Closed Janrupf closed 7 years ago
This is likely a bug in your own code. In future, you would be better off posting on the forums.
As for the cause of the issue, your function calls itself:
if msg == nil then
print("Invalid message recived! (No JSON)")
placeholder()
handle()
end
Every time you call a function, a new "instance" of that function goes on the end of the "function stack" (really a Java array with LuaJ, as is used by ComputerCraft). Every time a function completes, it's removed from the stack and execution continues from the point where the previous function instance left off. - [source](http://www.computercraft.info/forums2/index.php?/topic/28876-javalangarrayindexoutofboundsexception/page__view__findpost__p__268721]
handle sometimes calls itself and sometimes (always?) never returns, each time this means that the function stack (stored in a java array) gets bigger. Eventually this overflows causing the error message you received,
This generally means you're getting a stack overflow - probably as sendTrain
calls itself. I think this is as try
is never reset on a failure, so is 21 when called the second time.
Maybe I should sleep a while. I trying it I often let functions call itself, but i9 never tried it without sleeping
adding a sleep block fixed the issuise, maybe cc should output the stacktrace to the console (or is there a debug mode?)
Although the crash is indeed due to problems in the script, how tricky would it be to have ComputerCraft produce a more descriptive error? "Too many functions in stack" or somesuch?
This is undoubtably a bug in your program, not cc
@dan200 the topic of the issue changed to having CC give a clearer error for stack overflow. Thoughts?
So, hello, the code above will result in an ArrayIndexOutOfBoundsExecption after the second fail... Im not getting any stacktrace in console (running on a server).
Here is an screenshot: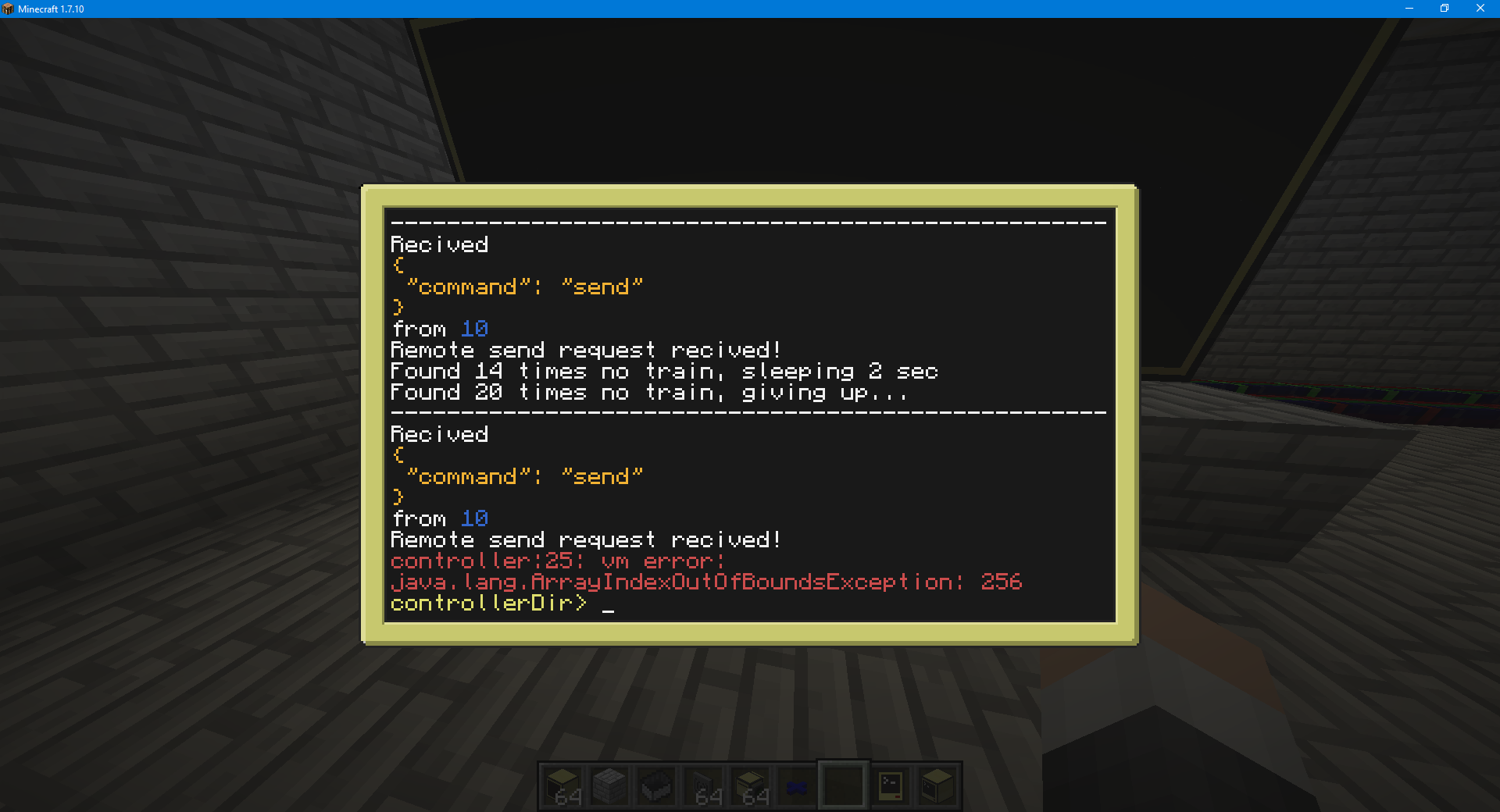
If Im doing something wrong then tell me ;)