Open barrownicholas opened 1 year ago
Hi, the error can be reproduced when passing the "raw" base64 string to the imagToPDF-Function.
What you need instead is a data URL (https://developer.mozilla.org/en-US/docs/Web/HTTP/Basics_of_HTTP/Data_URLs).
If you want to convert PNGs for example, the data URL would be: data:image/png;bas64,yourbase64here
.
So you have to prepend your base64 with the fitting data:...
-prefix.
I guess the underlying pdfkit-package does not realize that the passed data is base64 without the data:...
-prefix and interprets it as a filename and hence the ENAMETOOLONG error.
Hello, when I attempt to pass a list of base-64 URLs into the plugin, it fails and outputs
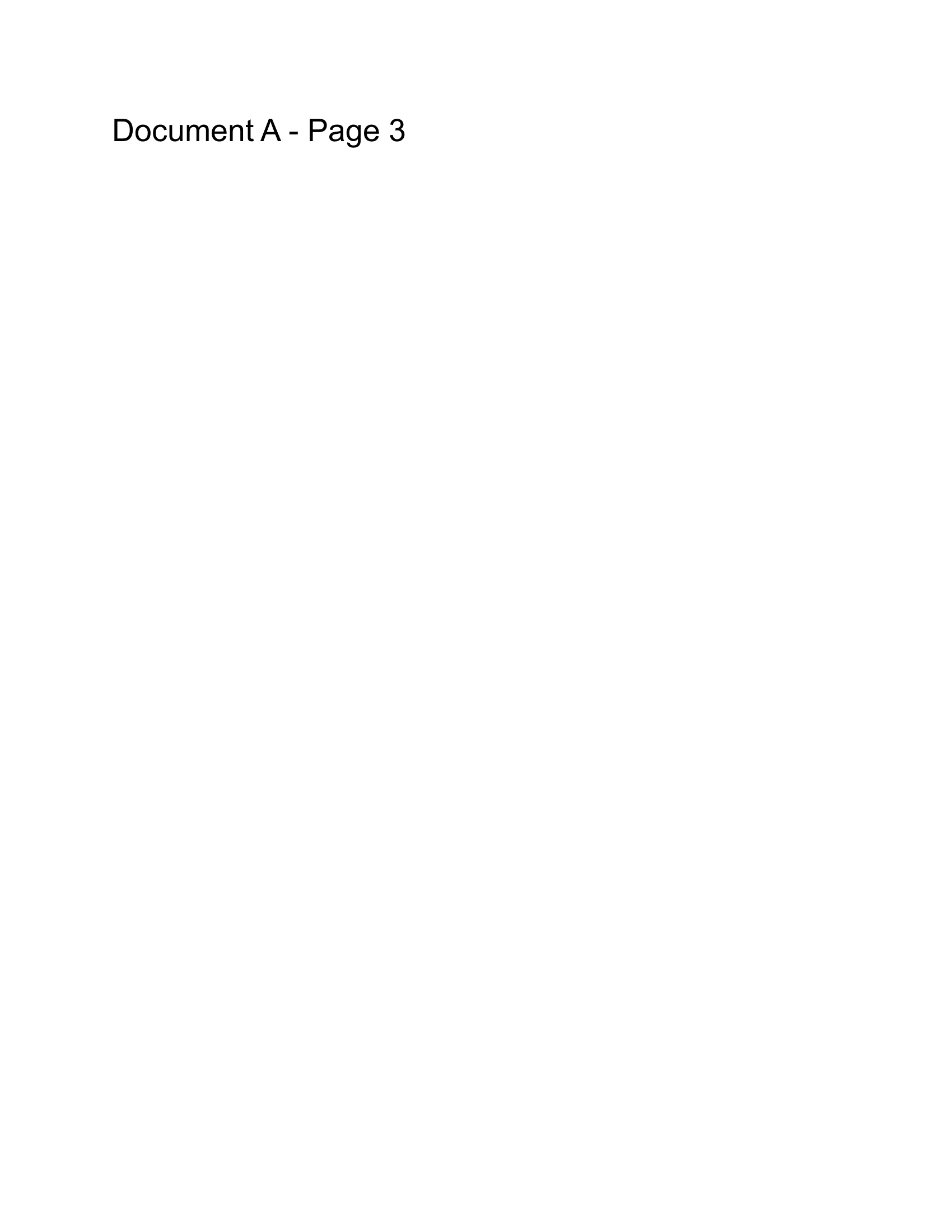
ENAMETOOLONG: name too long, open '...'
where the ... appears to be the base 64 of the item in the first position of the list. I'm attaching the files I attempted to use.