Closed jared711 closed 7 years ago
Here's the code I'm using
import usbiss import opc import argparse import sys import os import time import csv import datetime import serial
try:
spi = usbiss.USBISS("/dev/ttyACM0", 'spi', spi_mode = 2, freq = 500000)
alpha = opc.OPCN2(spi)
except Exception as e: print ("Startup Error: {}".format(e)) sys.exit(1)
alpha.on()
time.sleep(10)
#change firmware to correct version
alpha.firmware = {'major': 18, 'version': 18.2, 'minor': 2}
files = os.listdir('/home/pi/Desktop/PMdata') number=1 for i in range(0, len(files)): string = files[i] string = string[:-4] #eliminate the .csv at the end index = 0 for letter in string: if not letter.isalpha(): index = index*10 + int(letter) #make sure the tens and hundreds places are accounted for if index >= number: number = index + 1
file_name = '/home/pi/Desktop/PMdata/OPCdata%s.csv' % (number) file_csv = open(file_name,'w') csv = csv.writer(file_csv, delimiter=',')
for i in range(1,5,1):
file_number = 'file number: %s' % (number)
print (file_number)
# Read the histogram and print to console
for key, value in alpha.histogram().items():
#separated by commas
data=[i, key, value]
csv.writerows([data])
print ("i: {}\tKey: {}\tValue: {}".format(i, key, value))
""" Other values that can be read from the OPC-N2 n = alpha.sn() #Serial Number print(n) alpha.read_firmware() """
file_csv.close()
alpha.off()
Hey @jared711 great questions! If you are using a recent version of the library (I think you are) then the histogram reports numbers in number concentration (#/cc) as mentioned in the docs. If you want to get the raw histogram values, you can add the argumenent number_concentration=False
.
So, for raw values:
alpha.histogram(number_concentration=False)
For values in actual number concentration:
alpha.histogram()
Otherwise, your numbers look fine by first glance. Let me know if you have any other questions.
Hey @jared711 Did this solution work for you?
Yes, it worked great for me! Thanks for the quick response!
I've been using the OPC-N2 with a Raspberry Pi 2 Model B. I have purchased a SPI-USB converter as shown in the attached images. The silver USB cable goes to the OPC, and the other USB connections are a keyboard and mouse, and there are several connections from the pins to CO, CO2, NO, and NO2 sensors.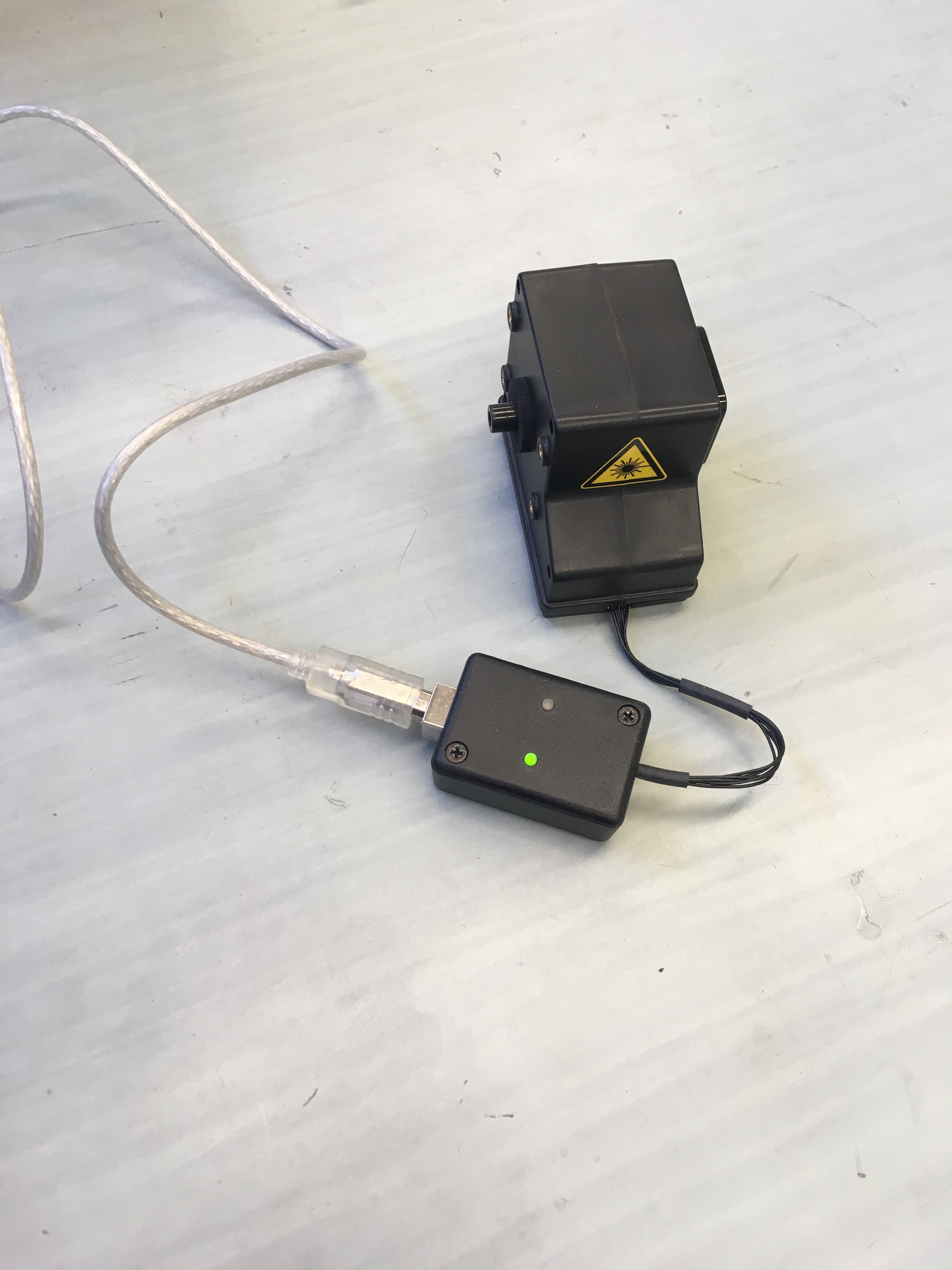
At first, I had an issue with detecting the firmware version. However, I circumvented this by writing a line of code to directly assign the firmware version to 18.2. This may be the reason for the other errors, but since I was using a commercial SPI-USB converter, I didn't think the problem could possibly be bad wiring or poor power supply. The code seemed to work and outputted a histogram, but the numbers didn't make sense. The bin values weren't even integers, as shown in the image below. I believe the problem may come from the fact that I changed the firmware version to get past an error that went a little deeper.
Do I need to get rid of that line of code and hook up the sensor differently, or can I somehow fix these histograms to show me integer values, assuming the bins are supposed to contain integer values?
I'll attach my code in another post.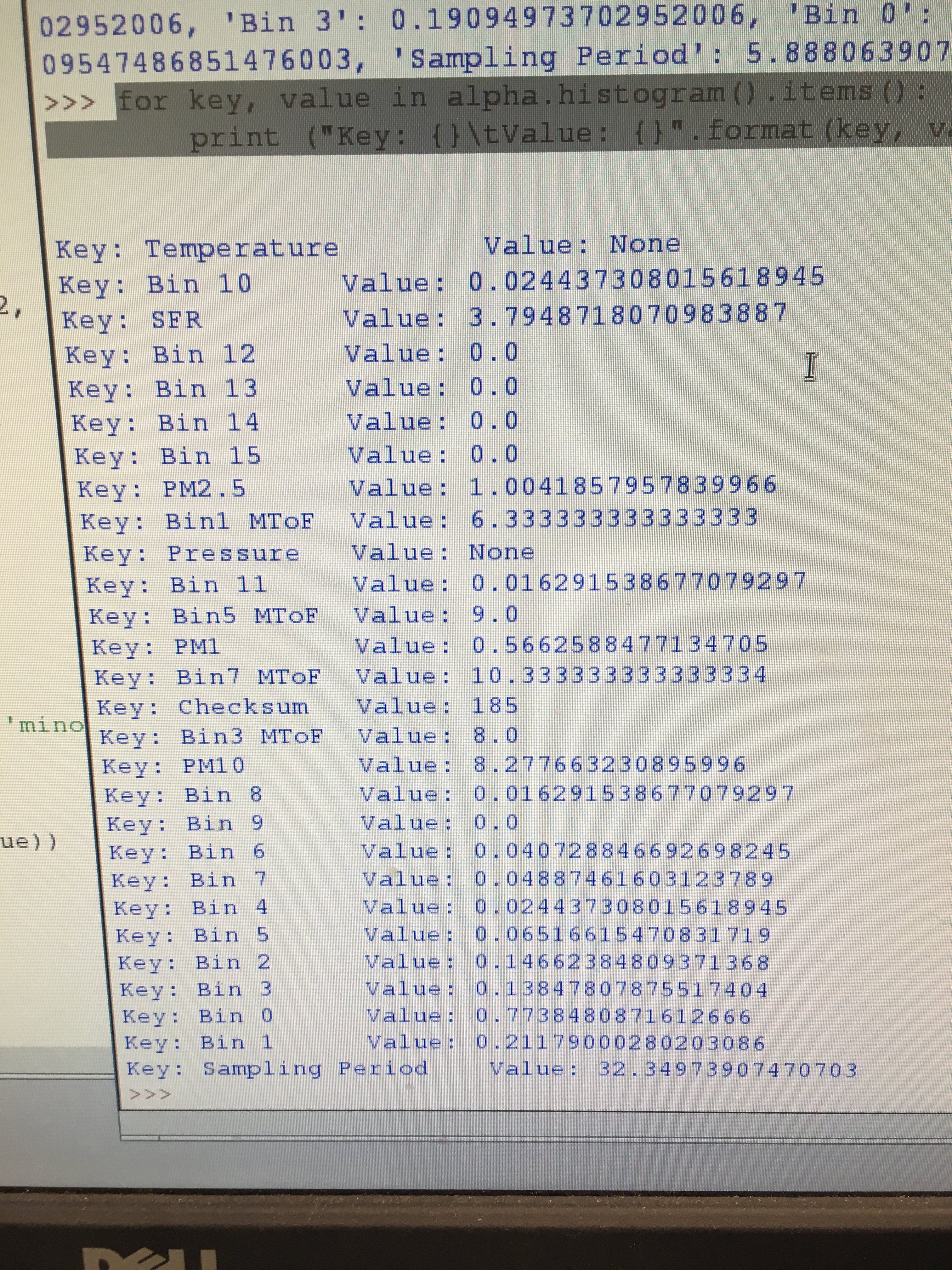