Closed ghost closed 3 years ago
Week 3 Step 7 ⬤⬤⬤⬤⬤⬤⬤◯◯ | 🕐 Estimated completion: 20-30 minutes
Store a message from Twilio in CosmosDB and return the most recent message
deepsecrets/index.js
to the deepsecrets
branch❗ Make sure to return your message in this format:
Thanks 😊! Stored your secret "insert_user's_message". 😯 Someone confessed that: "the_most_recent_message"
To test your work, send your Twilio number a text message. Once you do so, if you navigate to your Cosmos database account, go to Data Explorer, and the dropdowns underneath your new SecretStorer
database, you should see an item that contains a message with your text message!
Important Note: If you are triggering the function for the first time, you may see an error message like the one below:
Don't worry! Try sending another text message, and everything should work fine the second time around. This error occurs because you don't have anything stored in CosmosDB yet.
💡 Yay! This means you've successfully connected Twilio with your Azure Cosmos database and Function.
Before we do anything else, we'll want to create our Cosmos DB account.
Now that your Azure Cosmos DB account is created, we should install the npm
package @azure/cosmos
.
First, we need to instantiate a variable CosmosClient
from the @azure/cosmos
package we just downloaded.
const CosmosClient = require("@azure/cosmos").CosmosClient;
Create a config object that contains all of the sensitive information that we need to manipulate our data.
Call it config
, and place keys for endpoint
, key
, databaseId
, containerId
, and partitionKey
.
create
FunctionNow, we want to write an asynchronous create
function that takes in the parameter of client
(this will be our CosmosClient
).
This function will:
:bulb: Hint: The
config.[value]
variables are accessed from theconfig
object you created earlier.
createDocument
FunctionNext, we need to create another asynchronous function called createDocument
that will take in a parameter of newItem
.
This function will create a new document within the database container that contains the newItem data.
In its entirety, the function will:
Finally, it should return your items
object from the function which contains the most recent message.
Instead of directly passing queryObject.Body
(the SMS message) to context.res
:
message
.createDocument
function you just coded to get your items
object.Your response message can then look something like:
const responseMessage = `Thanks 😊! Stored your secret "${message}". 😯 Someone confessed that: ${JSON.stringify(items[0].message)}`
That's it for Week 3, move on to Week 4 in your new issue!
Week 3 Step 6 ⬤⬤⬤⬤⬤⬤◯◯◯ | 🕐 Estimated completion: 10-20 minutes
Can you keep a secret?
This week, you will be going through steps to set up a Twilio account and create an Azure function that texts your Twilio number.
✅ Task:
DEEPSECRETS_ENDPOINT
deepsecrets/index.js
to thedeepsecrets
branchdeepsecrets
tomain
, but do not merge it🚧 Test Your Work
To test your work, try texting a message to your Twilio number - you should receive a text message back that repeats your own message.
:question: How do I find my Twilio number?
See your [phone numbers](https://www.twilio.com/console/phone-numbers/incoming).❗ Example output?
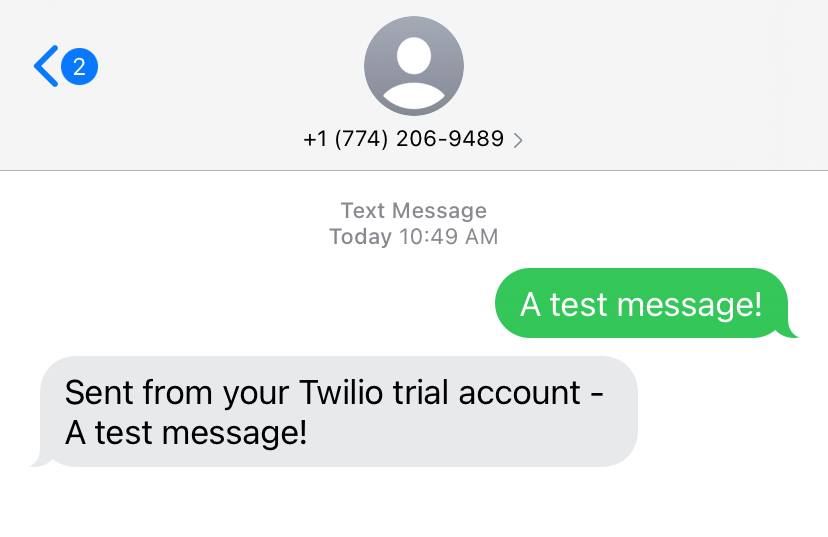 > Note: You might not get a text back on your phone because Twilio sometimes won't allow you to return a single variable as the entire body, but as long as the code passes the test, you will be okay!Set Up a Twilio Account
Since you should already have a trial Twilio Account from Week 2, you will only need to reconfigure the webhook URL. You will use the same phone number as before.
1. Create an Azure Function
Next, we'll want to create an Azure Function that will eventually output the content of texts that your Twilio number receives. This will just be a simple HTTP trigger function.
Finally, we need to install the
npm
packagequerystring
to use in our function code later.2. Configure Your Webhook URL
When someone sends a text message to your Twilio number, Twillio can call a webhook: your Azure Function. You can send a reply back simply by returning your message in the request body.
:question: Webhook? Twilio? I'm lost!
Fear not! [Webhooks](https://www.twilio.com/docs/usage/webhooks) are essentially just HTTP callbacks that are triggered by an event - in our case, this event is receiving an SMS message. When that event occurs, Twilio makes an HTTP request to the URL configured for the webhook.We'll configure the Webhook URL by filling in the Azure Function URL as a webhook.
:question: How do I find my Azure Function URL?
Navigate to your Function page (Overview), and click `Get Function URL`. 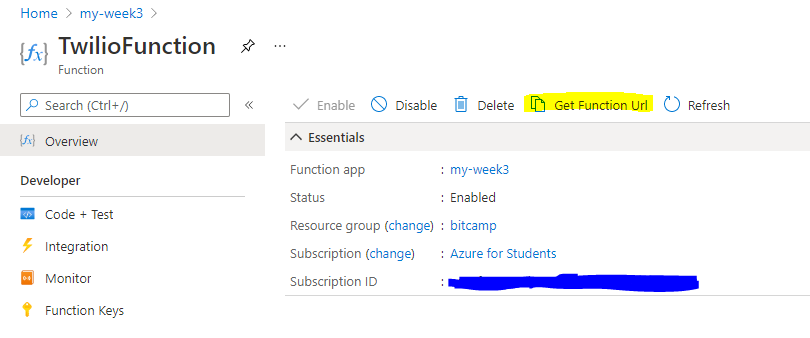:question: How do I configure my Twilio webhook URL?
1. Go to the [Twilio Console's Numbers page](https://www.twilio.com/console/phone-numbers/incoming) 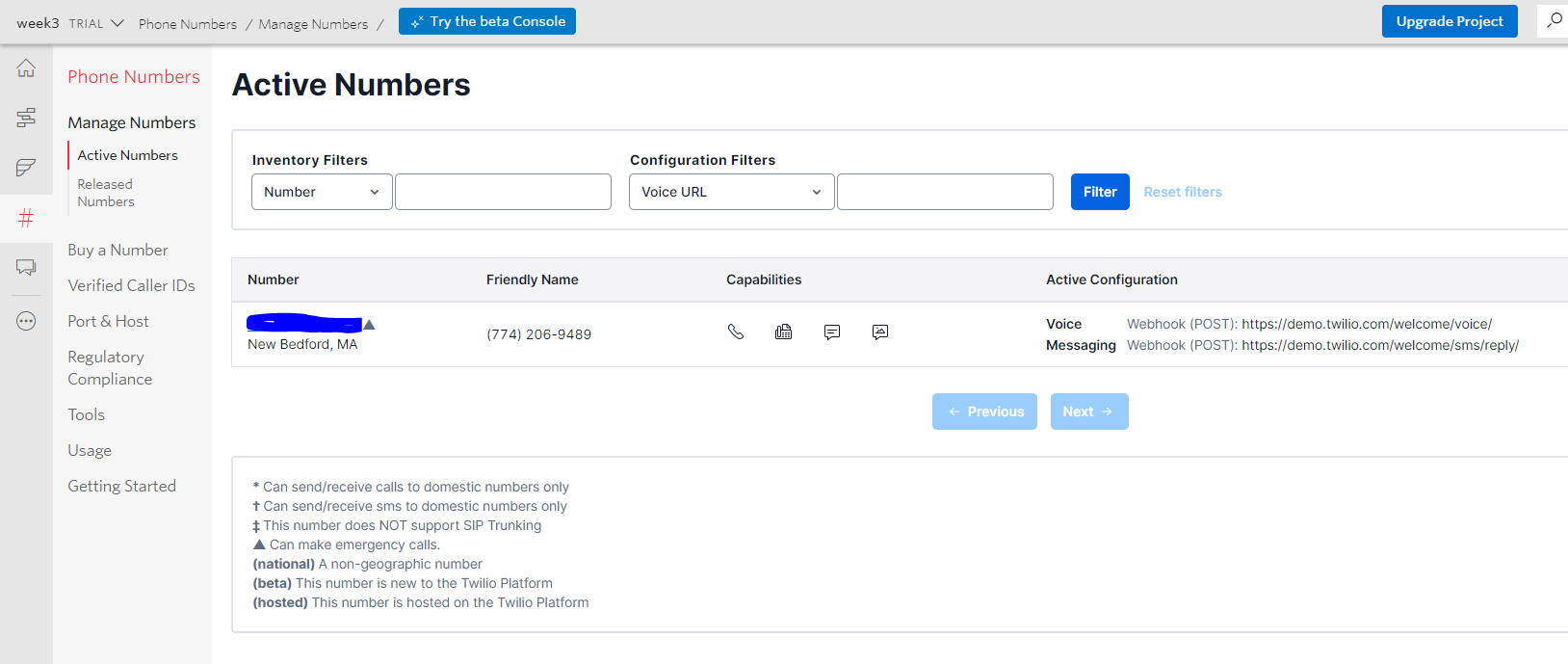 2. Click on the phone number you'd like to modify 3. Scroll down to the Messaging section and the "A MESSAGE COMES IN" option. 4. Paste in your Azure Function URL. Make sure to click `Save` afterwards!! 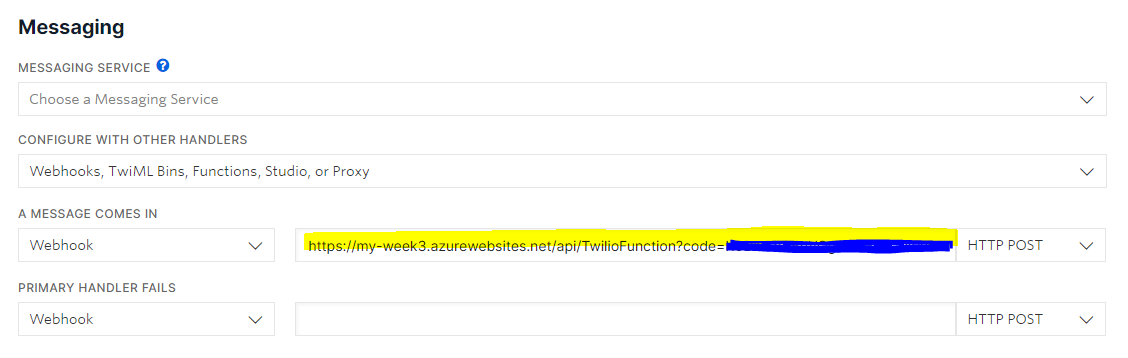3. Write your Azure Function
Now, we'll want to write our Azure Function. Go to the code for the function you created previously in this step.
First, we'll need to instantiate our
querystring
npm package in order to use it.:question: How do I use the `querystring` package?
```js const querystring = require('querystring'); ```Next, we'll use the
querystring
object to parse our request's body.:question: How do I parse the request body?
```js const queryObject = querystring.parse(req.body); ```From this output, we'll find its
Body
attribute and return it.:question: How do I return the output?
```js context.res = { body: queryObject.Body }; ``` > Since we are returning `queryObject.Body` as the entire body, you might not receive a text back on your phone. This is Twilio's issue, but the counselor bot's test should pass fine if your code is correct still!