Open saiakk opened 1 year ago
If you use our built-in yolov5s model, it generate correct output:
Image img = ImageFactory.getInstance().fromUrl("https://github.com/ultralytics/yolov5/raw/master/data/images/bus.jpg");
Criteria<Image, DetectedObjects> criteria =
Criteria.builder()
.setTypes(Image.class, DetectedObjects.class)
.optModelUrls("djl://ai.djl.pytorch/yolov5s")
.optArgument("threshold", "0.8")
.optEngine("PyTorch")
.optProgress(new ProgressBar())
.build();
If you use our built-in yolov5s model, it generate correct output:
Image img = ImageFactory.getInstance().fromUrl("https://github.com/ultralytics/yolov5/raw/master/data/images/bus.jpg"); Criteria<Image, DetectedObjects> criteria = Criteria.builder() .setTypes(Image.class, DetectedObjects.class) .optModelUrls("djl://ai.djl.pytorch/yolov5s") .optArgument("threshold", "0.8") .optEngine("PyTorch") .optProgress(new ProgressBar()) .build();
What do you mean by correct output. I am also checking the values of the output. And what about using an external library for pre-processing?
I also tested your model with the following code, it find all three person:
Criteria<Image, DetectedObjects> criteria =
Criteria.builder()
.setTypes(Image.class, DetectedObjects.class)
.optModelPath(Paths.get("best.pt"))
.optEngine("PyTorch")
.optArgument("width", "640")
.optArgument("height", "640")
.optArgument("resize", "true")
.optArgument("rescale", "true")
.optArgument("optApplyRatio", "true")
.optArgument("threshold", "0.8")
.optTranslatorFactory(new YoloV5TranslatorFactory())
.build();
The image re-size algorithm has to match when the model was trained. If you want to use opencv for high performance, you can consider use our opencv extension: https://github.com/deepjavalibrary/djl/tree/master/extensions/opencv
Description
The output of YOLOv5 does not match/inaccurate if image is pre-processed using Python OpenCV instead of DJL.
Pre-processing
Inference
The output of YOLOv5 in DJL does not match with PyTorch output, though the result is accurate. DJL
PyTorch
Expected Behavior
All 3 bounding boxes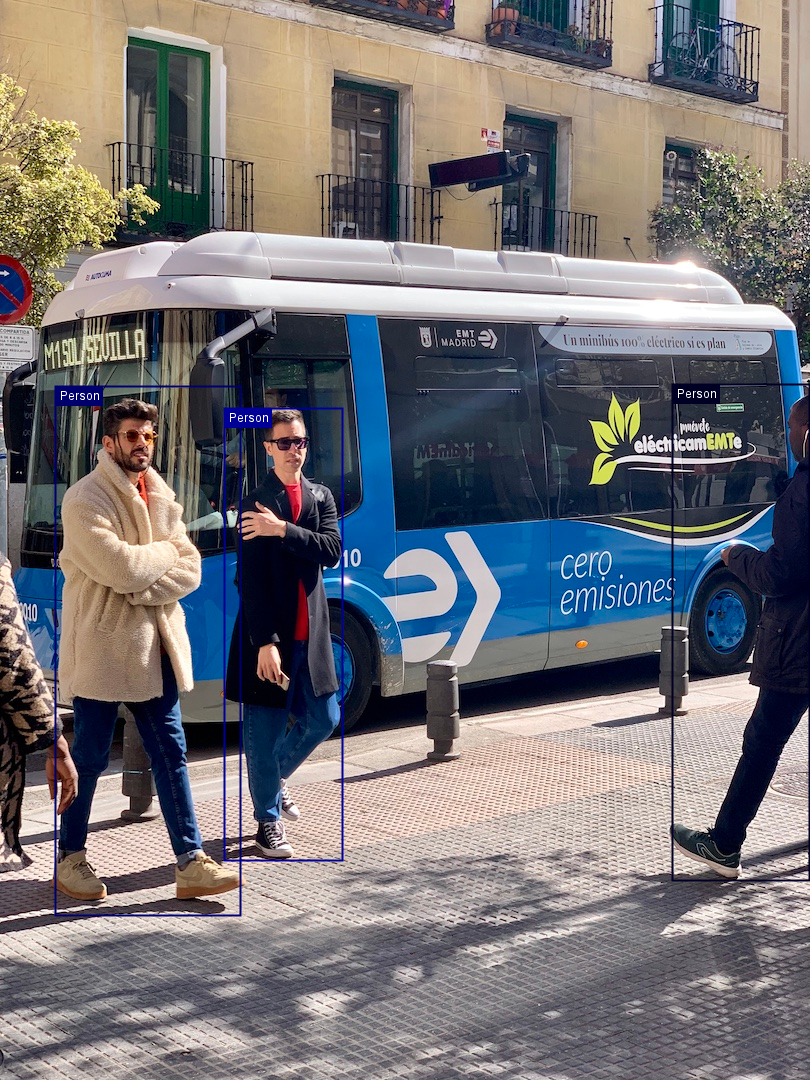
Both PyTorch and DJL output should match in ideal case.
Environment Info
Windows x86, OpenJDK, IJava,
ai.djl:api:0.21.0 ai.djl.pytorch:pytorch-jni:1.13.1-0.21.0 ai.djl.pytorch:pytorch-engine:0.21.0 ai.djl.pytorch:pytorch-model-zoo:0.21.0 ai.djl.pytorch:pytorch-native-cpu:1.13.1
Steps to reproduce
model
1563