Closed sunnyHelen closed 2 years ago
Hi @sunnyHelen, thanks for your interest in our work. Please take a look at the Stereo Tutorial notebook.
If you are working with the Argoverse Stereo v1.1 Dataset (rectified data preferred for the Stereo Benchmark, see https://www.argoverse.org/data.html#download-link), you would use:
from argoverse.data_loading.stereo_dataloader import ArgoverseStereoDataLoader
loader = ArgoverseStereoDataLoader(data_dir=DATA_ROOT, split_name="train") # or name of your desired split
ordered_img_paths = get_ordered_log_stereo_image_fpaths(log_id=LOG_ID, camera_name="stereo_front_left_rect")
i = 10
print(ordered_img_paths[i])
print(ordered_img_paths[i+1])
vs. Argoverse 3D Tracking v1.1, you would use a different dataloader:
from argoverse.data_loading.simple_track_dataloader import SimpleArgoverseTrackingDataLoader
class SimpleArgoverseTrackingDataLoader(data_dir=DATA_ROOT, labels_dir=DATA_ROOT)
ordered_img_fpaths = get_ordered_log_cam_fpaths(log_id=LOG_ID, camera_name="stereo_front_left")
Please take a look at the tracking tutorial here.
Thanks for your reply. I mean when I have the filename of the current frame, for example, "argoverse-tracking/train1/1d676737-4110-3f7e-bec0-0c90f74c248f/stereo_front_left/stereo_front_left_315984808066087728.jpg“, how can I get the filename of its next frame quickly?
I would recommend doing:
from pathlib import Path
... (code above) ...
ordered_img_fnames = [Path(fpath) for fpath in ordered_img_fpaths]
i = ordered_img_fnames.index(query_fname)
next_fname = ordered_img_fnames[i+1)
Or, you could create a lookup table:
next_fname_lookup = {ordered_img_fnames[i]:ordered_img_fnames[i+1] for i in range(len(ordered_img_fnames)-1) }
Ok, thanks a lot for your reply.
Due to the complicated naming rules, I cannot get the filename of the next image.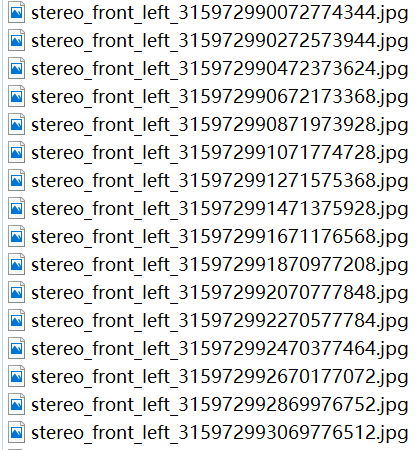
Like in KITTI, the next frame can be obtained by simply adding 1 to the current frame index. I guess there is a way to get the filename of the next image is that by getting the image_list of a certain log_id, getting the index of the current image, and using the new index to get the filename of the next image. However, this way is very time-consuming. I want to know how to quickly get the filename of the next image.