Open vaibhav231093 opened 2 years ago
Hey there Vaibhav, thank you for your submission. Nice job getting the app correctly configured and deployed. The only thing we are missing is step 2 of the homework.
Please post an image of the "completed" screen once finished.
Name: Vaibhav E Email: e.vaibhav93@gmail.com Linkedin Profile: https://www.linkedin.com/in/vaibhav231093
Step 1 : Set up the server
Step 1a : unzip demo.zip and open DemoApplication.java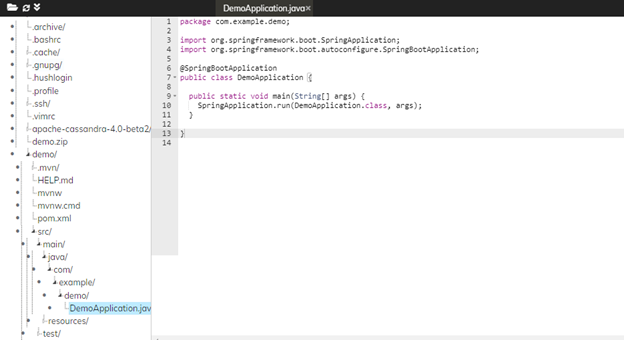
Step 1b : Run the project in the terminal with the following command.
Step 1c : Run the below command in the second terminal
curl 127.0.0.1:8080/ | jq
Expected Output :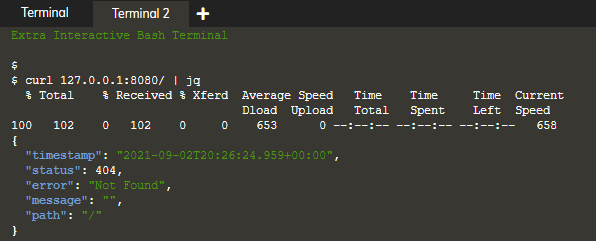
Step 2 : Add a simple response
Step 2a : Modify DemoApplication.java by adding request mapping method, changing class annotations and fix up the imports like below.
Open demo/src/main/java/com/example/demo/DemoApplication.java
`package com.example.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.; import org.springframework.web.bind.annotation.;
@RestController @EnableAutoConfiguration public class DemoApplication {
public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); }
@RequestMapping("/") String home() { return "Home\n"; }
// TODO: add Hello handler here }`
Step 2b : Run the below command in the terminal 1
# Sending a Ctrl-C to stop the server
Step 2c : Run the below command to start the server
mvn spring-boot:run
Step 2d : Run the below command to see the expected output (i.e, Home)
curl 127.0.0.1:8080/
Expected Output :
Step 3 : Create a parameterized response
Step 3a : Modify DemoApplication.java to add a response for the /hello endpoint
Open demo/src/main/java/com/example/demo/DemoApplication.java
@RequestMapping("/hello") String hello() { return "Hello World\n"; }
Step 3 b : Run the below command to restart the terminal 1
Step 3c : Run the below command to see the hello endpoint output
curl 127.0.0.1:8080/hello
Expected Output :
Step 3d : We can customize the end point by adding a parameter. We'll pass the parameter into our handler method using the @RequestParam annotation, which also allows us to set a default value.
`package com.example.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.; import org.springframework.web.bind.annotation.;
@RestController @EnableAutoConfiguration public class DemoApplication {
public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); }
@RequestMapping("/hello") String hello(@RequestParam(value = "name", defaultValue = "World") String name) { return String.format("Hello %s!\n", name); }
@RequestMapping("/") String home() { return "Home\n"; } } `
Step 3e : Back in terminal 1, restart the server.
# Sending a Ctrl-C and restarting the server mvn spring-boot:run
Step 3f : In terminal 2, first try the endpoint with no parameter to see the default behavior (Hello World!). Run the below command
curl 127.0.0.1:8080/hello
Expected Output:
Step 3g: Now, try it with a parameter to see a greeting for Alex (Hello Alex!).
curl 127.0.0.1:8080/hello?name=Alex
Expected Output:
Step 4 : Access Cassandra from Java
Step 4a : To put things in context, let's see how we can access a Cassandra cluster using the Java driver.
Let's start by creating a user_credentials table. We've already installed and started Cassandra for you, so just execute the following to create the keyspace and table.
export PATH="/root/apache-cassandra-4.0-beta2/bin:$PATH" cqlsh -e " CREATE KEYSPACE user_management WITH REPLICATION = { 'class' : 'SimpleStrategy', 'replication_factor' : 1 }; CREATE TABLE user_management.user_credentials( username text, password text, PRIMARY KEY(username));"
Step 4b : We can add a handful of records to the table.
cqlsh -e " INSERT INTO user_management.user_credentials (username, password) VALUES ('User1', 'YouWontGuessThis'); INSERT INTO user_management.user_credentials (username, password) VALUES ('User2', 'SuperSecret'); INSERT INTO user_management.user_credentials (username, password) VALUES ('User3', 'UncrackablePassword');"
Step 4c : Next, let's create a Java program to access the table. We'll start by setting up a Maven project
mvn archetype:generate -DgroupId=com.datastax -DartifactId=driver-example -Dversion=1.0-SNAPSHOT -DinteractiveMode=false
Step 4d : Open App.java
Open driver-example/src/main/java/com/datastax/App.java
Step 4e : Maven projects use a pom.xml file to keep track of dependencies, etc. Inspect the pom.xml for this project
Open driver-example/pom.xml
`