Closed koterthecoder closed 4 years ago
@koterthecoder as you said, our console is able to connect to your master as the second viewer. Since our console uses the same signaling protocol, the problem seems to be not from the SDK or backend. I'm afraid that there's a logic error in your application.
Could you confirm if the signaling is already opened before you start sending an offer? If you don't mind, could you share a snippet of your code and a stack trace?
Thank you for your quick reply.
I do believe that the signaling channel is already open (before sending first offer) since another viewer is already viewing the stream.
Here is my viewer code and stack trace. This viewer is based off of the ReactJs code provided in this comment. There is only one real difference, which is that i get the channelArn on the backend and pass it into the signaling client here.
viewer-stream-component.js
import React from 'react';
import AWS from "aws-sdk";
import { SignalingClient, Role } from 'amazon-kinesis-video-streams-webrtc'
import { Row, Col, Button, Badge } from 'reactstrap'
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome'
import { faHeart, faExclamationTriangle, faUser } from '@fortawesome/free-solid-svg-icons'
const viewer = {};
function uid() {
return Math.random().toString(36).substring(2, 15) + Math.random().toString(36).substring(2, 15);
}
class LiveFeedView extends React.Component {
constructor(props) {
super(props);
this.videoRef = React.createRef()
console.log( this.props )
this.state = { isLiked: this.props.isLiked }
}
componentWillUnmount() {
console.log('[VIEWER] Stopping viewer connection');
if (viewer.signalingClient) {
viewer.signalingClient.close();
viewer.signalingClient = null;
}
if (viewer.peerConnection) {
viewer.peerConnection.close();
viewer.peerConnection = null;
}
if (viewer.remoteStream) {
viewer.remoteStream.getTracks().forEach(track => track.stop());
viewer.remoteStream = null;
}
if (viewer.peerConnectionStatsInterval) {
clearInterval(viewer.peerConnectionStatsInterval);
viewer.peerConnectionStatsInterval = null;
}
if (viewer.remoteView) {
viewer.remoteView.srcObject = null;
}
if (viewer.dataChannel) {
viewer.dataChannel = null;
}
}
async componentDidMount() {
// Create KVS client
const kinesisVideoClient = new AWS.KinesisVideo({
region: this.props.formValues.region,
accessKeyId: this.props.formValues.accessKeyId,
secretAccessKey: this.props.formValues.secretAccessKey,
sessionToken: this.props.formValues.sessionToken,
endpoint: this.props.formValues.endpoint,
});
// Get signaling channel ARN
// const describeSignalingChannelResponse = await kinesisVideoClient.describeSignalingChannel({ ChannelName: this.props.formValues.channelName}).promise();
// const channelARN = describeSignalingChannelResponse.ChannelInfo.ChannelARN;
const channelARN = this.props.peer.channelArn
console.log('[VIEWER] Channel ARN: ', channelARN);
// Get signaling channel endpoints
const getSignalingChannelEndpointResponse = await kinesisVideoClient.getSignalingChannelEndpoint({ ChannelARN: channelARN,
SingleMasterChannelEndpointConfiguration: {
Protocols: ['WSS', 'HTTPS'],
Role: Role.VIEWER,
},
}).promise();
const endpointsByProtocol = getSignalingChannelEndpointResponse.ResourceEndpointList.reduce((endpoints, endpoint) => {
endpoints[endpoint.Protocol] = endpoint.ResourceEndpoint;
return endpoints;
}, {});
console.log('[VIEWER] Endpoints: ', endpointsByProtocol);
const kinesisVideoSignalingChannelsClient = new AWS.KinesisVideoSignalingChannels({
region: this.props.formValues.region,
accessKeyId: this.props.formValues.accessKeyId,
secretAccessKey: this.props.formValues.secretAccessKey,
sessionToken: this.props.formValues.sessionToken,
endpoint: endpointsByProtocol.HTTPS,
});
// Get ICE server configuration
const getIceServerConfigResponse = await kinesisVideoSignalingChannelsClient.getIceServerConfig({
ChannelARN: channelARN,
}).promise();
const iceServers = [];
iceServers.push({ urls: `stun:stun.kinesisvideo.${this.props.formValues.region}.amazonaws.com:443` });
//if (!formValues.natTraversalDisabled) {
getIceServerConfigResponse.IceServerList.forEach(iceServer =>
iceServers.push({
urls: iceServer.Uris,
username: iceServer.Username,
credential: iceServer.Password,
}),
);
//}
console.log('[VIEWER] ICE servers: ', iceServers);
// Create Signaling Client
viewer.signalingClient = new SignalingClient({
channelARN,
channelEndpoint: endpointsByProtocol.WSS,
clientId: uid(),
role: Role.VIEWER,
region: this.props.formValues.region,
credentials: {
accessKeyId: this.props.formValues.accessKeyId,
secretAccessKey: this.props.formValues.secretAccessKey,
},
});
const configuration = {
iceServers,
iceTransportPolicy: 'all',
};
viewer.peerConnection = new RTCPeerConnection(configuration);
viewer.signalingClient.on('open', async () => {
console.log('[VIEWER] Connected to signaling service');
// Create an SDP offer to send to the master
console.log('[VIEWER] Creating SDP offer');
await viewer.peerConnection.setLocalDescription(
await viewer.peerConnection.createOffer({
offerToReceiveAudio: true,
offerToReceiveVideo: true,
}),
);
// When trickle ICE is enabled, send the offer now and then send ICE candidates as they are generated. Otherwise wait on the ICE candidates.
console.log('[VIEWER] Sending SDP offer');
viewer.signalingClient.sendSdpOffer(viewer.peerConnection.localDescription);
console.log('[VIEWER] Generating ICE candidates');
});
viewer.signalingClient.on('sdpAnswer', async answer => {
// Add the SDP answer to the peer connection
console.log('[VIEWER] Received SDP answer');
await viewer.peerConnection.setRemoteDescription(answer);
});
viewer.signalingClient.on('iceCandidate', candidate => {
// Add the ICE candidate received from the MASTER to the peer connection
console.log('[VIEWER] Received ICE candidate');
viewer.peerConnection.addIceCandidate(candidate);
});
viewer.signalingClient.on('close', () => {
console.log('[VIEWER] Disconnected from signaling channel');
});
viewer.signalingClient.on('error', error => {
console.error('[VIEWER] Signaling client error: ', error);
});
// Send any ICE candidates to the other peer
viewer.peerConnection.addEventListener('icecandidate', ({ candidate }) => {
if (candidate) {
console.log('[VIEWER] Generated ICE candidate');
// When trickle ICE is enabled, send the ICE candidates as they are generated.
console.log('[VIEWER] Sending ICE candidate');
console.log({ candidate })
viewer.signalingClient.sendIceCandidate(candidate);
} else {
console.log('[VIEWER] All ICE candidates have been generated');
}
});
// As remote tracks are received, add them to the remote view
viewer.peerConnection.addEventListener('track', async (event) => {
console.log('[VIEWER] Received remote track');
// if (remoteView.srcObject) {
// return;
// }
viewer.remoteStream = event.streams[0];
//this.setState({streamURL: event.streams[0]});
this.videoRef.current.srcObject = event.streams[0];
});
console.log('[VIEWER] Starting viewer connection');
viewer.signalingClient.open();
}
triggerLikeAction = () => {
const { peer } = this.props
const { profileId } = peer
const newValue = !this.state.isLiked
console.log({ newValue })
this.setState({ isLiked: newValue })
this.props.likeUser(profileId, newValue)
}
triggerReportAction = () => {
console.log('reporting user')
const { peer } = this.props
const { profileId } = peer
this.props.reportUser(profileId)
}
peerControls = () => {
const { peer, hostProfileId } = this.props
const { displayName, profileId } = peer
console.log({ displayName, profileId })
const formattedDisplayName = displayName.length > 10 ? `${displayName.substring(0, 9)}...` : displayName
console.log({ isLiked: this.state.isLiked })
return (
<Row style={{ position: 'relative', top: '40px', margin: 0, zIndex: 1000 }}>
<Col >
{ displayName && <Badge color="dark" className="mb-2" >{ formattedDisplayName }</Badge>}
</Col>
<Col className="text-right" >
{ profileId && <FontAwesomeIcon color={ this.state.isLiked ? 'red' : 'white' } icon={faHeart} onClick={this.triggerLikeAction} className="mr-2" size="2x" /> }
{ profileId && <FontAwesomeIcon icon={faExclamationTriangle} onClick={this.triggerReportAction} size="2x" /> }
</Col>
</Row>
)
}
render() {
return (
<>
{ this.peerControls() }
<video ref={this.videoRef} width="100%" autoPlay playsInline style={{ margin: '0px', borderRadius: '10px' }} />
</>
)
}
}
function ViewerStreamComponent({ index, peer, reportUser, likeUser, hostProfileId, isLiked }) {
const opts = {
accessKeyId: 'xxx',
secretAccessKey: 'xxx',
region: 'us-east-1',
channelName: '<your channel>'
}
return (
<div >
<LiveFeedView formValues={opts} index={index} peer={peer} reportUser={reportUser} likeUser={likeUser} hostProfileId={hostProfileId} isLiked={isLiked} ></LiveFeedView>
</div>
)
}
export default ViewerStreamComponent
stack trace
| SignalingClient.sendMessage | @ | SignalingClient.ts:199
-- | -- | -- | --
| SignalingClient.sendSdpOffer | @ | SignalingClient.ts:168
| (anonymous) | @ | index.js:149
| async function (async) | |
| (anonymous) | @ | index.js:140
| emit | @ | events.js:146
| SignalingClient.onOpen | @ | SignalingClient.ts:231
| sentryWrapped | @ | helpers.ts:85
There shouldn't be any issue with passing down channel ARN from a higher level component as long as you're still using the same channel ARN. Could you also share the logs? Also, if you don't mind, could you also share your channel ARN?
The signaling channels are created with a ChannelName which correlates to a user's profileId. The following channel ARN is from a scenario I have just tested with: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
.
Here are the console logs for a client that encountered the error...
instrument.ts:129 [VIEWER] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/8bbc261157c079fe24d34cbb95ed5078f4616a40/1593445332905
instrument.ts:129 [VIEWER] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
instrument.ts:129 [VIEWER] Endpoints: {HTTPS: "https://r-13e2a5fc.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-ef08a1db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER] Endpoints: {HTTPS: "https://r-b0e0aa33.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-d622a7db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER] Starting viewer connection
instrument.ts:129 [VIEWER] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER] Starting viewer connection
instrument.ts:129 [MASTER] Received SDP offer from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
3instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Connected to signaling service
instrument.ts:129 [VIEWER] Creating SDP offer
instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Creating SDP answer for client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Sending SDP offer
SignalingClient.ts:199 Uncaught (in promise) Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendSdpOffer (SignalingClient.ts:168)
at SignalingClient.<anonymous> (index.js:149)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendSdpOffer @ SignalingClient.ts:168
(anonymous) @ index.js:149
async function (async)
(anonymous) @ index.js:140
emit @ events.js:146
SignalingClient.onOpen @ SignalingClient.ts:231
sentryWrapped @ helpers.ts:85
instrument.ts:129 [MASTER] Sending SDP answer to client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Generating ICE candidates for client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Connected to signaling service
instrument.ts:129 [VIEWER] Creating SDP offer
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [MASTER] Generated ICE candidate for client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Sending ICE candidate to client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Generated ICE candidate for client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Sending ICE candidate to client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Sending SDP offer
instrument.ts:129 [VIEWER] Generating ICE candidates
2instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
12instrument.ts:129 [MASTER] Received ICE candidate from client: ou3pwmlmbjnre1i25qmuci
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Generated ICE candidate
instrument.ts:129 [VIEWER] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER] Received SDP answer
4instrument.ts:129 [VIEWER] Received ICE candidate
4instrument.ts:129 [VIEWER] Received ICE candidate
instrument.ts:129 [MASTER] Received SDP offer from client: mu8s84dnrdernxaaeljkg
2instrument.ts:129 [VIEWER] Received remote track
2instrument.ts:129 [VIEWER] Received ICE candidate
15instrument.ts:129 [MASTER] Received ICE candidate from client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [VIEWER] Received ICE candidate
instrument.ts:129 [VIEWER] All ICE candidates have been generated
instrument.ts:129 [MASTER] Received ICE candidate from client: mu8s84dnrdernxaaeljkg
3instrument.ts:129 [VIEWER] Received ICE candidate
instrument.ts:129 [MASTER] Creating SDP answer for client: mu8s84dnrdernxaaeljkg
38instrument.ts:129 [MASTER] Received ICE candidate from client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Sending SDP answer to client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Generating ICE candidates for client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Generated ICE candidate for client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Sending ICE candidate to client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Generated ICE candidate for client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Sending ICE candidate to client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Generated ICE candidate for client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Sending ICE candidate to client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Generated ICE candidate for client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] Sending ICE candidate to client: mu8s84dnrdernxaaeljkg
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: mu8s84dnrdernxaaeljkg
So, from what I can see, you have 3 peers: 1 master and 2 viewers. Your first viewer seems to be connected and able to send/receive signaling messages. But, your second viewer, somehow, got connected after it tried to send an offer, which became a failure.
Could you confirm if I interpreted the log accurately? Also, if correct, could you mark the viewers with a unique id so that we can differentiate the logs?
Your interpretation is accurate. I marked each viewer log with the viewerId for the particular viewer
instrument.ts:129 [MASTER] Starting master connection
instrument.ts:129 [MASTER] Connected to signaling service
instrument.ts:129 {request: {…}}
instrument.ts:129 {startSessionResponse: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 addPeers {oldSet: Array(0), newSet: Array(3)}
instrument.ts:129 {friends: Array(1)}
instrument.ts:129 {friends: Array(1)}
instrument.ts:129 {formValues: {…}, index: 0, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {formValues: {…}, index: 0, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {formValues: {…}, index: 1, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {formValues: {…}, index: 1, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/8bbc261157c079fe24d34cbb95ed5078f4616a40/1593445332905
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Endpoints: {HTTPS: "https://r-b0e0aa33.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-d622a7db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Endpoints: {HTTPS: "https://r-13e2a5fc.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-ef08a1db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Starting viewer connection
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Starting viewer connection
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Connected to signaling service
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Creating SDP offer
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Sending SDP offer
SignalingClient.ts:199 Uncaught (in promise) Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendSdpOffer (SignalingClient.ts:168)
at SignalingClient.<anonymous> (index.js:148)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendSdpOffer @ SignalingClient.ts:168
(anonymous) @ index.js:148
async function (async)
(anonymous) @ index.js:139
emit @ events.js:146
SignalingClient.onOpen @ SignalingClient.ts:231
sentryWrapped @ helpers.ts:85
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Connected to signaling service
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Creating SDP offer
instrument.ts:129 [MASTER] Received SDP offer from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Received ICE candidate from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending SDP offer
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generating ICE candidates
3instrument.ts:129 [MASTER] Received ICE candidate from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [MASTER] Received ICE candidate from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Creating SDP answer for client: xq6yj0f71kksnjngjigq49
6instrument.ts:129 [MASTER] Received ICE candidate from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Sending SDP answer to client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Generating ICE candidates for client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Received ICE candidate from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Generated ICE candidate for client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Sending ICE candidate to client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Generated ICE candidate for client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Sending ICE candidate to client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Generated ICE candidate for client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Sending ICE candidate to client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Generated ICE candidate for client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] Sending ICE candidate to client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Generated ICE candidate
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Sending ICE candidate
instrument.ts:129 {candidate: RTCIceCandidate}
4instrument.ts:129 [MASTER] Received ICE candidate from client: xq6yj0f71kksnjngjigq49
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Received SDP answer
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Received ICE candidate
4instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Received ICE candidate
2instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Received remote track
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] All ICE candidates have been generated
instrument.ts:129 [MASTER] Received SDP offer from client: n53eyx6wog0xj21sm8rfd
40instrument.ts:129 [MASTER] Received ICE candidate from client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Creating SDP answer for client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Sending SDP answer to client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Generating ICE candidates for client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Generated ICE candidate for client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Sending ICE candidate to client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Generated ICE candidate for client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] Sending ICE candidate to client: n53eyx6wog0xj21sm8rfd
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: n53eyx6wog0xj21sm8rfd
2instrument.ts:129 [MASTER] Received ICE candidate from client: n53eyx6wog0xj21sm8rfd
@koterthecoder thanks for details! So, it looks like either your first/second viewer didn't use the right channel arn, they both should have the same channel arn.
But, instead, your logs show the following instead:
instrument.ts:129 [VIEWER 8v14e9g0rrmbatk3qzl3bl] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
instrument.ts:129 [VIEWER gwob57xgojsj05j9mrd7r] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/8bbc261157c079fe24d34cbb95ed5078f4616a40/1593445332905
@lherman-cs no problem!
For this particular client (of the posted logs), there should be two separate viewing windows for the two separate signaling channels. The client should be displaying the feeds for the other two peers, and essentially provide a three-way video conference.
The following scenario is the expected user experience
1) Device A
connects as master to Channel A
and is waiting for peers
2) Device B
connects as master to Channel B
then connects as viewer to Channel A
3) Device A
connects as viewer to Channel B
At this point there should be a two-way video conference between Device A and Device B
4) Device C
connects as master to Channel C
then connects as viewer to Channel A
and connects as viewer to Channel B
5) Device A
connects as viewer to Channel C
6) Device B
connects as viewer to Channel C
Now at this point there should be a three-way conference between Device A, Device B, and Device C, where each device is viewing video feeds from two independent signaling channels.
Out of that list of steps, I am only encountering issues with step 4. Steps 5 and 6 are experienced successfully and can view all relevant video feeds, even though Device C
encountered the error I initially posted.
Your architecture should be OK. Even if a viewer starts before master, each message has a TTL and our backend will keep the messages until the master is ready.
As you mentioned that steps 5 and 6 are fine, I think this is because Device C
could connect as master to Channel C
. However, it somehow failed to connect to either Channel A
or Channel B
as a viewer. This is because Device C
failed on sending the sdp offer (only viewers that send sdp offers).
What I really don't understand is that, "open" event is only fired after the connection state has changed to ready, https://github.com/awslabs/amazon-kinesis-video-streams-webrtc-sdk-js/blob/master/src/SignalingClient.ts#L231-L232. So, the only possibility that I can think of is that somehow the connection state changed to non-ready before sending sdp offer but after on open callback is called, https://github.com/awslabs/amazon-kinesis-video-streams-webrtc-sdk-js/blob/master/examples/viewer.js#L110-L141.
However, if that's the case, there are 3 other states: CLOSED
, CLOSING
, and CONNECTING
. If the state changed to CLOSED
, we would emit a close event and your application would print out a closing message, but we don't see this message. If the state changed to CONNECTING
, this transition only occurs when you call open, and you can call open again if and only if the state has changed to CLOSED
, but since we don't see a closing message, this is not possible either. Lastly, it's not possible to transition to CONNECTING
directly from CONNECTED
, similar reason to CLOSING
.
I'm sorry, but I'm really puzzled. Could you also send the logs from all of your devices with markers to differentiate them?
@lherman-cs No need for apologies, I really appreciate your help here, for I too am quite puzzled by this. Also one thing to note, I believe there could potentially be a race condition here. Because there was one time where three clients were conferencing successfully, but this only happened once in the time this applications has been in development.
Below are the different logs for three separate simultaneous clients running on: Chrome, Firefox, and Brave.
Device A (Brave)
instrument.ts:129 [MASTER] Starting master connection
instrument.ts:129 [MASTER] Connected to signaling service
instrument.ts:129 {request: {…}}
instrument.ts:129 {startSessionResponse: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 addPeers {oldSet: Array(0), newSet: Array(1)}
instrument.ts:129 {friends: Array(0)}
instrument.ts:129 {friends: Array(0)}
instrument.ts:129 ws message
instrument.ts:129 newPeerHandler {msg: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {friends: Array(0)}
instrument.ts:129 {friends: Array(0)}
instrument.ts:129 {formValues: {…}, index: 0, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {formValues: {…}, index: 0, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
instrument.ts:129 addPeers {oldSet: Array(1), newSet: Array(2)}
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Endpoints: {HTTPS: "https://r-b0e0aa33.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-d622a7db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Starting viewer connection
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Connected to signaling service
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Creating SDP offer
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending SDP offer
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generating ICE candidates
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received SDP answer
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
2instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received remote track
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Generated ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Sending ICE candidate
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] All ICE candidates have been generated
4instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
instrument.ts:129 [MASTER] Received SDP offer from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
3instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Creating SDP answer for client: jvwaxmj0fcwbkcmja0y9b
2instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Sending SDP answer to client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Generating ICE candidates for client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Generated ICE candidate for client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Sending ICE candidate to client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Generated ICE candidate for client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] Sending ICE candidate to client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: jvwaxmj0fcwbkcmja0y9b
2instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
2instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
2instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
2instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
3instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
2instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
2instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
7instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 [VIEWER vz0waux1x9clg1z5xp6my] Received ICE candidate
17instrument.ts:129 [MASTER] Received ICE candidate from client: jvwaxmj0fcwbkcmja0y9b
instrument.ts:129 ws message
instrument.ts:129 newPeerHandler {msg: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {friends: Array(0)}
instrument.ts:129 {friends: Array(0)}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {formValues: {…}, index: 1, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {formValues: {…}, index: 1, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {displayName: "dko", profileId: "822a28567ae513bcfef4c3ad98f2ae33f7a54c1c"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "dko", profileId: "822a28567ae513bcfef4c3ad98f2ae33f7a54c1c"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/822a28567ae513bcfef4c3ad98f2ae33f7a54c1c/1593368624057
instrument.ts:129 addPeers {oldSet: Array(2), newSet: Array(3)}
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Endpoints: {HTTPS: "https://r-0606824a.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-4424355c.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] ICE servers: (2) [{…}, {…}]
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Starting viewer connection
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Connected to signaling service
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Creating SDP offer
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending SDP offer
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generating ICE candidates
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Received SDP answer
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Generated ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Sending ICE candidate
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Received ICE candidate
2instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Received remote track
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] All ICE candidates have been generated
instrument.ts:129 [VIEWER iqf37x3k1e6cs0fhiijp] Received ICE candidate
Device 2 (Firefox)
[MASTER] Starting master connection instrument.ts:129
[MASTER] Connected to signaling service instrument.ts:129
Object { request: {…} }
instrument.ts:129
Object { startSessionResponse: {…} }
instrument.ts:129
Object { initProfile: {…} }
instrument.ts:129
Object { initProfile: {…} }
instrument.ts:129
Object { profile: {…} }
instrument.ts:129
Object { profile: {…} }
instrument.ts:129
addPeers
Object { oldSet: [], newSet: (2) […] }
instrument.ts:129
Object { friends: [] }
instrument.ts:129
Object { friends: [] }
instrument.ts:129
Object { formValues: {…}, index: 0, peer: {…}, reportUser: reportUser(profileId), likeUser: likeUser(profileId, value)
, hostProfileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d", isLiked: false }
instrument.ts:129
Object { formValues: {…}, index: 0, peer: {…}, reportUser: reportUser(profileId), likeUser: likeUser(profileId, value)
, hostProfileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d", isLiked: false }
instrument.ts:129
Object { displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40" }
instrument.ts:129
Object { isLiked: false }
instrument.ts:129
Object { displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40" }
instrument.ts:129
Object { isLiked: false }
instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/8bbc261157c079fe24d34cbb95ed5078f4616a40/1593445332905 instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Endpoints:
Object { HTTPS: "https://r-13e2a5fc.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-ef08a1db.kinesisvideo.us-east-1.amazonaws.com" }
instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] ICE servers:
Array(3) [ {…}, {…}, {…} ]
instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:132
WebRTC: Using five or more STUN/TURN servers causes problems 3 index.js:132
[VIEWER jvwaxmj0fcwbkcmja0y9b] Starting viewer connection instrument.ts:129
[MASTER] Received SDP offer from client: vz0waux1x9clg1z5xp6my instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:165
WebRTC: Using five or more STUN/TURN servers causes problems 3 index.js:165
[MASTER] Received ICE candidate from client: vz0waux1x9clg1z5xp6my 2 instrument.ts:129
[MASTER] Creating SDP answer for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending SDP answer to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generating ICE candidates for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Received ICE candidate from client: vz0waux1x9clg1z5xp6my 2 instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Received ICE candidate from client: vz0waux1x9clg1z5xp6my 7 instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Connected to signaling service instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Creating SDP offer instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending SDP offer instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generating ICE candidates instrument.ts:129
[MASTER] Received ICE candidate from client: vz0waux1x9clg1z5xp6my instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Received ICE candidate from client: vz0waux1x9clg1z5xp6my instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[MASTER] Received ICE candidate from client: vz0waux1x9clg1z5xp6my 2 instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Generated ICE candidate for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] Sending ICE candidate to client: vz0waux1x9clg1z5xp6my instrument.ts:129
[MASTER] All ICE candidates have been generated for client: vz0waux1x9clg1z5xp6my instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Received SDP answer instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Received remote track 2 instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Generated ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Sending ICE candidate instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] All ICE candidates have been generated instrument.ts:129
[VIEWER jvwaxmj0fcwbkcmja0y9b] Received ICE candidate 2 instrument.ts:129
ws message instrument.ts:129
newPeerHandler
Object { msg: {…} }
instrument.ts:129
Object { initProfile: {…} }
instrument.ts:129
Object { initProfile: {…} }
instrument.ts:129
Object { profile: {…} }
instrument.ts:129
Object { profile: {…} }
instrument.ts:129
Object { friends: [] }
instrument.ts:129
Object { friends: [] }
instrument.ts:129
Object { displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40" }
instrument.ts:129
Object { isLiked: false }
instrument.ts:129
Object { displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40" }
instrument.ts:129
Object { isLiked: false }
instrument.ts:129
Object { formValues: {…}, index: 1, peer: {…}, reportUser: reportUser(profileId), likeUser: likeUser(profileId, value)
, hostProfileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d", isLiked: false }
instrument.ts:129
Object { formValues: {…}, index: 1, peer: {…}, reportUser: reportUser(profileId), likeUser: likeUser(profileId, value)
, hostProfileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d", isLiked: false }
instrument.ts:129
Object { displayName: "dko", profileId: "822a28567ae513bcfef4c3ad98f2ae33f7a54c1c" }
instrument.ts:129
Object { isLiked: false }
instrument.ts:129
Object { displayName: "dko", profileId: "822a28567ae513bcfef4c3ad98f2ae33f7a54c1c" }
instrument.ts:129
Object { isLiked: false }
instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/822a28567ae513bcfef4c3ad98f2ae33f7a54c1c/1593368624057 instrument.ts:129
addPeers
Object { oldSet: (2) […], newSet: (3) […] }
instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Endpoints:
Object { HTTPS: "https://r-0606824a.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-4424355c.kinesisvideo.us-east-1.amazonaws.com" }
instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] ICE servers:
Array [ {…}, {…} ]
instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:132
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Starting viewer connection instrument.ts:129
[MASTER] Received SDP offer from client: tswptwdh753s7wqcfahwj instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:165
WebRTC: Using five or more STUN/TURN servers causes problems 3 index.js:165
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Creating SDP answer for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj 3 instrument.ts:129
[MASTER] Sending SDP answer to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generating ICE candidates for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj 6 instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj 3 instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Connected to signaling service instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Creating SDP offer instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending SDP offer instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generating ICE candidates instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj 2 instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Received SDP answer instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Received ICE candidate 2 instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Received remote track 2 instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Generated ICE candidate for client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] Sending ICE candidate to client: tswptwdh753s7wqcfahwj instrument.ts:129
[MASTER] All ICE candidates have been generated for client: tswptwdh753s7wqcfahwj instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Generated ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] Sending ICE candidate instrument.ts:129
[VIEWER c8l2la8dpzbtb9gj5ctt6o] All ICE candidates have been generated instrument.ts:129
[MASTER] Received ICE candidate from client: tswptwdh753s7wqcfahwj 2 instrument.ts:129
Device C (Chrome)
instrument.ts:129 [MASTER] Starting master connection
instrument.ts:129 [MASTER] Connected to signaling service
instrument.ts:129 {request: {…}}
instrument.ts:129 {startSessionResponse: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {initProfile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 {profile: {…}}
instrument.ts:129 addPeers {oldSet: Array(0), newSet: Array(3)}
instrument.ts:129 {friends: Array(1)}
instrument.ts:129 {friends: Array(1)}
instrument.ts:129 {formValues: {…}, index: 0, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {formValues: {…}, index: 0, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "Benji", profileId: "8bbc261157c079fe24d34cbb95ed5078f4616a40"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {formValues: {…}, index: 1, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {formValues: {…}, index: 1, peer: {…}, reportUser: ƒ, likeUser: ƒ, …}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 {displayName: "fir", profileId: "ea12e0aa3956ab5a87954004a8f7b416ca12564d"}
instrument.ts:129 {isLiked: false}
instrument.ts:129 [VIEWER c0uo23kuo56h3rqs0nfbkg] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/8bbc261157c079fe24d34cbb95ed5078f4616a40/1593445332905
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
instrument.ts:129 [VIEWER c0uo23kuo56h3rqs0nfbkg] Endpoints: {HTTPS: "https://r-13e2a5fc.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-ef08a1db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Endpoints: {HTTPS: "https://r-b0e0aa33.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-d622a7db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER c0uo23kuo56h3rqs0nfbkg] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER c0uo23kuo56h3rqs0nfbkg] Starting viewer connection
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Starting viewer connection
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Connected to signaling service
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Creating SDP offer
instrument.ts:129 [VIEWER c0uo23kuo56h3rqs0nfbkg] Connected to signaling service
instrument.ts:129 [VIEWER c0uo23kuo56h3rqs0nfbkg] Creating SDP offer
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending SDP offer
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generating ICE candidates
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
index.js:150 Uncaught (in promise) DOMException: Failed to execute 'setLocalDescription' on 'RTCPeerConnection': Failed to set local offer sdp: The order of m-lines in subsequent offer doesn't match order from previous offer/answer.
async function (async)
(anonymous) @ index.js:139
emit @ events.js:146
SignalingClient.onOpen @ SignalingClient.ts:231
sentryWrapped @ helpers.ts:85
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [MASTER] Received SDP offer from client: iqf37x3k1e6cs0fhiijp
3instrument.ts:129 [MASTER] Received ICE candidate from client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Creating SDP answer for client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Sending SDP answer to client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Generating ICE candidates for client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Generated ICE candidate for client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Sending ICE candidate to client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Generated ICE candidate for client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] Sending ICE candidate to client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: iqf37x3k1e6cs0fhiijp
5instrument.ts:129 [MASTER] Received ICE candidate from client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received SDP answer
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Generated ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Sending ICE candidate
2instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received remote track
instrument.ts:129 [MASTER] Received ICE candidate from client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received ICE candidate
instrument.ts:129 [MASTER] Received ICE candidate from client: iqf37x3k1e6cs0fhiijp
3instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received ICE candidate
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] All ICE candidates have been generated
instrument.ts:129 [MASTER] Received ICE candidate from client: iqf37x3k1e6cs0fhiijp
3instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received ICE candidate
4instrument.ts:129 [MASTER] Received ICE candidate from client: iqf37x3k1e6cs0fhiijp
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received ICE candidate
instrument.ts:129 [MASTER] Received SDP offer from client: c8l2la8dpzbtb9gj5ctt6o
6instrument.ts:129 [MASTER] Received ICE candidate from client: c8l2la8dpzbtb9gj5ctt6o
2instrument.ts:129 [MASTER] Received ICE candidate from client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received ICE candidate
3instrument.ts:129 [MASTER] Received ICE candidate from client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Creating SDP answer for client: c8l2la8dpzbtb9gj5ctt6o
6instrument.ts:129 [MASTER] Received ICE candidate from client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Sending SDP answer to client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Generating ICE candidates for client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Generated ICE candidate for client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Sending ICE candidate to client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Generated ICE candidate for client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] Sending ICE candidate to client: c8l2la8dpzbtb9gj5ctt6o
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: c8l2la8dpzbtb9gj5ctt6o
5instrument.ts:129 [MASTER] Received ICE candidate from client: c8l2la8dpzbtb9gj5ctt6o
5instrument.ts:129 [VIEWER tswptwdh753s7wqcfahwj] Received ICE candidate
13instrument.ts:129 [MASTER] Received ICE candidate from client: c8l2la8dpzbtb9gj5ctt6o
From this log, I only see 1 issue in your Device C
, it failed to setLocalDescription
which seems to be related to a browser compatibility issue. Since it failed, it didn't send the offer to master. Therefore, Device C
was not able to connect to Channel A
or Channel B
.
Could you try to use https://github.com/webrtcHacks/adapter? I hope that it can solve this issue. Also, to isolate the issue further, can you use Firefox/Brave for Device C
?
I installed webrtc-adapter
and imported it in my project, and am now getting a different error for Chrome
Uncaught (in promise) DOMException: Failed to execute 'setRemoteDescription' on 'RTCPeerConnection': Failed to set remote answer sdp: Called in wrong state: kStable
Firefox Device C
Console Logs
[MASTER] Starting master connection instrument.ts:129
[MASTER] Connected to signaling service instrument.ts:129
[VIEWER dnkffuuofq6qubytoliblo] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/822a28567ae513bcfef4c3ad98f2ae33f7a54c1c/1593368624057 instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/8bbc261157c079fe24d34cbb95ed5078f4616a40/1593445332905 instrument.ts:129
[VIEWER dnkffuuofq6qubytoliblo] Endpoints:
Object { HTTPS: "https://r-0606824a.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-4424355c.kinesisvideo.us-east-1.amazonaws.com" }
instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Endpoints:
Object { HTTPS: "https://r-13e2a5fc.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-ef08a1db.kinesisvideo.us-east-1.amazonaws.com" }
instrument.ts:129
[VIEWER dnkffuuofq6qubytoliblo] ICE servers:
Array(3) [ {…}, {…}, {…} ]
instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:132
WebRTC: Using five or more STUN/TURN servers causes problems 3 index.js:132
[VIEWER dnkffuuofq6qubytoliblo] Starting viewer connection instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] ICE servers:
Array(3) [ {…}, {…}, {…} ]
instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:132
WebRTC: Using five or more STUN/TURN servers causes problems 3 index.js:132
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Starting viewer connection instrument.ts:129
[MASTER] Received SDP offer from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery index.js:165
WebRTC: Using more than two STUN/TURN servers slows down discovery index.js:165
WebRTC: Using five or more STUN/TURN servers causes problems index.js:165
WebRTC: Using five or more STUN/TURN servers causes problems index.js:165
WebRTC: Using five or more STUN/TURN servers causes problems index.js:165
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i 2 instrument.ts:129
[MASTER] Creating SDP answer for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending SDP answer to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generating ICE candidates for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i 3 instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Received SDP offer from client: bil4pu8adrafh5gegnag4h instrument.ts:129
WebRTC: Using more than two STUN/TURN servers slows down discovery 2 index.js:165
WebRTC: Using five or more STUN/TURN servers causes problems 3 index.js:165
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h 2 instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h 2 instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Creating SDP answer for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i 6 instrument.ts:129
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i 3 instrument.ts:129
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h 3 instrument.ts:129
[VIEWER dnkffuuofq6qubytoliblo] Connected to signaling service instrument.ts:129
[VIEWER dnkffuuofq6qubytoliblo] Creating SDP offer instrument.ts:129
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h 2 instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending SDP answer to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generating ICE candidates for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[VIEWER dnkffuuofq6qubytoliblo] Sending SDP offer instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
Error: Could not send message because the connection to the signaling service is not open. SignalingClient.ts:199
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Connected to signaling service instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Creating SDP offer instrument.ts:129
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h 9 instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending SDP offer instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generating ICE candidates instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Received SDP answer instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Received remote track 2 instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Received ICE candidate 2 instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Generated ICE candidate for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Sending ICE candidate to client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] All ICE candidates have been generated for client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Generated ICE candidate for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] Sending ICE candidate to client: bil4pu8adrafh5gegnag4h instrument.ts:129
[MASTER] All ICE candidates have been generated for client: bil4pu8adrafh5gegnag4h instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Generated ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] Sending ICE candidate instrument.ts:129
[VIEWER hdgydq4wkvgsr3l4lmvuwe] All ICE candidates have been generated instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i 2 instrument.ts:129
[MASTER] Received ICE candidate from client: bil4pu8adrafh5gegnag4h 3 instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
[MASTER] Received ICE candidate from client: c27qontwjiunxhzgkrrp8i instrument.ts:129
Brave Device C
Console Logs
instrument.ts:129 [MASTER] Connected to signaling service
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/ea12e0aa3956ab5a87954004a8f7b416ca12564d/1593464623023
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Channel ARN: arn:aws:kinesisvideo:us-east-1:149328592612:channel/822a28567ae513bcfef4c3ad98f2ae33f7a54c1c/1593368624057
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] Endpoints: {HTTPS: "https://r-b0e0aa33.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-d622a7db.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Endpoints: {HTTPS: "https://r-0606824a.kinesisvideo.us-east-1.amazonaws.com", WSS: "wss://v-4424355c.kinesisvideo.us-east-1.amazonaws.com"}
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] Starting viewer connection
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] ICE servers: (3) [{…}, {…}, {…}]
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Starting viewer connection
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] Connected to signaling service
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] Creating SDP offer
instrument.ts:129 [VIEWER gly7e3zk8m9yqjrmzvq1q9] Sending SDP offer
SignalingClient.ts:199 Uncaught (in promise) Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendSdpOffer (SignalingClient.ts:168)
at SignalingClient.<anonymous> (index.js:148)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendSdpOffer @ SignalingClient.ts:168
(anonymous) @ index.js:148
async function (async)
(anonymous) @ index.js:139
emit @ events.js:146
SignalingClient.onOpen @ SignalingClient.ts:231
sentryWrapped @ helpers.ts:85
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
SignalingClient.ts:199 Uncaught Error: Could not send message because the connection to the signaling service is not open.
at SignalingClient.sendMessage (SignalingClient.ts:199)
at SignalingClient.sendIceCandidate (SignalingClient.ts:190)
at RTCPeerConnection.<anonymous> (index.js:182)
SignalingClient.sendMessage @ SignalingClient.ts:199
SignalingClient.sendIceCandidate @ SignalingClient.ts:190
(anonymous) @ index.js:182
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Connected to signaling service
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Creating SDP offer
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [MASTER] Received SDP offer from client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Received ICE candidate from client: bij6jh35s7mcepqlcuehet
5instrument.ts:129 [MASTER] Received ICE candidate from client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Received SDP offer from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
2instrument.ts:129 [MASTER] Received ICE candidate from client: bij6jh35s7mcepqlcuehet
14instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Received ICE candidate from client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending SDP offer
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generating ICE candidates
6instrument.ts:129 [MASTER] Received ICE candidate from client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Received ICE candidate from client: bij6jh35s7mcepqlcuehet
17instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Generated ICE candidate
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Sending ICE candidate
instrument.ts:129 [MASTER] Creating SDP answer for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Creating SDP answer for client: apqu4hv9t9i7fljmcoj1e
16instrument.ts:129 [MASTER] Received ICE candidate from client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Received SDP answer
2instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Received ICE candidate
2instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] Received remote track
instrument.ts:129 [MASTER] Sending SDP answer to client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Generating ICE candidates for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Sending SDP answer to client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Generating ICE candidates for client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [VIEWER ktnjlm8myg530xr77qw42] All ICE candidates have been generated
instrument.ts:129 [MASTER] Generated ICE candidate for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Sending ICE candidate to client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Generated ICE candidate for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Sending ICE candidate to client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Generated ICE candidate for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Sending ICE candidate to client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Generated ICE candidate for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Sending ICE candidate to client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] Generated ICE candidate for client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Sending ICE candidate to client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Generated ICE candidate for client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Sending ICE candidate to client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Generated ICE candidate for client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Sending ICE candidate to client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Generated ICE candidate for client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] Sending ICE candidate to client: apqu4hv9t9i7fljmcoj1e
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: bij6jh35s7mcepqlcuehet
instrument.ts:129 [MASTER] All ICE candidates have been generated for client: apqu4hv9t9i7fljmcoj1e
I still have no idea what went wrong here. To make sure that we're on the same page, what SDK version are you using?
I've been using version 1.0.5
. I just upgraded to 1.0.8
and saw no difference.
Does the state of kstable
mean anything to you? I've been seeing it in errors since I've added the webrtc-adapter
.
From this state diagram https://www.w3.org/TR/webrtc/#rtcsignalingstate-enum, it sounds to me that your peer connection is in stable state, but somehow it calls setRemote(answer)
before setLocal(offer)
.
Hmmmmm...
Do you think there could be an issue with how the viewer
variable is used?
Do you see any issues with rendering multiple LiveFeedView
like this?
cosnt peers = [ 'arn:...', 'arn:...' ]
return (
<Row>
{ peers.length && peers.map( channelArn =>
<Col>
<LiveFeedView channelArn={channelArn} />
</Col>
}
</Row>
)
Given viewer
is defined like this
Ah! Yes, this will definitely cause a problem! Since all of your viewers will share the same peer connection, which is really bad. When I created that example, it was only based of a customer's snippet. I tried to not modify a lot of things so that it's clear to the customer.
But, yes, you can simply move viewer to the component so that each viewer will have a copy of it.
That was it! With that change, I got working results for a mesh conference of 5 separate devices! I'm still getting an issue for Safari for OSX (Safari for iOS works fine), getting and error on the following line...
peerConnection.addIceCandidate(candidate)
I will continue investigating on my own since my original request has been fulfilled. But other than that one scenario, every browser is having success.
Thank you @lherman-cs! You're the man! 💯
That's super awesome!! I'm glad that we found the problem. 😁
In that case, I'll close this issue.
@koterthecoder have you ever managed to finish up the implementation? I'm building the system where I need mesh structure (N:N) where 1 < N < 6 but I'm sceptic about the actual performance and complexity. Can you share your experiences? Thank you.
@pozhega yes, I was able to get this implementation working with several peers. Your performance concerns are valid for high numbers of peers (7+), I noticed video degradation, additional latency, and the machines were red-lining from a resource perspective.
BUT for your range of 4 or less, I found that the mesh architecture works quite well. Even mobile devices could handle that kind of capacity. Also if you configure audio only, the resource constraints are much less.
Thank you @koterthecoder and @lherman-cs , it worked like a charm! (i'm building a multiplayer card game with audio and video features, with 4 players connected, in a mesh topology)
That was it! With that change, I got working results for a mesh conference of 5 separate devices! I'm still getting an issue for Safari for OSX (Safari for iOS works fine), getting and error on the following line...
peerConnection.addIceCandidate(candidate)
I will continue investigating on my own since my original request has been fulfilled. But other than that one scenario, every browser is having success.
Thank you @lherman-cs! You're the man! 💯
Hey @koterthecoder , i am trying to do the same on react-native-webview for ios and can't get the video in sdp Offer/Answer. Does your code works fine on IOS (react-native-webview) uses safari ? as it is giving me a blank screen. It is working fine on chrome/firefox etc.
Description
I'm building a web application using ReactJs which provides a multi-user video conferencing experience. Currently for every peer that joins a "room," a dedicated signaling channel is created for their master connection. As users join the "room," a new signaling channel is created for the new user's master connection, in addition, the new user connects as a viewer to the existing signaling channels.
Sample Architecture: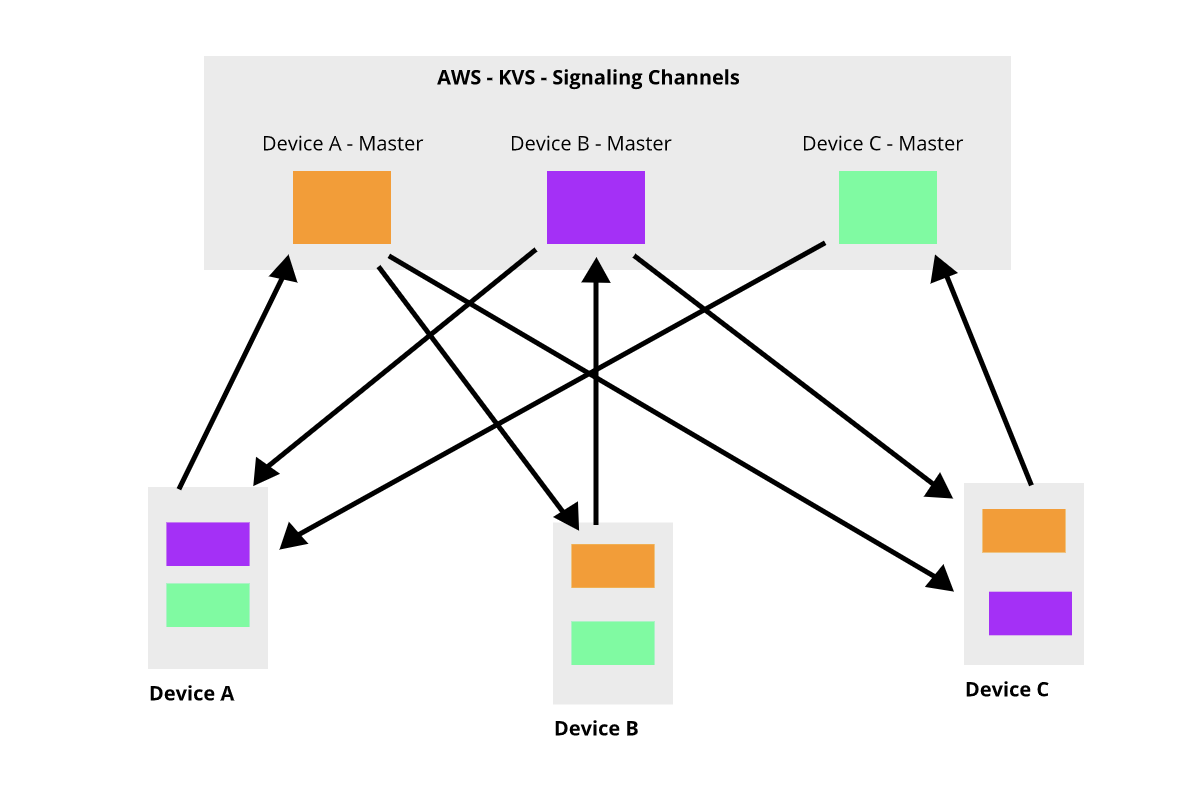
Problem
Currently my solution is working when the number of peers is two, but when the third user enters the room, the following error appears. It doesn't make sense to me that an existing signaling channel that already has a connected viewer is giving this error to a new viewer attempting to view the feed. For reference, I can view the feed just fine from the AWS console, just not in my app.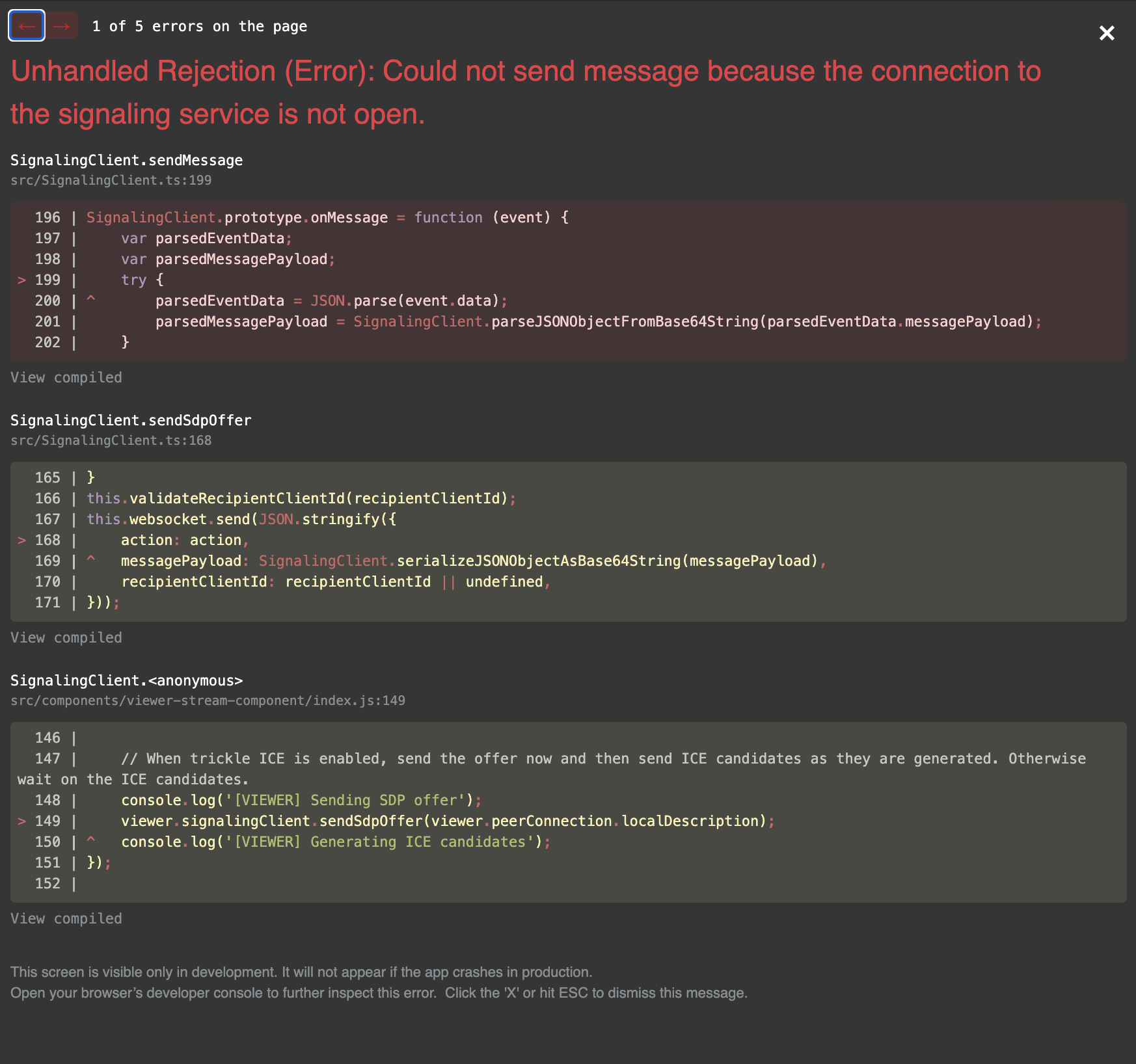
Thank you for reviewing my issue 🙌