Closed athenasaurav closed 3 years ago
I can't see clear information from the screenshot that showing issue of query order.
Please try to send request to GET /api/v3/order
with:
please let us know if you have any concern.
Hello @2pd Thanks for your response.
Sorry it was my fault trade was working I was just checking the wrong Binance account.
Hello Everyone
I m trying to use you this python binance to buy and sell crypto currency.
Currently i have more than enough balance to place BTCUSDT trade of buy or sell in my account. I have written a simple script to buy crypto first and then after some delay time sell them using your following code :
Code used :
In the terminal i see no errors, buy and sell are successful i even get order id, trade id etc but when i check the history in SPOT order of trade history it doesn't appear in there.
Terminal Window screenshot attached. But in the SPOT wallet order history or trade history it doesn't shows up. Only those trades are shown that I did manually.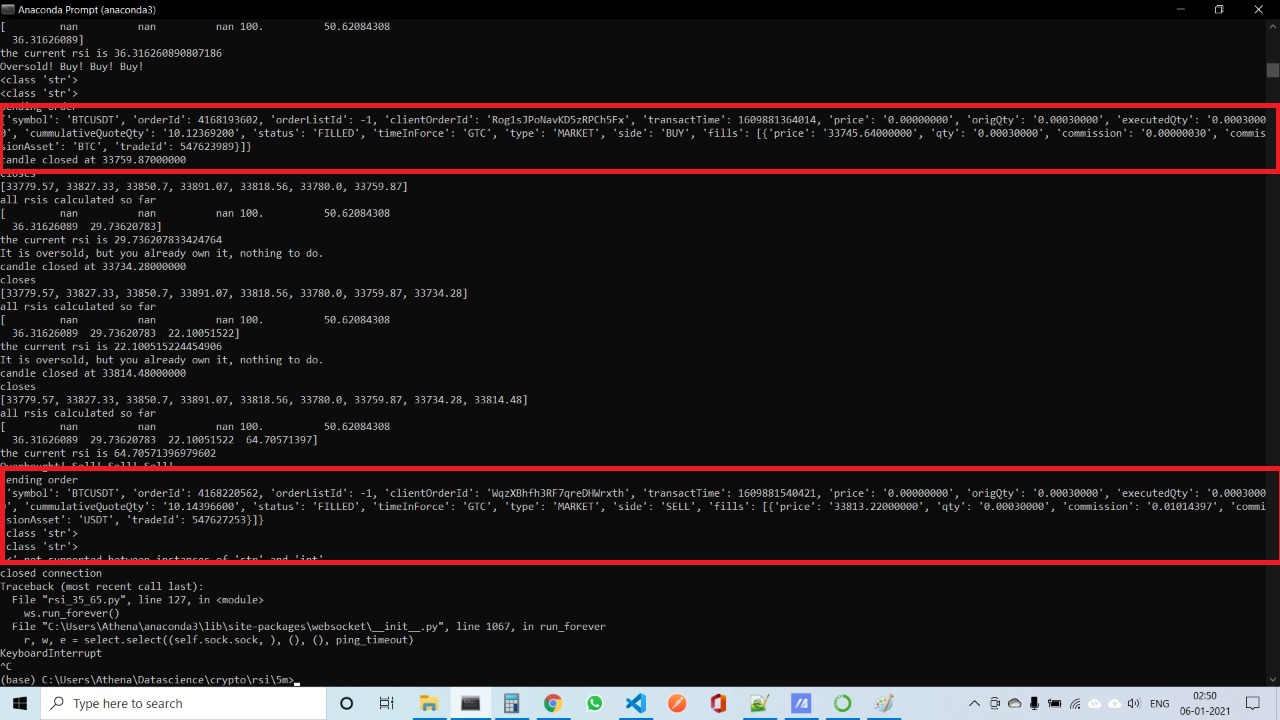