I'm using CodeIgniter for my project, and I put this code under Controller as a function like this :
`
public function barcode($barcode_data)
{
// print_r($this->input->get());
/*
Author David S. Tufts
Company davidscotttufts.com
Date: 05/25/2003
Usage:
*/
// For demonstration purposes, get pararameters that are passed in through $_GET or set to the default value
$filepath = (!empty($barcode_data["filepath"])?$barcode_data["filepath"]:"");
$text = (!empty($barcode_data["text"])?$barcode_data["text"]:"0");
$size = (!empty($barcode_data["size"])?$barcode_data["size"]:"20");
$orientation = (!empty($barcode_data["orientation"])?$barcode_data["orientation"]:"horizontal");
$code_type = (!empty($barcode_data["codetype"])?$barcode_data["codetype"]:"code128");
$print = (!empty($barcode_data["print"])&&$barcode_data["print"]=='true'?true:false);
$sizefactor = (!empty($barcode_data["sizefactor"])?$barcode_data["sizefactor"]:"1");
// This function call can be copied into your project and can be made from anywhere in your code
$this->barcode_generator( $filepath, $text, $size, $orientation, $code_type, $print, $sizefactor );
}
public function barcode_generator( $filepath="", $text="0", $size="20", $orientation="horizontal", $code_type="code128", $print=false, $SizeFactor=1 )
{
$code_string = "";
// Translate the $text into barcode the correct $code_type
if ( in_array(strtolower($code_type), array("code128", "code128b")) ) {
$chksum = 104;
// Must not change order of array elements as the checksum depends on the array's key to validate final code
$codearray = array(" "=>"212222","!"=>"222122","\""=>"222221","#"=>"121223","$"=>"121322","%"=>"131222","&"=>"122213","'"=>"122312","("=>"132212",")"=>"221213","*"=>"221312","+"=>"231212",","=>"112232","-"=>"122132","."=>"122231","/"=>"113222","0"=>"123122","1"=>"123221","2"=>"223211","3"=>"221132","4"=>"221231","5"=>"213212","6"=>"223112","7"=>"312131","8"=>"311222","9"=>"321122",":"=>"321221",";"=>"312212",""=>"322112","="=>"322211",">"=>"212123","?"=>"212321","@"="232121","A"=>"111323","B"=>"131123","C"=>"131321","D"=>"112313","E"=>"132113","F"=>"132311","G"=>"211313","H"=>"231113","I"=>"231311","J"=>"112133","K"=>"112331","L"=>"132131","M"=>"113123","N"=>"113321","O"=>"133121","P"=>"313121","Q"=>"211331","R"=>"231131","S"=>"213113","T"=>"213311","U"=>"213131","V"=>"311123","W"=>"311321","X"=>"331121","Y"=>"312113","Z"=>"312311","["=>"332111","\"=>"314111","]"=>"221411","^"=>"431111",""=>"111224","`"=>"111422","a"=>"121124","b"=>"121421","c"=>"141122","d"=>"141221","e"=>"112214","f"=>"112412","g"=>"122114","h"=>"122411","i"=>"142112","j"=>"142211","k"=>"241211","l"=>"221114","m"=>"413111","n"=>"241112","o"=>"134111","p"=>"111242","q"=>"121142","r"=>"121241","s"=>"114212","t"=>"124112","u"=>"124211","v"=>"411212","w"=>"421112","x"=>"421211","y"=>"212141","z"=>"214121","{"=>"412121","|"=>"111143","}"=>"111341","~"=>"131141","DEL"=>"114113","FNC 3"=>"114311","FNC 2"=>"411113","SHIFT"=>"411311","CODE C"=>"113141","FNC 4"=>"114131","CODE A"=>"311141","FNC 1"=>"411131","Start A"=>"211412","Start B"=>"211214","Start C"=>"211232","Stop"=>"2331112");
$code_keys = array_keys($code_array);
$code_values = array_flip($code_keys);
for ( $X = 1; $X <= strlen($text); $X++ ) {
$activeKey = substr( $text, ($X-1), 1);
$code_string .= $code_array[$activeKey];
$chksum=($chksum + ($code_values[$activeKey] $X));
}
$code_string .= $code_array[$code_keys[($chksum - (intval($chksum / 103) 103))]];
I'm using CodeIgniter for my project, and I put this code under Controller as a function like this :
` public function barcode($barcode_data) { // print_r($this->input->get()); /*
Usage:
*/
// For demonstration purposes, get pararameters that are passed in through $_GET or set to the default value
$filepath = (!empty($barcode_data["filepath"])?$barcode_data["filepath"]:"");
$text = (!empty($barcode_data["text"])?$barcode_data["text"]:"0");
$size = (!empty($barcode_data["size"])?$barcode_data["size"]:"20");
$orientation = (!empty($barcode_data["orientation"])?$barcode_data["orientation"]:"horizontal");
$code_type = (!empty($barcode_data["codetype"])?$barcode_data["codetype"]:"code128");
$print = (!empty($barcode_data["print"])&&$barcode_data["print"]=='true'?true:false);
$sizefactor = (!empty($barcode_data["sizefactor"])?$barcode_data["sizefactor"]:"1");
}
public function barcode_generator( $filepath="", $text="0", $size="20", $orientation="horizontal", $code_type="code128", $print=false, $SizeFactor=1 ) { $code_string = ""; // Translate the $text into barcode the correct $code_type if ( in_array(strtolower($code_type), array("code128", "code128b")) ) { $chksum = 104; // Must not change order of array elements as the checksum depends on the array's key to validate final code $codearray = array(" "=>"212222","!"=>"222122","\""=>"222221","#"=>"121223","$"=>"121322","%"=>"131222","&"=>"122213","'"=>"122312","("=>"132212",")"=>"221213","*"=>"221312","+"=>"231212",","=>"112232","-"=>"122132","."=>"122231","/"=>"113222","0"=>"123122","1"=>"123221","2"=>"223211","3"=>"221132","4"=>"221231","5"=>"213212","6"=>"223112","7"=>"312131","8"=>"311222","9"=>"321122",":"=>"321221",";"=>"312212",""=>"322112","="=>"322211",">"=>"212123","?"=>"212321","@"="232121","A"=>"111323","B"=>"131123","C"=>"131321","D"=>"112313","E"=>"132113","F"=>"132311","G"=>"211313","H"=>"231113","I"=>"231311","J"=>"112133","K"=>"112331","L"=>"132131","M"=>"113123","N"=>"113321","O"=>"133121","P"=>"313121","Q"=>"211331","R"=>"231131","S"=>"213113","T"=>"213311","U"=>"213131","V"=>"311123","W"=>"311321","X"=>"331121","Y"=>"312113","Z"=>"312311","["=>"332111","\"=>"314111","]"=>"221411","^"=>"431111",""=>"111224","`"=>"111422","a"=>"121124","b"=>"121421","c"=>"141122","d"=>"141221","e"=>"112214","f"=>"112412","g"=>"122114","h"=>"122411","i"=>"142112","j"=>"142211","k"=>"241211","l"=>"221114","m"=>"413111","n"=>"241112","o"=>"134111","p"=>"111242","q"=>"121142","r"=>"121241","s"=>"114212","t"=>"124112","u"=>"124211","v"=>"411212","w"=>"421112","x"=>"421211","y"=>"212141","z"=>"214121","{"=>"412121","|"=>"111143","}"=>"111341","~"=>"131141","DEL"=>"114113","FNC 3"=>"114311","FNC 2"=>"411113","SHIFT"=>"411311","CODE C"=>"113141","FNC 4"=>"114131","CODE A"=>"311141","FNC 1"=>"411131","Start A"=>"211412","Start B"=>"211214","Start C"=>"211232","Stop"=>"2331112"); $code_keys = array_keys($code_array); $code_values = array_flip($code_keys); for ( $X = 1; $X <= strlen($text); $X++ ) { $activeKey = substr( $text, ($X-1), 1); $code_string .= $code_array[$activeKey]; $chksum=($chksum + ($code_values[$activeKey] $X)); } $code_string .= $code_array[$code_keys[($chksum - (intval($chksum / 103) 103))]];
} `
and I call the function like this :
$barcode_data = [ 'filepath' => null, 'text' => '1234567', 'size' => 60, 'orientation' => null, 'code_type' => 'code128', 'print' => true, 'sizefactor' => null ]; $barcode = $this->barcode($barcode_data);
and used it in here ` <!DOCTYPE html>
Please Save This Code
`
why is it showing weird string like this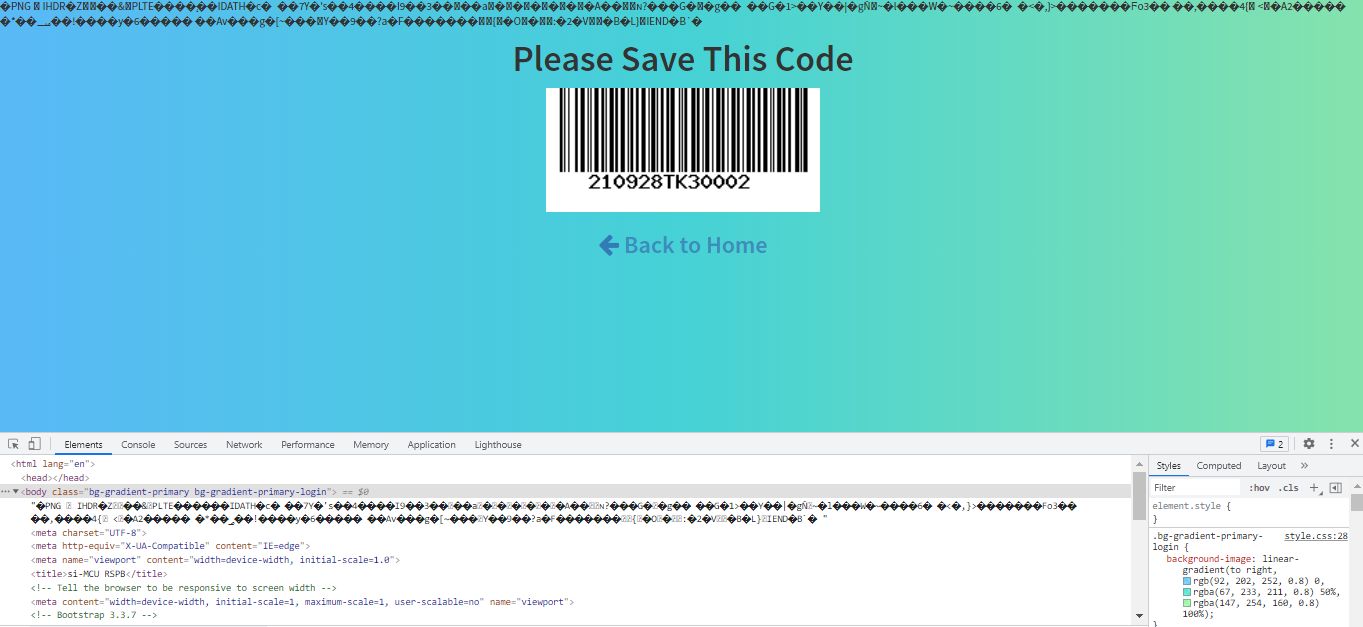