Closed AntmanTmp closed 4 years ago
Don't alias the good or supplier name in query2. The aliases are confusing you. Query2 returns rows but the repr is empty (none) because of the way you aliases the good name.
I strongly suggest you check out the docs on relationships and joins:
http://docs.peewee-orm.com/en/latest/peewee/relationships.html
@coleifer Thanks all for you help, i made a mistake and not use the relationship
query3 = (Goods.select(
Goods.name.alias('g_name'), Supplier.name.alias('s_name')
).join(Supplier).where(Goods.name.contains('am')).order_by(Goods.name).limit(10))
for item in query3:
print(f'{item.g_name:>15}:{item.supplier.s_name}')
Amaranth Leaves:Joshua
Amaranth Leaves:Georgeann
Amaranth Leaves:Londa
Amaranth Leaves:Lee
Bamboo Shoots:Hubert
Bamboo Shoots:Seymour
Bamboo Shoots:Delmar
Bamboo Shoots:Buster
Bamboo Shoots:Mirella
Edamame:Delmy
I have two model and random insert some rows
When I try to use join method, I found some doubts, so maybe there is wrong about my code or some flaws of this lib
when i call query_data func, get this result
But I print sql of query2, and it is ok when run script in mysql
result below: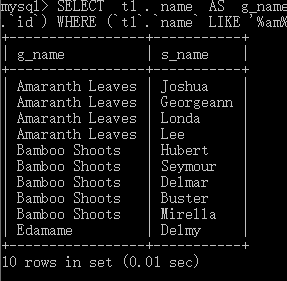
Q: why all the item in query2 is None?