Open LukasBergs opened 3 years ago
What you want to achieve is merging 2 pose graphs. In cartographer's map builder this would be possible by using LoadState
two times for each of your files. But there's no direct way to add an offset to one of these loaded states (at least none that I know from top of my head atm).
Given that loading a pose graph state requires a bit more than just loading the proto data (see https://github.com/cartographer-project/cartographer/blob/105c034577220268cd28a304a185adbec46b729f/cartographer/mapping/map_builder.cc#L216), I'm not sure if this would be a trivial thing to do in a Python script. Theoretically, it should be possible though.
Hi.
I use the Cartographer for pure localization mode. For my use case it is necessary to update certain areas of the map regularly. However, I want to avoid having to re-record the entire map each time. Therefore I would like to compose an overall map from several individual maps. Since not all areas of the map are relevant for localization, it is not a problem if the individual maps do not overlap. The following pictures should clarify the situation.
Overall Map: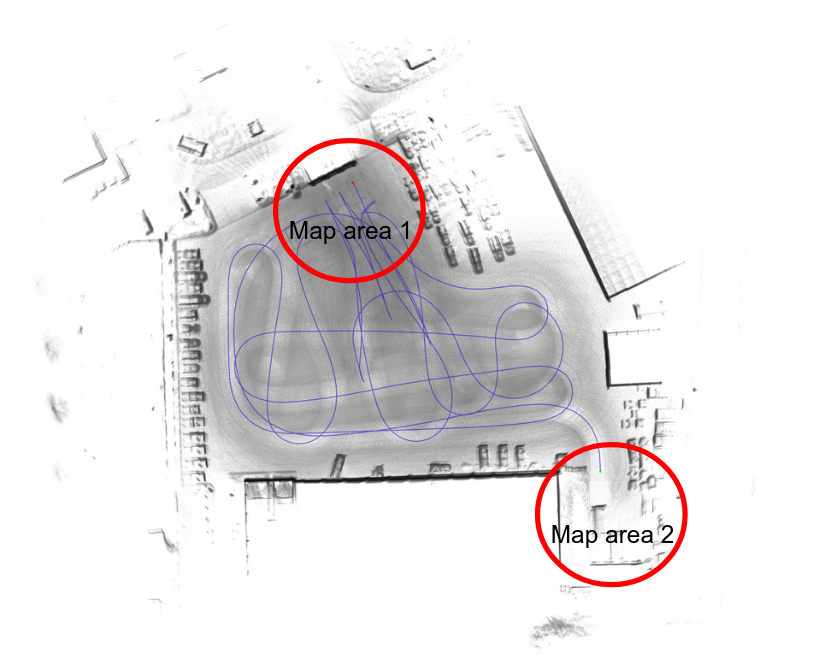
Individual map 1: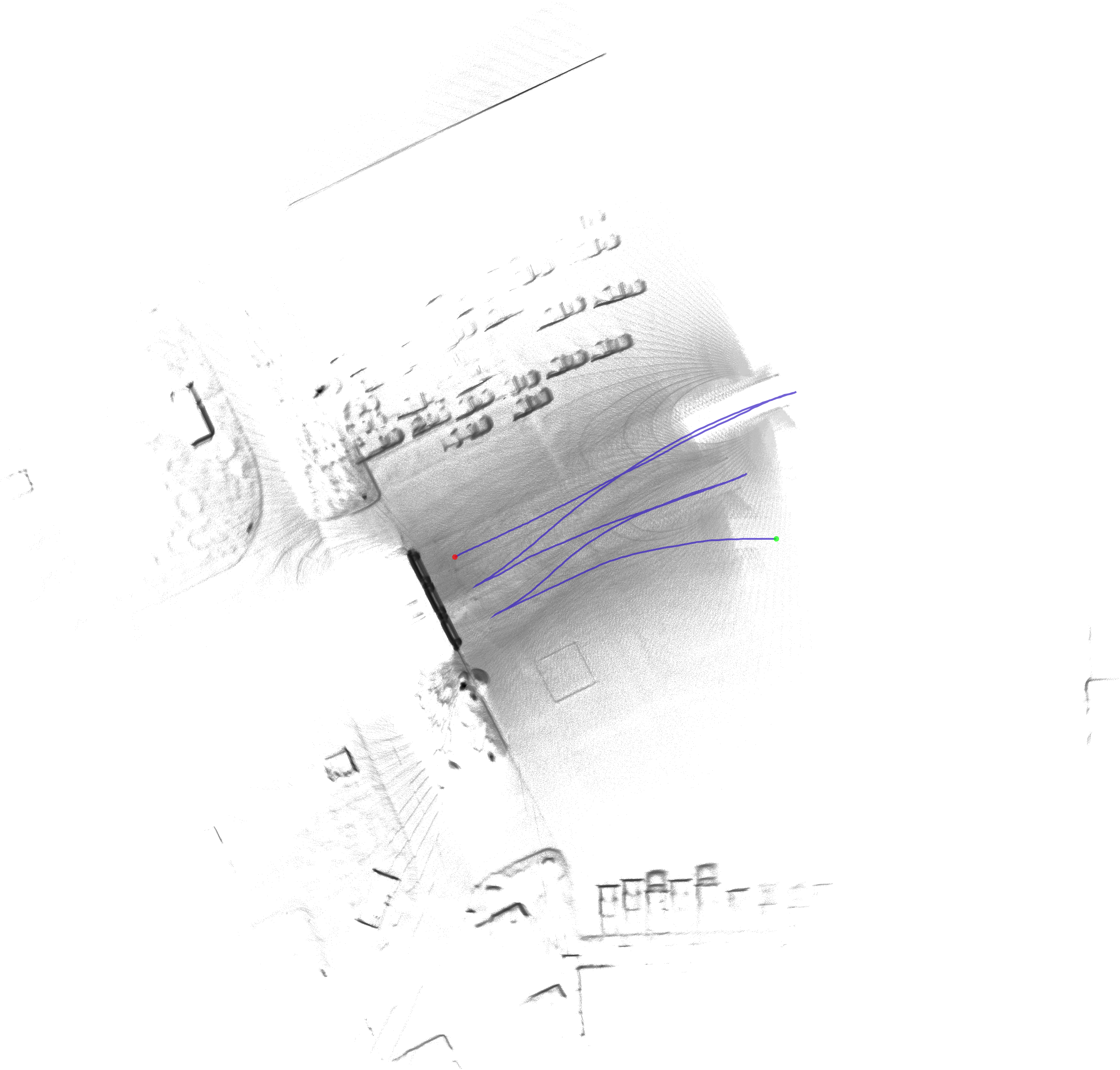
Individual map 2: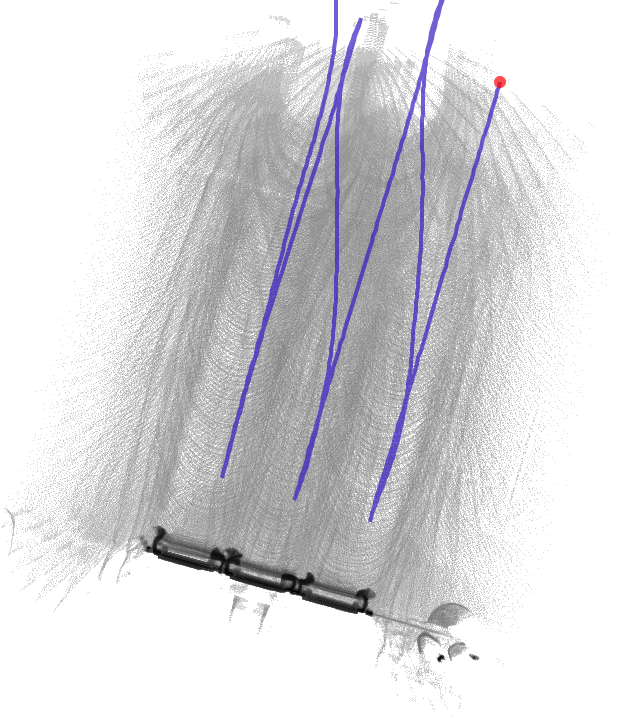
Merged Map: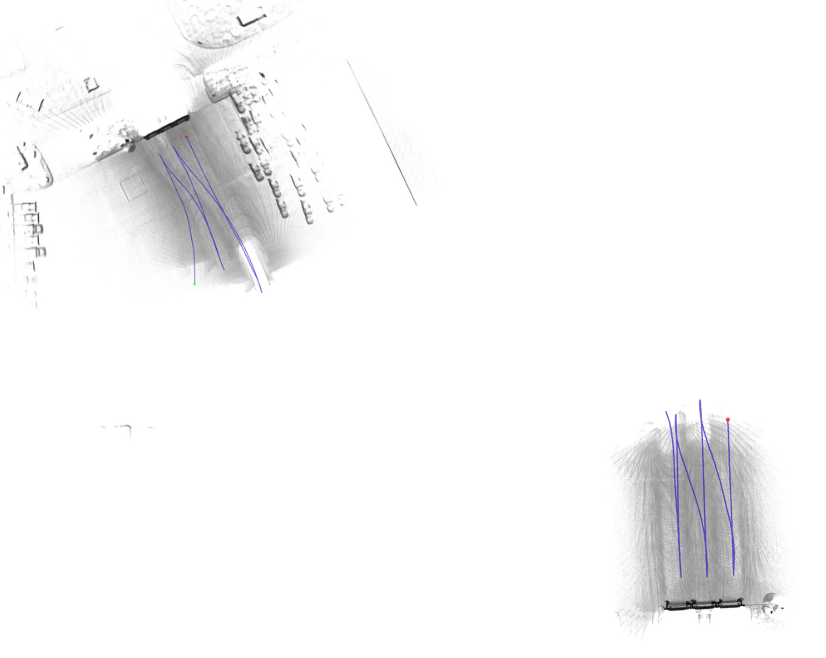
My questions:
1) Is it possible to merge multiple pbstreams into one map/pbstream that i can use for pure localization mode? I already figured out how to read pbstreams in python and get the stored information. For this I use the code below. However, I don't know how to merge multiple pbstreams and save them as a new pbstream. Can anyone help me with this?
2) Before I can merge the pbstreams, I have to arrange them correctly. Otherwise, they would simply overlap, since the origin of each frame is at the beginning of the trajectory. To do this, I need to apply a translation and a rotation to the pbstream content. Is it possible to simply "move" the submap origin or do I need to consider other things?
I hope someone can help me, as I am currently stuck. I would be very grateful for any help!
Many thanks in advance, Lukas