Closed CodingOctocat closed 1 year ago
@CodingOctocat I revised the package, please upgrade to version 2, plus don't AWAIT _debounceDispatcher.DebounceAsync, run it asynchronously
How do I understand this statement? Is not using await contradictory to running asynchronously?
plus don't AWAIT _debounceDispatcher.DebounceAsync, run it asynchronously
Could you give the code?
@CodingOctocat DebounceAsync means the invocation it's a task and this method returns this task, so if you await then it will wait for the execution, so just do not await. here is the working sample code (don't forget to upgrade the NuGet):
using DebounceThrottle;
string str = "";
var _debounceDispatcher = new DebounceDispatcher(1000);
while (true)
{
var key = Console.ReadKey(true);
//trigger when to stop and exit
if (key.Key == ConsoleKey.Escape) break;
str += key.KeyChar;
Console.Write(key.KeyChar);
Dump("log.txt", str);
}
return;
void Dump(string path, string text, CancellationToken cancellationToken = default)
{
var task = _debounceDispatcher.DebounceAsync(async () => {
await File.WriteAllTextAsync(path, text, cancellationToken);
Console.WriteLine("---- dump ----");
});
}
I always thought the Async
method was always used in conjunction with await
, but in that case, why not design it to use the Debounce()
method?
@CodingOctocat you are right, but here you get the task in case you want run ContinueWith on executing task or explicitly await when it’s invoked
I want to save text to file, I need to save in real time, when the typing is finished 1s after the save.
DebounceAsync shows throttle behavior: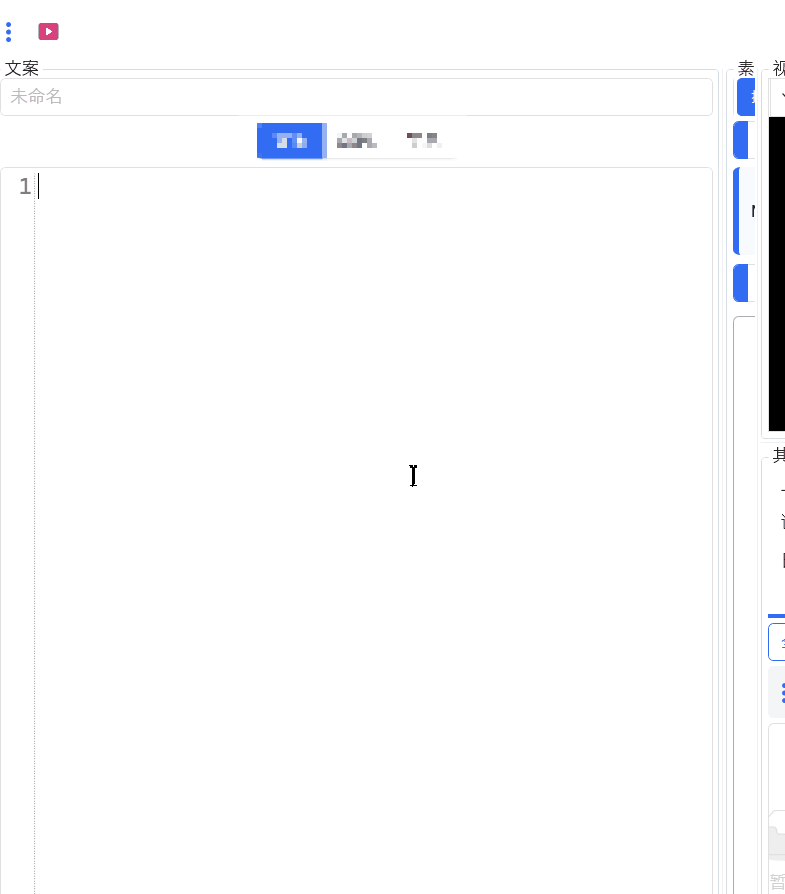