Closed horseinthesky closed 1 year ago
@bashbunni Hi. I've watched a couple of your vids and CLI Kanban Board playlist on youtube. I just don't see any examples using two lists in one model so can't figure out what is my mistake here. Could you pls help we with this?
Ah that's because you need to set the height and width on both lists to see the items. It looks like it's missing for listTwo
. That should fix it!
@bashbunni Thank you.
It somehow wants me to set height/width only on second list. It is perfectly fine with 0, 0
on the first one. Anyway it works =)
Hello. I try to create a model with two lists and the ability to toggle between them
I've changed
list-default
example but got into an issue: the second list shows the number of its items but not showing the items themselves.The first list is fine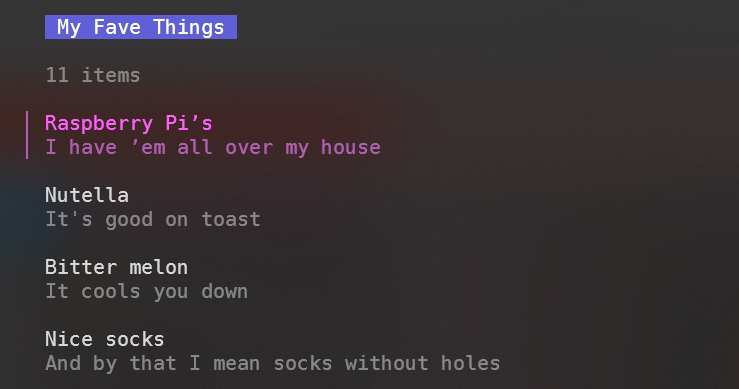
The Second has amount of items and a counter below but items are hidden somehow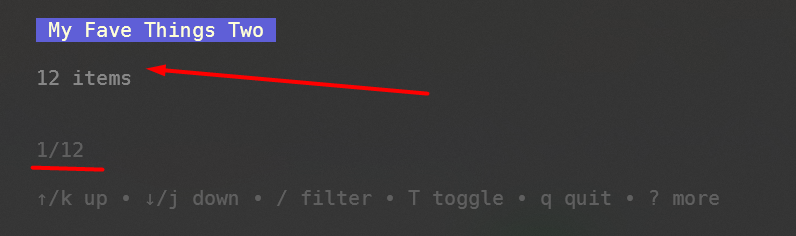
Could you please help me to fix it?
Here is the code: