Closed eromoe closed 4 years ago
Read this article by this you can understand all the details of implement detail of BayesianLinearRegression: https://towardsdatascience.com/bayesian-linear-regression-in-python-using-machine-learning-to-predict-student-grades-part-2-b72059a8ac7e
As a general rule, I'd like to reserve Github issues for identifying errors in the codebase / making feature requests. I'm not able to provide a detailed explanation of the logic here, but you may find the following page helpful: https://www.statlect.com/fundamentals-of-statistics/Bayesian-regression
I am learning the implement of BayesianLinearRegression through numpy-ml project
I copy the code here
latex doesn't display well in github , so I pasted a picture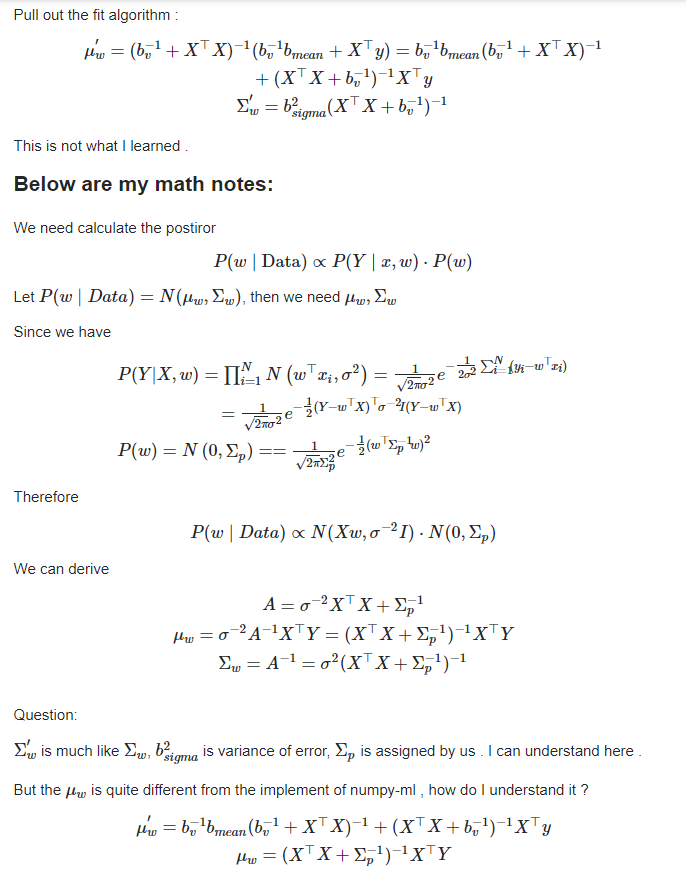
Also can look to this https://stats.stackexchange.com/questions/477618/understand-the-implement-detail-of-bayesianlinearregression-in-python