Closed Abrynos closed 5 years ago
That mapping is configured here: https://github.com/AzureAD/azure-activedirectory-identitymodel-extensions-for-dotnet/blob/d2361e5dcd1abbf6d0ea441cdb2e7404166b122c/src/System.IdentityModel.Tokens.Jwt/ClaimTypeMapping.cs#L61
You can call JwtSecurityTokenHandler.DefaultInboundClaimTypeMap.Clear() if you want to remove those.
Thanks for pointing it out, although it doesn't make sense having to do that in the first place IMHO
Currently i'm writing a project for university using JWT-Authentication. When creating JWTs in my TokenManager i add a claim with the type
JwtRegisteredClaimNames.UniqueName
which is just"unique_name"
. In my Controllers now i try to get the unique name of the user sending the JWT withHttpContext?.User?.Claims?.FirstOrDefault(c => c.Type == JwtRegisteredClaimNames.UniqueName)?.Value
which always returns null. So i made a foreach loop to just write all Claims to the console. Thing is: according to jwt.io the claim is saved (as intended) tounique_name
The JWT decoder puts that claim in a Claim-object, which' Type-property has the value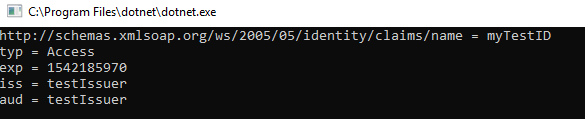
http://schemas.xmlsoap.org/ws/2005/05/identity/claims/name
(an equivalent to System.Security.Claims.ClaimTypes.Name) as shown in the console outputIf this is intended behaviour i'd appreciate you explaining why or pointing to where i can find out :)
Here's the code of a test-project i checked with:
Program.cs
TestKestrel.cs
Startup.cs
TestController.cs
TokenManager.cs