Closed ignitum-ryan closed 2 years ago
@ignitum-ryan - Thanks for posting this issue. I believe it is a duplicate of this issue. Please check there for updates.
Contributor
Thanks for the reply @haverchuck . The issue does seem similar. The only piece that I'm not sure about is that the issue you linked has no mention of the varying 'ID!' fields being dropped from the ModelMutations.create() function. This could be semantics, I just want to make sure that the other ticket notes that it's impossible to create records that contain the multiple 'belongsTo' annotated fields.
Thanks again.
thanks you @ignitum-ryan
Description
When attempting to create new models that contain multiple belongsTo annotations, it is returning the error 'Variable 'input' has coerced Null value for NonNull type 'ID!'
Here are the model schemas being used:
The code used to create the models and attempt to save in the database:
The saving of the Friendship is successful, however, for each of the saving of the friends, the response returns a GraphQL error stating: 'Variable 'input' has coerced Null value for NonNull type 'ID!'
The reason I believe it has to do with the multiple belongsTo annotations is due to the ordering of the Friend model. The model coming into the saveNewFriend function appears correctly (Notated above as 'LINE A'), meaning it has all of the fields populated and the correct relationship. (See screenshots below). However, once the request object is returned from the ModelMutations.create() function, it DROPS the friendshipID (also in screenshots below). If I go to the schema.graphql file and SWAP the positions of the user/userID field with the friendship/friendshipID field, then the request object returned will DROP the userID resulting in the same error.
According to the documentation found here, it specifies that multiple belongsTo annotations should be allowed.
Categories
Steps to Reproduce
Screenshots
The friendToSave object being passed into the saveNewFriend method: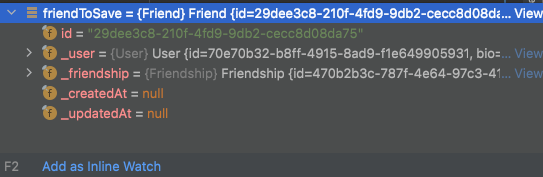
Above, you can see that both the User field and the Friendship field is populated in the Friend object.
The request object from 'LINE A' above: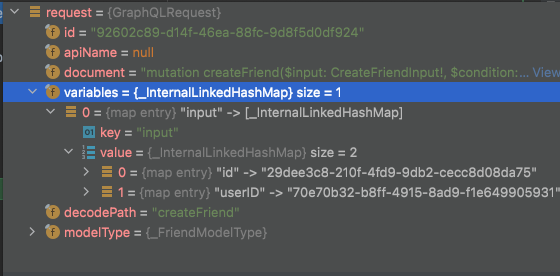
After going through the
ModelMutation.create()
function, it drops the friendshipID field and the only inputs remaining are the ID from the Friendship, and the ID of the user field.Here's the response of the call followed by the error: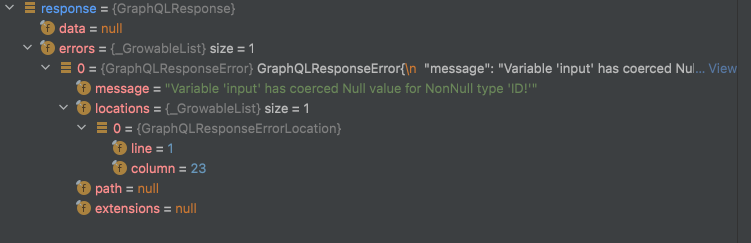
Platforms
Android Device/Emulator API Level
API 32+
Environment
Dependencies
Device
N/A
OS
N/A
Deployment Method
Amplify CLI
CLI Version
9.2.1
Additional Context
No response
Amplify Config
{ "UserAgent": "aws-amplify-cli/2.0", "Version": "1.0", "api": { "plugins": { "awsAPIPlugin": { "": {
"endpointType": "GraphQL",
"endpoint": "",
"region": "us-east-2",
"authorizationType": "AMAZON_COGNITO_USER_POOLS"
}
}
}
},
"auth": {
"plugins": {
"awsCognitoAuthPlugin": {
"UserAgent": "aws-amplify-cli/0.1.0",
"Version": "0.1.0",
"IdentityManager": {
"Default": {}
},
"CredentialsProvider": {
"CognitoIdentity": {
"Default": {
"PoolId": "",
"Region": "us-east-2"
}
}
},
"CognitoUserPool": {
"Default": {
"PoolId": "",
"AppClientId": "",
"Region": "us-east-2"
}
},
"Auth": {
"Default": {
"OAuth": {
"WebDomain": "",
"AppClientId": "",
"SignInRedirectURI": "",
"SignOutRedirectURI": "",
"Scopes": [
"phone",
"email",
"openid",
"profile",
"aws.cognito.signin.user.admin"
]
},
"authenticationFlowType": "USER_SRP_AUTH",
"socialProviders": [
"FACEBOOK",
"GOOGLE"
],
"usernameAttributes": [
"EMAIL"
],
"signupAttributes": [
"EMAIL",
"NAME"
],
"passwordProtectionSettings": {
"passwordPolicyMinLength": 8,
"passwordPolicyCharacters": []
},
"mfaConfiguration": "OPTIONAL",
"mfaTypes": [
"SMS"
],
"verificationMechanisms": [
"EMAIL"
]
}
},
"AppSync": {
"Default": {
"ApiUrl": "",
"Region": "us-east-2",
"AuthMode": "AMAZON_COGNITO_USER_POOLS",
"ClientDatabasePrefix": "_AMAZON_COGNITO_USER_POOLS"
},
"_AWS_IAM": {
"ApiUrl": "",
"Region": "us-east-2",
"AuthMode": "AWS_IAM",
"ClientDatabasePrefix": "_AWS_IAM"
}
},
"S3TransferUtility": {
"Default": {
"Bucket": "",
"Region": "us-east-2"
}
}
}
}
},
"storage": {
"plugins": {
"awsS3StoragePlugin": {
"bucket": "",
"region": "us-east-2",
"defaultAccessLevel": "guest"
}
}
}
}