Closed Liqiankun closed 2 years ago
I solved it. Then key is video path.
const finalVideo = `${RNFS.CachesDirectoryPath}/${Date.now()}.mp4`;
const str_cmd = `-i ${rVideoUrl} -c:v mpeg4 ${finalVideo}`; // `y -i ${rVideoUrl} -c:v libx264 -crf 28 -preset ultrafast ${finalVideo}`;
FFmpegKit.executeAsync(
str_cmd,
session => {
const returnCode = session.getReturnCode();
if (ReturnCode.isSuccess(returnCode)) {
callback({
videoPath: 'file://' + finalVideo
});
} else if (ReturnCode.isCancel(returnCode)) {
setLoading(false);
Alert.alert('提示', '压缩取消');
} else {
setLoading(false);
Alert.alert('提示', '压缩失败');
}
},
log => {
Alert.alert('提示', log.getMessage());
},
statistics => {
setProgress(Math.ceil((statistics.getTime() / 1000 / time) * 100));
}
);
Description release mode not working on android, debug node works fine
Expected behavior works on both modes
Current behavior nothing happened
Screenshots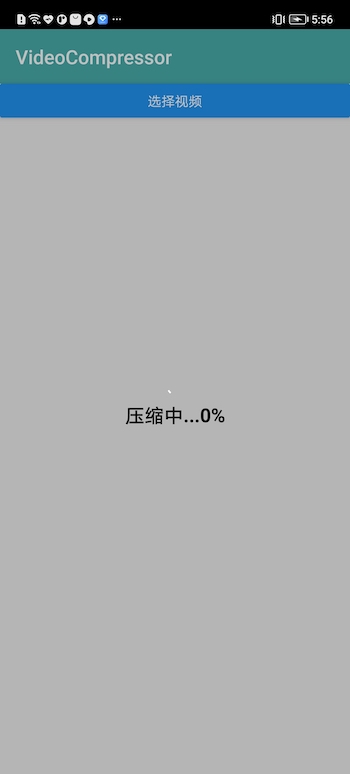
Logs No logs
Environment
Android``ReactNative
arm64-v8a
^4.5.2
main
,4.0
24
System: OS: macOS 10.15.7 CPU: (12) x64 Intel(R) Core(TM) i5-10600 CPU @ 3.30GHz Memory: 4.10 GB / 16.00 GB Shell: 5.8 - /usr/local/bin/zsh Binaries: Node: 12.18.3 - /usr/local/bin/node Yarn: 1.21.1 - /usr/local/bin/yarn npm: 6.14.6 - /usr/local/bin/npm Watchman: 4.9.0 - /usr/local/bin/watchman Managers: CocoaPods: 1.10.1 - /usr/local/bin/pod SDKs: iOS SDK: Platforms: iOS 14.4, DriverKit 20.2, macOS 11.1, tvOS 14.3, watchOS 7.2 Android SDK: API Levels: 23, 27, 28, 29, 30 Build Tools: 28.0.3, 29.0.2, 29.0.3 System Images: android-19 | Intel x86 Atom, android-24 | Google APIs Intel x86 Atom_64, android-28 | Intel x86 Atom_64 Android NDK: Not Found IDEs: Android Studio: 4.0 AI-193.6911.18.40.6514223 Xcode: 12.4/12D4e - /usr/bin/xcodebuild Languages: Java: 1.8.0_251 - /usr/bin/javac Python: 2.7.16 - /usr/bin/python npmPackages: @react-native-community/cli: Not Found react: Not Found react-native: Not Found npmGlobalPackages: *react-native*: Not Found
Code