Closed yngtodd closed 6 years ago
I tested it on Ubuntu 16 and there it works. Can you maybe show the hello.c / .h and stdio.h? The compiler output should be in output/hello/build/build.log..
Here is the hello.c
:
// WARNING: this file is auto-generated by the C2 compiler.
// Any changes you make might be lost!
#include "stdio.h"
#include "hello.h"
int32_t main(int32_t argc, unsigned char* argv[]) {
printf("Hello C2!\n");
return 0;
}
hello.h
:
// WARNING: this file is auto-generated by the C2 compiler.
// Any changes you make might be lost!
#ifndef HELLO_H
#define HELLO_H
#include "c2types.h"
#ifdef __cplusplus
extern "C" {
#endif
#ifdef __cplusplus
}
#endif
#endif
And stdio.h
:
// WARNING: this file is auto-generated by the C2 compiler.
// Any changes you make might be lost!
#ifndef STDIO_H
#define STDIO_H
#include "c2types.h"
#include "c2.h"
#ifdef __cplusplus
extern "C" {
#endif
#define _IOFBF 0
#define _IOLBF 1
#define _IONBF 2
#define EOF -1
#define SEEK_SET 0
#define SEEK_CUR 1
#define SEEK_END 2
typedef struct _IO_marker_ _IO_marker;
typedef struct FILE_ FILE;
struct _IO_marker_ {
_IO_marker* next;
FILE* sbuf;
int32_t _pos;
};
typedef uint64_t off_t;
int32_t remove(const char* __filename);
int32_t rename(const char* __old, const char* __new);
FILE* tmpfile();
char* tempnam(const char* __dir, const char* __pfx);
int32_t fclose(FILE* __stream);
int32_t fflush(FILE* __stream);
FILE* fopen(const char* __filename, const char* __modes);
FILE* freopen(const char* __filename, const char* __modes, FILE* __stream);
FILE* fdopen(int32_t __fd, const char* __modes);
void setbuf(FILE* __stream, char* __buf);
int32_t setvbuf(FILE* __stream, char* __buf, int32_t __modes, uint64_t __n);
void setbuffer(FILE* __stream, char* __buf, uint64_t __size);
void setlinebuf(FILE* __stream);
int32_t fprintf(FILE* __stream, const char* __format, ...);
int32_t printf(const char* __format, ...);
int32_t sprintf(char* __s, const char* __format, ...);
int32_t dprintf(int32_t __fd, const char* __fmt, ...);
int32_t fscanf(FILE* __stream, const char* __format, ...);
int32_t scanf(const char* __format, ...);
int32_t sscanf(const char* __s, const char* __format, ...);
int32_t fgetc(FILE* __stream);
int32_t getc(FILE* __stream);
int32_t getchar();
int32_t getc_unlocked(FILE* __stream);
int32_t getchar_unlocked();
int32_t fputc(int32_t __c, FILE* __stream);
int32_t putc(int32_t __c, FILE* __stream);
int32_t putchar(int32_t __c);
int32_t putchar_unlocked(int32_t __c);
int32_t getw(FILE* __stream);
int32_t putw(int32_t __w, FILE* __stream);
char* fgets(char* __s, int32_t __n, FILE* __stream);
int64_t getdelim(char** __lineptr, uint64_t* __n, int32_t __delimiter, FILE* __stream);
int64_t getline(char** __lineptr, uint64_t* __n, FILE* __stream);
int32_t fputs(const char* __s, FILE* __stream);
int32_t puts(const char* __s);
int32_t ungetc(int32_t __c, FILE* __stream);
int32_t fread(void* __ptr, uint64_t __size, uint64_t __n, FILE* __stream);
uint64_t fwrite(const void* __ptr, uint64_t __size, uint64_t __n, FILE* __s);
int32_t fseek(FILE* __stream, int64_t __off, int32_t __whence);
int64_t ftell(FILE* __stream);
void rewind(FILE* __stream);
int32_t fseeko(FILE* __stream, uint64_t __off, int32_t __whence);
uint64_t ftello(FILE* __stream);
void clearerr(FILE* __stream);
int32_t feof(FILE* __stream);
int32_t ferror(FILE* __stream);
void perror(const char* __s);
int32_t fileno(FILE* __stream);
FILE* popen(const char* __command, const char* __modes);
int32_t pclose(FILE* __stream);
char* ctermid(char* __s);
void flockfile(FILE* __stream);
int32_t ftrylockfile(FILE* __stream);
void funlockfile(FILE* __stream);
char* tmpnam_r(char* __s);
int32_t renameat(int32_t __oldfd, const char* __old, int32_t __newfd, const char* __new);
FILE* fmemopen(void* __s, uint64_t __len, const char* __modes);
FILE* open_memstream(char** __bufloc, uint64_t* __sizeloc);
int32_t fflush_unlocked(FILE* __stream);
int32_t fgetc_unlocked(FILE* __stream);
int32_t putc_unlocked(int32_t __c, FILE* __stream);
int32_t fputc_unlocked(int32_t __c, FILE* __stream);
int64_t __getdelim(char** __lineptr, uint64_t* __n, int32_t __delimiter, FILE* __stream);
int32_t fread_unlocked(void* __ptr, uint64_t __size, uint64_t __n, FILE* __stream);
uint64_t fwrite_unlocked(const void* __ptr, uint64_t __size, uint64_t __n, FILE* __stream);
void clearerr_unlocked(FILE* __stream);
int32_t feof_unlocked(FILE* __stream);
int32_t ferror_unlocked(FILE* __stream);
int32_t asprintf(char** _arg0, const char* _arg1, ...);
char* ctermid_r(char* _arg0);
char* fgetln(FILE* _arg0, uint64_t* _arg1);
const char* fmtcheck(const char* _arg0, const char* _arg1);
int32_t fpurge(FILE* _arg0);
FILE* zopen(const char* _arg0, const char* _arg1, int32_t _arg2);
#define stdin __stdinp
extern FILE* __stdinp;
#define stdout __stdoutp
extern FILE* __stdoutp;
#define stderr __stderrp
extern FILE* __stderrp;
#ifdef __cplusplus
}
#endif
#endif
what does the build.log say? (it should contain the actual compiler error message)
On Sat, Feb 3, 2018 at 9:47 PM, Todd Young notifications@github.com wrote:
Here is the hello.c:
// WARNING: this file is auto-generated by the C2 compiler. // Any changes you make might be lost!
include "stdio.h"
include "hello.h"
int32_t main(int32_t argc, unsigned char* argv[]) { printf("Hello C2!\n"); return 0; }
hello.h:
// WARNING: this file is auto-generated by the C2 compiler. // Any changes you make might be lost!
ifndef HELLO_H
define HELLO_H
include "c2types.h"
ifdef __cplusplus
extern "C" {
endif
ifdef __cplusplus
}
endif
endif
And stdio.h:
// WARNING: this file is auto-generated by the C2 compiler. // Any changes you make might be lost!
ifndef STDIO_H
define STDIO_H
include "c2types.h"
include "c2.h"
ifdef __cplusplus
extern "C" {
endif
define _IOFBF 0
define _IOLBF 1
define _IONBF 2
define EOF -1
define SEEK_SET 0
define SEEK_CUR 1
define SEEK_END 2
typedef struct _IOmarker _IO_marker;
typedef struct FILE_ FILE;
struct _IOmarker { _IO_marker next; FILE sbuf; int32_t _pos; };
typedef uint64_t off_t;
int32_t remove(const char __filename); int32_t rename(const char old, const char* new); FILE tmpfile(); char tempnam(const char __dir, const char pfx); int32_t fclose(FILE __stream); int32_t fflush(FILE stream); FILE fopen(const char filename, const char* modes); FILE freopen(const char filename, const char* modes, FILE __stream); FILE fdopen(int32_t fd, const char* modes); void setbuf(FILE __stream, char buf); int32_t setvbuf(FILE __stream, char __buf, int32_t modes, uint64_t n); void setbuffer(FILE* stream, char __buf, uint64_t __size); void setlinebuf(FILE stream); int32_t fprintf(FILE __stream, const char __format, ...); int32_t printf(const char* format, ...); int32_t sprintf(char __s, const char format, ...); int32_t dprintf(int32_t fd, const char __fmt, ...); int32_t fscanf(FILE stream, const char* format, ...); int32_t scanf(const char __format, ...); int32_t sscanf(const char s, const char* format, ...); int32_t fgetc(FILE __stream); int32_t getc(FILE stream); int32_t getchar(); int32_t getc_unlocked(FILE stream); int32_t getchar_unlocked(); int32_t fputc(int32_t c, FILE stream); int32_t putc(int32_t c, FILE* stream); int32_t putchar(int32_t c); int32_t putchar_unlocked(int32_t c); int32_t getw(FILE stream); int32_t putw(int32_t w, FILE stream); char fgets(char s, int32_t n, FILE* stream); int64_t getdelim(char lineptr, uint64_t* __n, int32_t delimiter, FILE* __stream); int64_t getline(char lineptr, uint64_t __n, FILE __stream); int32_t fputs(const char* s, FILE __stream); int32_t puts(const char s); int32_t ungetc(int32_t c, FILE __stream); int32_t fread(void ptr, uint64_t __size, uint64_t n, FILE __stream); uint64_t fwrite(const void ptr, uint64_t __size, uint64_t n, FILE __s); int32_t fseek(FILE stream, int64_t __off, int32_t whence); int64_t ftell(FILE __stream); void rewind(FILE stream); int32_t fseeko(FILE* __stream, uint64_t off, int32_t whence); uint64_t ftello(FILE __stream); void clearerr(FILE __stream); int32_t feof(FILE* stream); int32_t ferror(FILE __stream); void perror(const char s); int32_t fileno(FILE __stream); FILE popen(const char __command, const char __modes); int32_t pclose(FILE* stream); char ctermid(char s); void flockfile(FILE* stream); int32_t ftrylockfile(FILE __stream); void funlockfile(FILE stream); char tmpnam_r(char s); int32_t renameat(int32_t oldfd, const char* __old, int32_t newfd, const char __new); FILE fmemopen(void __s, uint64_t __len, const char modes); FILE* open_memstream(char* __bufloc, uint64_t sizeloc); int32_t fflush_unlocked(FILE __stream); int32_t fgetc_unlocked(FILE stream); int32_t putc_unlocked(int32_t c, FILE* stream); int32_t fputc_unlocked(int32_t c, FILE* stream); int64_t getdelim(char** lineptr, uint64_t* n, int32_t delimiter, FILE* stream); int32_t fread_unlocked(void ptr, uint64_t __size, uint64_t n, FILE stream); uint64_t fwrite_unlocked(const void* ptr, uint64_t size, uint64_t n, FILE* stream); void clearerr_unlocked(FILE* stream); int32_t feof_unlocked(FILE __stream); int32_t ferror_unlocked(FILE __stream); int32_t asprintf(char* _arg0, const char _arg1, ...); char ctermid_r(char _arg0); char fgetln(FILE _arg0, uint64_t _arg1); const char fmtcheck(const char _arg0, const char _arg1); int32_t fpurge(FILE _arg0); FILE zopen(const char _arg0, const char _arg1, int32_t _arg2);
define stdin __stdinp
extern FILE* __stdinp;
define stdout __stdoutp
extern FILE* __stdoutp;
define stderr __stderrp
extern FILE* __stderrp;
ifdef __cplusplus
}
endif
endif
— You are receiving this because you commented. Reply to this email directly, view it on GitHub https://github.com/c2lang/c2compiler/issues/30#issuecomment-362853067, or mute the thread https://github.com/notifications/unsubscribe-auth/AAC1munrubbVNcpbgMP-SH1Bc75FW3nxks5tRMXogaJpZM4R4Sfc .
Ah, sorry I forgot to post that. Here it is:
current dir: /Users/youngtodd/c2/hello
changing to dir: output/hello/build/
running command: /usr/bin/make
gcc -O3 -Wall -Wextra -Wno-unused -Wno-unused-parameter -std=c99 -c hello.c -o hello.o
hello.c:9:9: error: second parameter of 'main' (argument array) must be of type 'char **'
int32_t main(int32_t argc, unsigned char* argv[]) {
^
1 error generated.
make: *** [../hello] Error 1
Currently in C2 a char is mapped to an 'unsigned char' in C. Please see the Forum discussion. Since this is causing a lot of compile warnings, I'm thinking about changing it back to char. This would fix your issues. Other examples in the codebase do work, i see they use:
main(i32 argc, const i8*[] argv). That should also work for you. Maybe you can try that before I change the compiler.
Using const i8*[] argv
works!
That then gives the a const char
in hello.c
:
// WARNING: this file is auto-generated by the C2 compiler.
// Any changes you make might be lost!
#include "stdio.h"
#include "hello.h"
int32_t main(int32_t argc, const char* argv[]) {
printf("Hello C2!\n");
return 0;
}
Thank you for your help!
When trying out the hello world example, I am getting an
external c compilation error
. After building the c2compiler, all the tests seem to be fine:Here is the .c2 file:
And the recipe:
Here is the tree of the resulting output: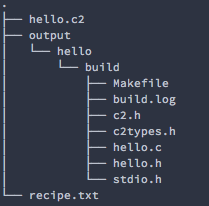
Here is the results of
gcc --version
:Any thoughts on where I should look into this one?