.h:111:33: note: in expansion of macro 'timer_hw'
111 | return (hi >= hi_target && (timer_hw->timerawl >= (uint32_t) target || hi != hi_target));
| ^~~~~~~~
.pio\libdeps\pico helicopter\TinyGPSPlus\src\TinyGPS++.cpp: In member function 'bool TinyGPSPlus::encode(char)':
.pio\libdeps\pico helicopter\TinyGPSPlus\src\TinyGPS++.cpp:63:12: warning: this statement may fall through [-Wimplicit-fallthrough=]
63 | parity ^= (uint8_t)c;
| ~~~~~~~^~~~~~~~~~~~~
.pio\libdeps\pico helicopter\TinyGPSPlus\src\TinyGPS++.cpp:64:3: note: here
64 | case '\r':
| ^~~~
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_analog.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_digital.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_pulse.cpp.o
Compiling .pio\build\pico helicopter\FrameworkArduino\wiring_shift.cpp.o
Archiving .pio\build\pico helicopter\libFrameworkArduino.a
Linking .pio\build\pico helicopter\firmware.elf
c:/users/ms26/.platformio/packages/toolchain-gccarmnoneeabi/bin/../lib/gcc/arm-none-eabi/9.2.1/../../../../arm-none-eabi/bin/ld.exe: .pio\build\pico helicopter\libFrameworkArduino.a(Serial.cpp.o):(.bss._UART2_+0x0): multiple definition of `_UART2_'; .pio\build\pico helicopter\src\main.cpp.o:(.bss._UART2_+0x0): first defined here
collect2.exe: error: ld returned 1 exit status
*** [.pio\build\pico helicopter\firmware.elf] Error 1
==================================================================== [FAILED] Took 13.01 seconds ====================================================================
The terminal process "C:\Users\MS26\.platformio\penv\Scripts\platformio.exe 'run', '--environment', 'pico helicopter'" terminated with exit code: 1.
Terminal will be reused by tasks, press any key to close it.
but when i set #define SERIAL_HOWMANY
to 1 it compiles without a problem.
@facchinm has there been any news on this issue? I have yet to find a way to use multiple serial ports at once.
Many thanks to those above, especially facchinm: Below is what I used for my NEO GPS connected to the Pico. I had trouble with Serial1, every time I used Serial1 along with Serial it would lock up the PC comport. I'm guessing that serial1 is the same as serial? I don't know, but the following code worked for me:
TinyGPSPlus gps;
// The serial connection to the GPS device UART Serial2(8,9); //THE GPS TX goes to GP9 to work, 9 on the PICO to the recieve pin //THE GPS TX goes to GP9 to work, 9 on the PICO to the recieve pin
void setup() { Serial.begin(9600); Serial2.begin(9600); //You might be 4800 depending on your GPS? }
void loop() {
delay(500); // This sketch displays information every time a new sentence is correctly encoded. while (Serial2.available() > 0) { if (gps.encode(Serial2.read())) displayInfo(); }
if (millis() > 5000 && gps.charsProcessed() < 10) { Serial.println(F("No GPS detected: check wiring.")); //while(true); delay(7000); Serial.println("Waited 7 sec for you to wire"); } }
void displayInfo() { Serial.print(F("Location: ")); if (gps.location.isValid()) { Serial.print(gps.location.lat(), 6); Serial.print(F(",")); Serial.print(gps.location.lng(), 6); } else { Serial.print(F("INVALID")); }
Serial.print(F(" Date/Time: ")); if (gps.date.isValid()) { Serial.print(gps.date.month()); Serial.print(F("/")); Serial.print(gps.date.day()); Serial.print(F("/")); Serial.print(gps.date.year()); } else { Serial.print(F("INVALID")); }
Serial.print(F(" ")); if (gps.time.isValid()) { if (gps.time.hour() < 10) Serial.print(F("0")); Serial.print(gps.time.hour()); Serial.print(F(":")); if (gps.time.minute() < 10) Serial.print(F("0")); Serial.print(gps.time.minute()); Serial.print(F(":")); if (gps.time.second() < 10) Serial.print(F("0")); Serial.print(gps.time.second()); Serial.print(F(".")); if (gps.time.centisecond() < 10) Serial.print(F("0")); Serial.print(gps.time.centisecond()); } else { Serial.print(F("INVALID")); }
Serial.println(); }
I was redirected here form platfromIO to open an issue about it. ( https://github.com/platformio/platform-raspberrypi/issues/3 )
I've been trying it read my gps module for a project and decided to use the pi pico for it, however even though it "sees" the connection (no check wire connection message) it receives no data.
i used the default "FullExampel" file from the TinyGPS library i removed SoftwareSerial because there is no interrup.h file and replaced it with Serial1 (RP pi pico should be able to have 2 hardware serial connections)
GPS lock is established (red led)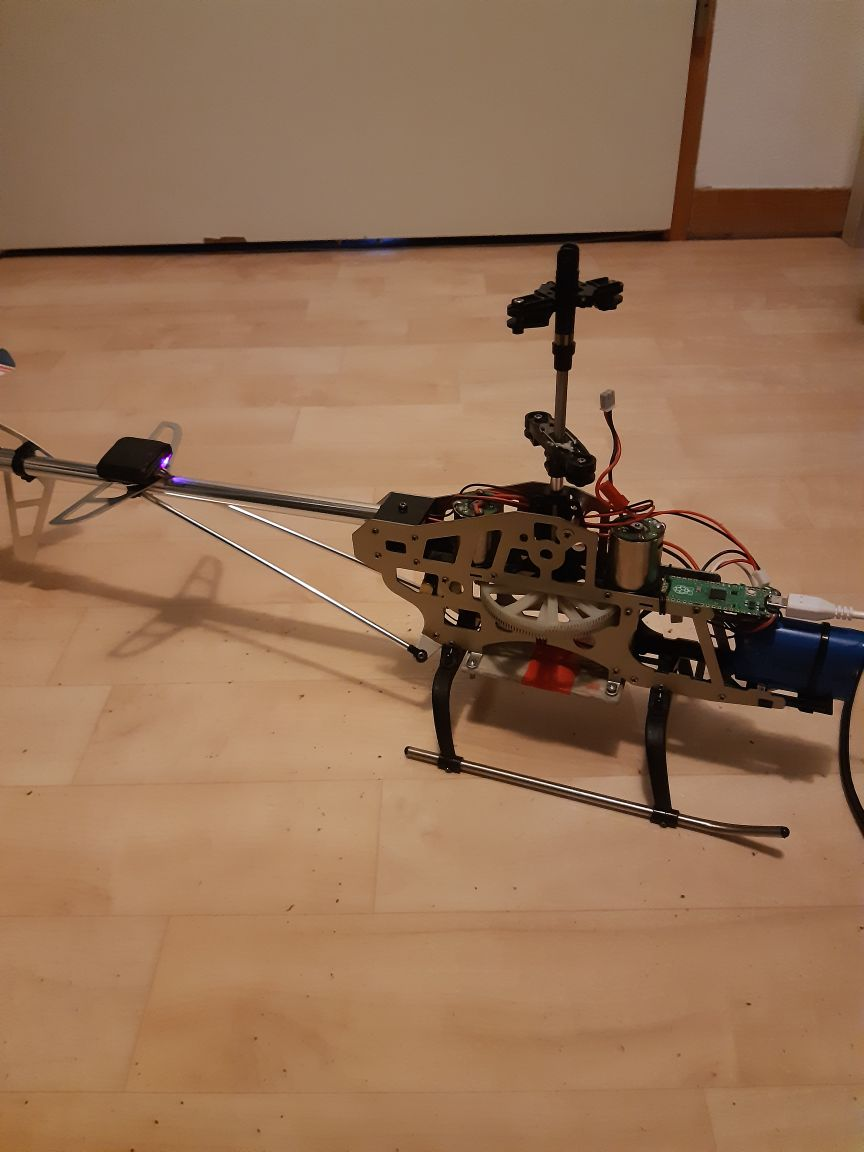
data from serial console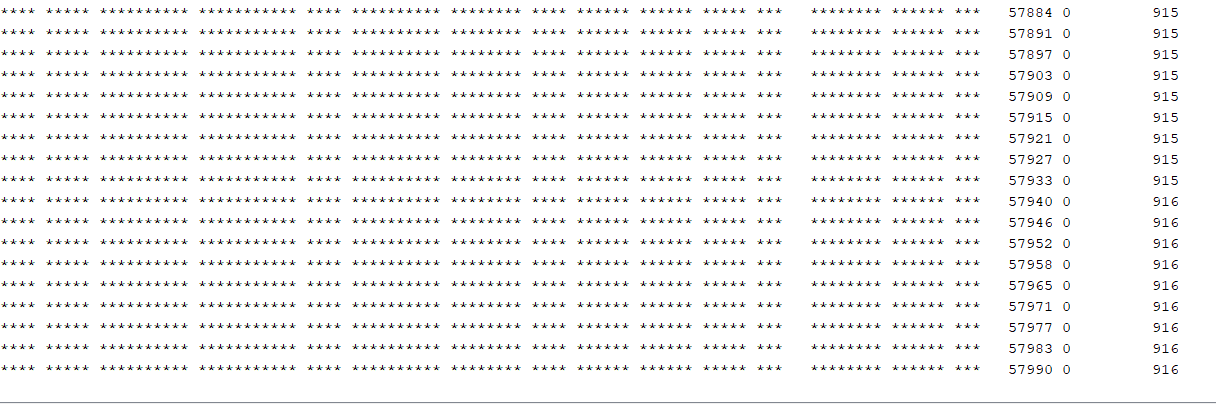