Closed raffaelmiranda closed 1 year ago
Hi @raffaelmiranda ,
First,
Software | Version(s) |
---|---|
Bogus NuGet Package | 34.0.3 |
At the time of this writing, Version 34.0.3 does not exist:
Second, your screenshot shows you are mostly using Random.String()
not Random.String2()
.
To understand the difference, you'll need to read the implementation and XML documentation for String
and String2
in how they differ shown below:
.String()
.String2()
Notice, that both implementations do not work with any locale. They are mostly a-z
and char.MinValue - char.MaxValue
methods.
Hope that helps.
Version Information
What locale are you using with Bogus?
Brazil
What's the problem?
When I use f.Random.String2(25), even if I set pt_BR in locale, it returns data that is impossible to read for another encoding or for another language. Maybe there was a missing setting in Bogus?
What possible solutions have you considered?
I considered only what is shown in the code example
Do you have sample code to show what you're trying to do?
Result: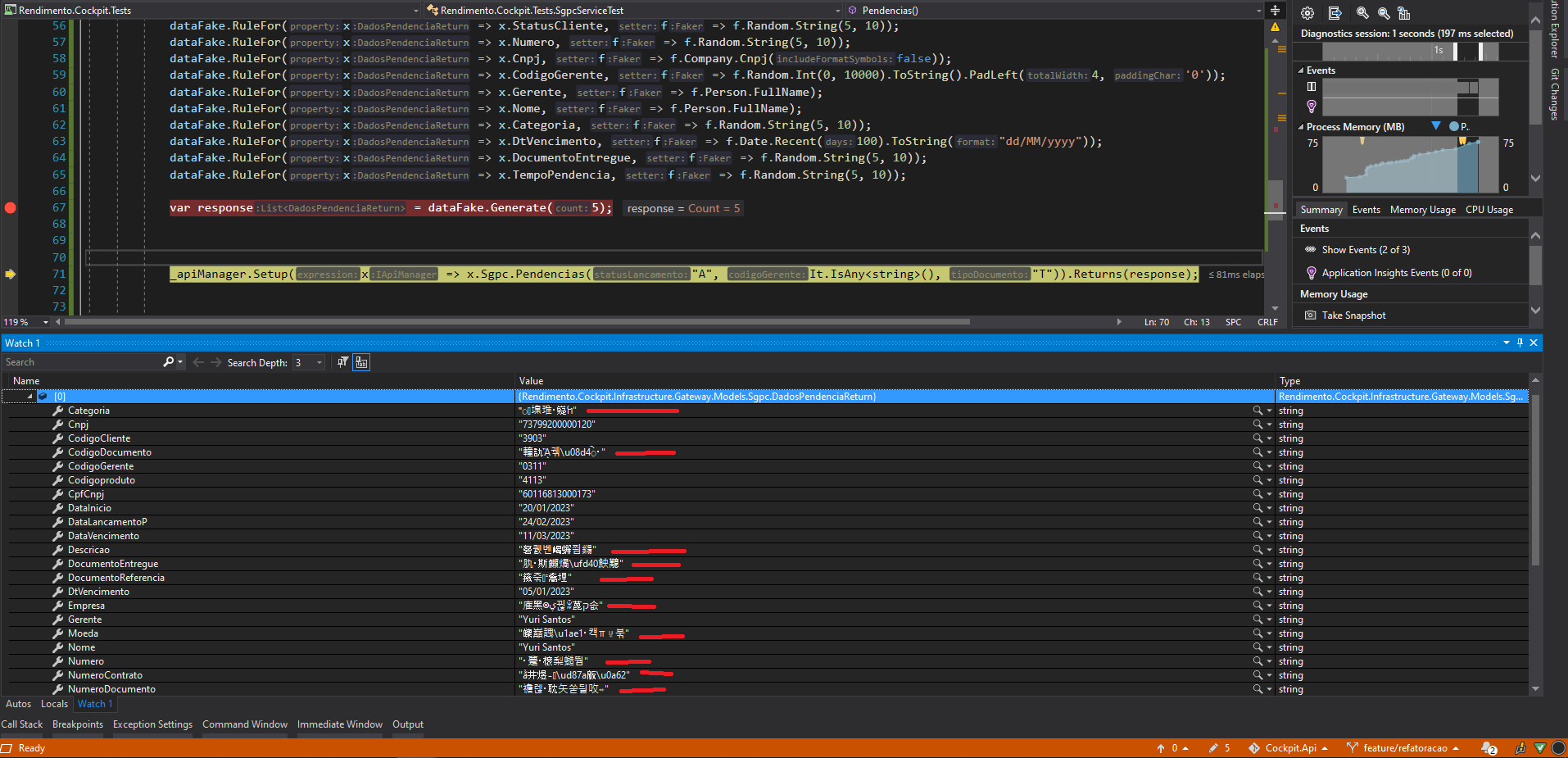
(Please be complete. Provide all code necessary to run your example in LINQPad.) (The more complete code examples are, the more accurate answers will be.) (https://www.linqpad.net)