I'm currently working on flutter app. One of main requests is PayPal/Braintree payments. I will make android and iOS separately. First I implemented android part.
private void showDropInUi(String clientToken) {
final DropInRequest dropInRequest = new DropInRequest()
.clientToken(clientToken)
.vaultManager(true);
startActivityForResult(dropInRequest.getIntent(this), REQUEST_CODE);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
final Map<String, String> map = new LinkedHashMap<>();
if (requestCode == REQUEST_CODE) {
if (resultCode == RESULT_OK) {
final DropInResult dropInResult = data.getParcelableExtra(DropInResult.EXTRA_DROP_IN_RESULT);
String paymentNonce = null;
if (dropInResult != null && dropInResult.getPaymentMethodNonce() != null) {
paymentNonce = dropInResult.getPaymentMethodNonce().getNonce();
}
if (paymentNonce == null || paymentNonce.isEmpty()) {
map.put("status", "failure");
map.put("message", "Payment nonce is null or empty.");
mResult.success(map);
} else {
map.put("status", "success");
map.put("message", "Payment nonce is ready.");
map.put("payment_nonce", paymentNonce);
mResult.success(map);
}
} else if (resultCode == RESULT_CANCELED) {
map.put("status", "failure");
map.put("message", "User canceled payment.");
mResult.success(map);
} else {
final Exception error = (Exception) data.getSerializableExtra(DropInActivity.EXTRA_ERROR);
map.put("status", "failure");
map.put("message", error != null ? error.toString() : "Error is null.");
mResult.success(map);
}
}
}
I make request to server to get 'client token'. Then I start Drop-in UI. And finally, when I get nonce, I make another request to make transaction. Braintree sdk is working and I already made dozens of payments using test credit cards and test PayPal account.
So, the question is: how to show recent payment methods in Drop-in UI? This must be handled by back end by managing customers and customerId? Or I need some extra java code for this? Because I can't find anything in documentation for this.
Hi @VladimirMitso. You will need to pass a customer ID when creating your client token in order to show vaulted (saved) payment methods in Drop-In. Please take a look at this documentation.
I'm currently working on flutter app. One of main requests is PayPal/Braintree payments. I will make android and iOS separately. First I implemented android part.
AndroidManifest.xml
build.gradle
Activity class
I make request to server to get 'client token'. Then I start Drop-in UI. And finally, when I get nonce, I make another request to make transaction. Braintree sdk is working and I already made dozens of payments using test credit cards and test PayPal account.
So, the question is: how to show recent payment methods in Drop-in UI? This must be handled by back end by managing customers and
customerId
? Or I need some extra java code for this? Because I can't find anything in documentation for this.I want this: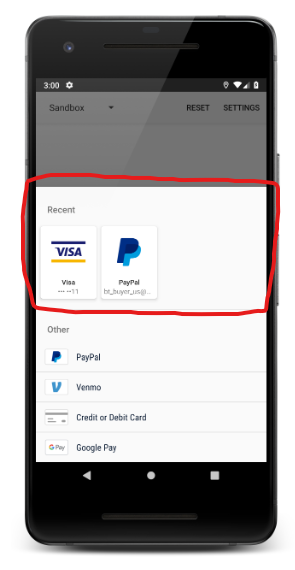
And only I can get is this: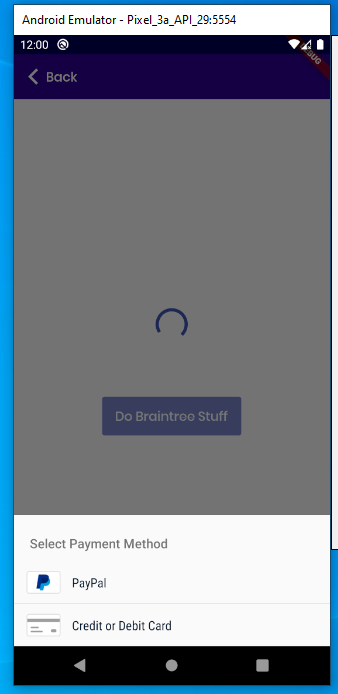