Open matthieusieben opened 2 years ago
Hey @matthieusieben! We really appreciate you taking the time to report an issue. The collaborators on this project attempt to help as many people as possible, but we're a limited number of volunteers, so it's possible this won't be addressed swiftly.
If you need any help, or just have general Babel or JavaScript questions, we have a vibrant Slack community that typically always has someone willing to help. You can sign-up here for an invite.
Hi! I don't have easy access to IE, but could you check if mySet[Symbol.iterator]
exists?
Yes it does.
Here is the result of running the following code:
Set
is not the only one that does not work properly in IE
function* enumerate(it) {
yield* it
}
console.log('enumerate Array:')
for (const item of enumerate([1, 2])) {
console.log('item:', item);
}
console.log('enumerate enumerate:')
for (const item of enumerate(enumerate([1, 2]))) {
console.log('item:', item);
}
console.log('enumerate Set:')
for (const item of enumerate(new Set([1, 2]))) {
console.log('item:', item);
}
console.log('enumerate Map:')
for (const item of enumerate(new Map([[1,1], [2,2]]))) {
console.log('item:', item);
}
console.log('enumerate Map.entries():')
for (const item of enumerate(new Map([[1,1], [2,2]]).entries())) {
console.log('item:', item);
}
console.log('enumerate Map.values():')
for (const item of enumerate(new Map([[1,1], [2,2]]).values())) {
console.log('item:', item);
}
console.log('enumerate Map.keys():')
for (const item of enumerate(new Map([[1,1], [2,2]]).keys())) {
console.log('item:', item);
}
console.log('enumerate customIterable:')
const customIterable = {
[Symbol.iterator]() {
let i = 0;
return {
next() {
i++
if (i > 2) return { done: true }
return { done: false, value: i }
}
}
}
}
for (const item of enumerate(customIterable)) {
console.log('item:', item);
}
console.log('enumerate customIterableInstance:')
function CustomIterable() {}
CustomIterable.prototype = customIterable
for (const item of enumerate(new CustomIterable())) {
console.log('item:', item);
}
I think I found the problem. In Babel's result, the regenerator-runtime
is imported before the core-js/modules/es.symbol
polyfills.
However, the regenerator-runtime
reads the value of Symbol.iterator
when the module is loaded (and not when regenerator functions are invoked) causing the fallback @@iterator
to be used by regenerator-runtime
methods, which is not correct.
Manually changing the order of the Babel result fixes the issue.
💻
How are you using Babel?
Other (Next.js, Gatsby, vue-cli, ...)
Input code
Here is a link to a code sandbox that shows the error when deploying on netlify and running in IE11: https://codesandbox.io/s/ie11-test-forked-7yfz7b?file=/src/index.js
Try yourself: https://csb-7yfz7b.netlify.app/
Configuration file name
No response
Configuration
Current and expected behavior
The console should show the following (as it does in chrome):
The actual console content in IE11 shows an empty array: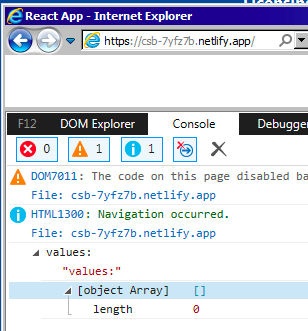
Environment
Possible solution
No response
Additional context
I can workaround this issue by doing:
or avoid using
yield*
: