Open EliasShekhGain opened 3 years ago
Hi I am having the same issue.
When I remove the authenticatedAttributes
the SIG_CRYPTO_FAILURE
disappears but then it fails at the Is the signed qualifying property: 'message-digest' or 'SignedProperties' present?
step.
Were you able to fix the problem?
This happens because the library doesn't sort by tag/length attributes (as it should), so they end up in the wrong order which leads to invalid signature.
As a workaround, put them in the following order: content type, signing time, message digest.
@ovk, not getting your order here, why 'signing time' before 'message digest' ?
Because this manually produces the correct order (content type is the shortest one, time is longer, digest is the longest). See my reply here https://github.com/digitalbazaar/forge/issues/400#issuecomment-839296548
thanks @ovk, My question was more how attributes are compared for sorting purpose ? it is only about the size of the attribute ?
They need to be sorted by tag and size.
Here is my code:
Here is the result from https://ec.europa.eu/cefdigital/DSS/webapp-demo/validation: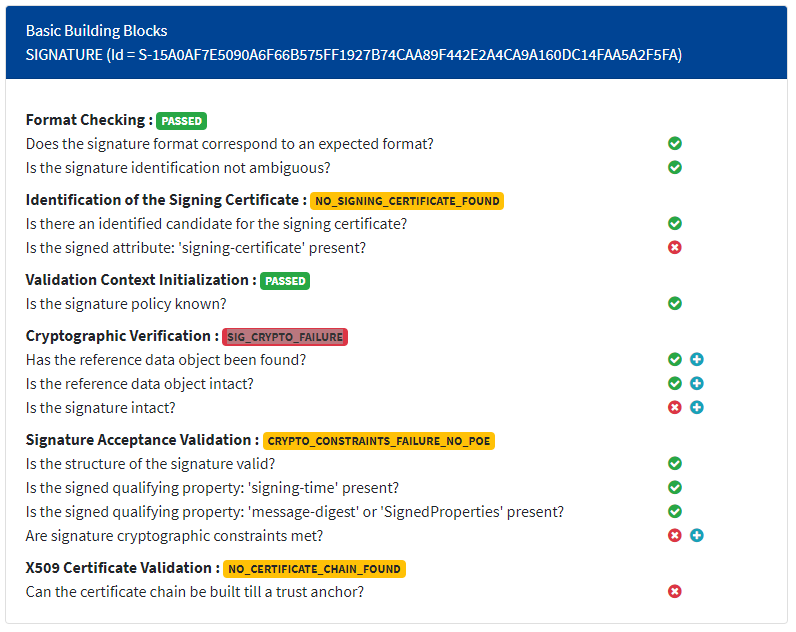
Please, help me solve this problem. Thanks in advance!!!